Reference parameters point directly to the variables passed to the function, providing efficiency, modifiability and security. Specifically, reference parameters improve efficiency (avoiding copies), allow functions to modify variables in the caller, and eliminate the risk of dangling references. The syntax is to add & before the type name, such as void foo(int& x);. In practice, using reference parameters to pass the radius can save the cost of copying the radius value. Precautions include initializing reference parameters, not modifying the address, and still pointing to the original variable after the call.
How to use reference parameters in C functions
In C, Reference parameters are parameters of the function, which point directly to the call Variables passed to the function. This is different from value parameters, which copies and stores the passed value. Using reference parameters provides several benefits:
- Efficiency: The overhead of copying the original value is avoided, thereby improving efficiency.
- Modifiability: When passing a reference, the function can modify the value of the actual variable in the calling function.
- Safety: Avoids the risk of dangling references pointing to destroyed variables.
Syntax
To declare a reference parameter, add the symbol &
before the type name:
void foo(int& x);
Practical case
Let us consider a function that calculates pi π
. Passing the radius by reference parameter can save the overhead of copying the radius value:
#include <iostream> #include <cmath> using namespace std; void calculatePi(double& pi, double radius) { pi = 2 * acos(-1.0) * radius; } int main() { double pi; double radius = 2.5; calculatePi(pi, radius); cout << "Pi: " << pi << endl; return 0; }
In the calculatePi
function, pi
is a reference parameter, allowing the function to directly modify pi
variable. So, in the main
function, when radius
changes, pi is updated accordingly.
Notes
When using reference parameters, you need to pay attention to the following:
- Reference parameters must be initialized and point to a valid variable.
- Functions must not modify the address of a variable passed as a reference parameter.
- After the function is called, the reference still points to the original variable, so any changes to it will be reflected in the original variable.
The above is the detailed content of How to use reference parameters in C++ functions. For more information, please follow other related articles on the PHP Chinese website!
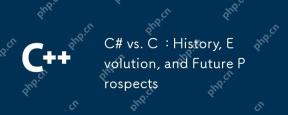
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
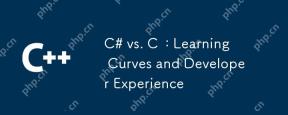
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
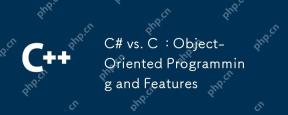
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
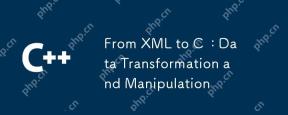
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
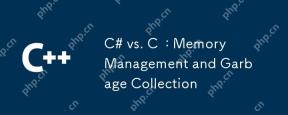
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
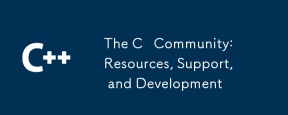
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
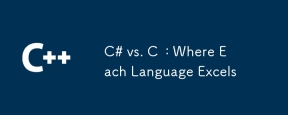
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
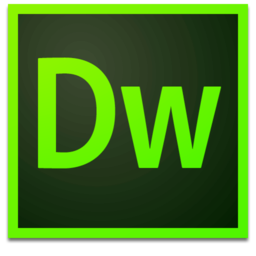
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
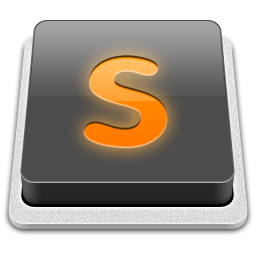
SublimeText3 Mac version
God-level code editing software (SublimeText3)
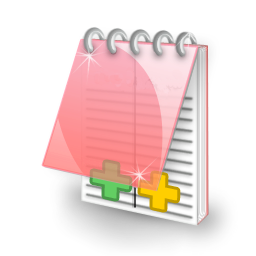
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function