


When a function is overloaded, a reference or pointer is passed, and all functions must use the same type of data. When a function is rewritten, for reference member functions, the subclass function must refer to the same type of variable; for pointing to member functions, the subclass function must point to the same type of variable.
Handling of references and pointers in C function overloading and rewriting
Function overloading
Function overloading is allowed in the same role Create multiple functions within a domain with the same name but different parameter lists. For references and pointers, we need to pay attention to the following points:
-
When passing references, all functions must refer to the same type of data:
void print(const int& num); void print(const double& d);
-
When passing pointers, all functions must point to the same type of data:
void print(int* num); void print(double* d);
Function override
Function override Is to implement a function in the parent class with the same name and parameter list in the subclass. For references and pointers, we need to pay attention:
-
For reference member functions, the subclass function must refer to the same type of variable:
class Parent { public: void setAge(const int& age); }; class Child : public Parent { public: void setAge(const int& age) override; // 同一类型引用 };
-
For pointing to member functions, subclass functions must point to variables of the same type:
class Parent { public: int* getPtr(); }; class Child : public Parent { public: int* getPtr() override; // 同一类型指针 };
Practical case
Assume we have A Person
class that represents a person's age and name. There are two member functions in this class: setAge(const int& age)
and setName(const string& name)
.
We create a subclass Employee
, inherit from the Person
class, and override the setAge
function to set the age to Add 5 to it before age
.
class Person { public: void setAge(const int& age); void setName(const string& name); }; class Employee : public Person { public: void setAge(const int& age) override; // 重写setAge函数 }; // Employee 类中的 setAge 函数 void Employee::setAge(const int& age) { Person::setAge(age + 5); // 调用父类setAge函数 }
Now, we can create an Employee
object and use the overridden setAge
function:
Employee emp; emp.setAge(20); // 实际设置为 25
The above is the detailed content of Handling of references and pointers in C++ function overloading and rewriting. For more information, please follow other related articles on the PHP Chinese website!
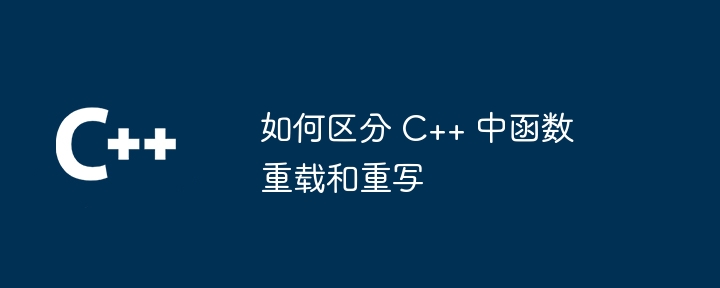
函数重载允许一个类中具有同名但签名不同的函数,而函数重写发生在派生类中,当它覆盖基类中具有相同签名的函数,提供不同的行为。
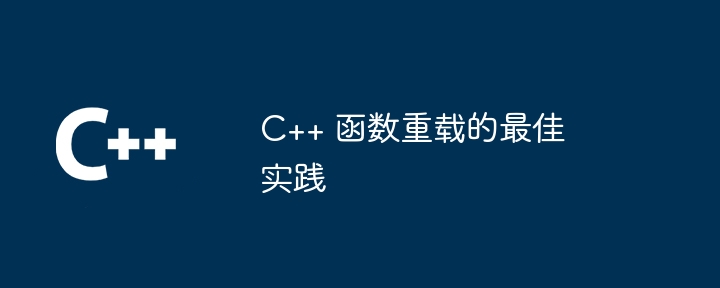
C++函数重载最佳实践:1、使用清晰且有意义的名称;2、避免过载过多;3、考虑默认参数;4、保持参数顺序一致;5、使用SFINAE。
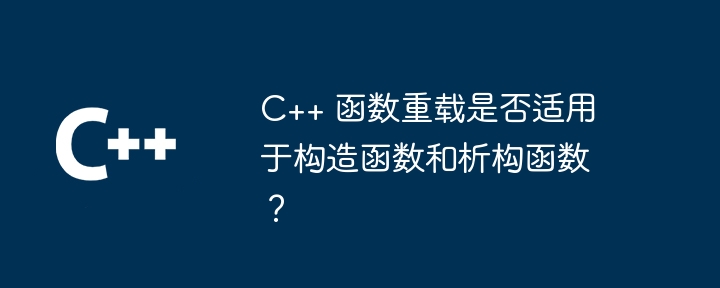
C++构造函数支持重载,而析构函数不支持。构造函数可具有不同的参数列表,而析构函数只能有一个空参数列表,因为它在销毁类实例时自动调用,不需输入参数。
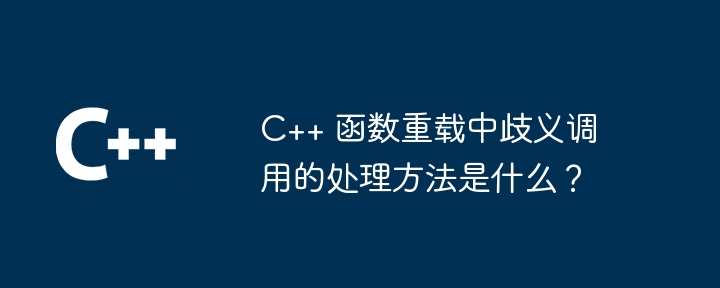
歧义调用发生在编译器无法确定调用哪个重载函数时。处理方法包括:为每个重载函数提供唯一的函数签名(参数类型和数量)。使用显式类型转换强制调用正确的函数,如果一个重载函数的参数类型更适合给定调用的参数。如果编译器无法解决歧义调用,将产生错误消息,需要重新检查函数重载并进行修改。
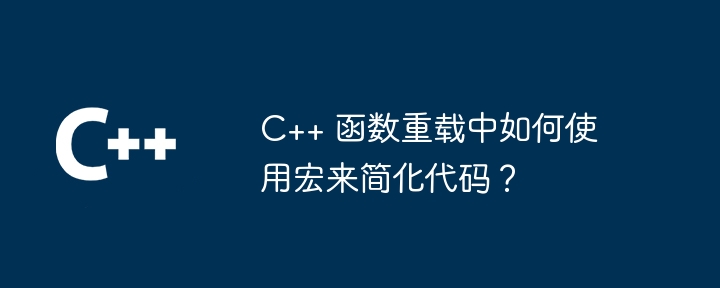
宏简化C++函数重载:创建宏,将通用代码提取到单个定义中。在每个重载函数中使用宏替换通用的代码部分。实际应用包括创建打印输入数据类型信息的函数,分别处理int、double和string数据类型。
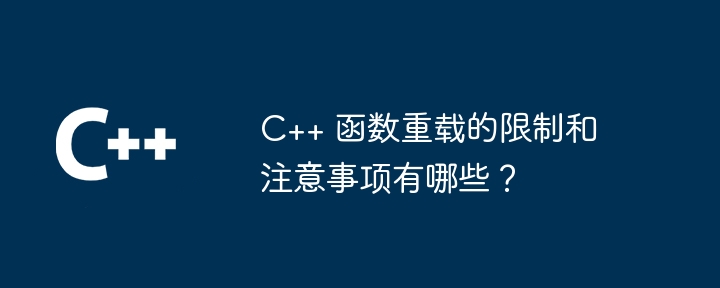
函数重载的限制包括:参数类型和顺序必须不同(相同参数个数时),不能使用默认参数区分重载。此外,模板函数和非模板函数不能重载,不同模板规范的模板函数可以重载。值得注意的是,过度使用函数重载会影响可读性和调试,编译器从最具体到最不具体的函数进行搜索以解决冲突。
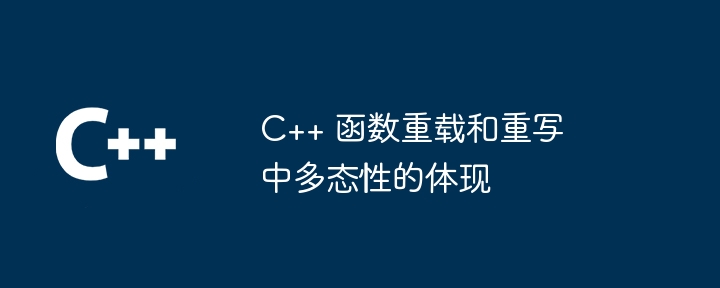
C++中的多态性:函数重载允许具有相同名称但不同参数列表的多个函数,根据调用时的参数类型选择执行的函数。函数重写允许派生类重新定义基类中已存在的方法,从而实现不同类型的行为,具体取决于对象的类型。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
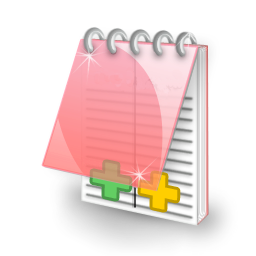
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
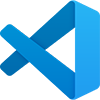
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
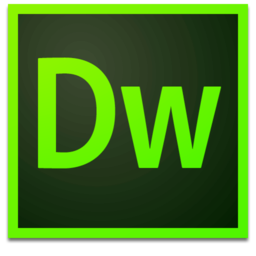
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool