


What is the difference between local variables and global variables of a C++ function?
C The difference between local variables and global variables: Visibility: Local variables are limited to the defining function, while global variables are visible throughout the program. Memory allocation: local variables are allocated on the stack, while global variables are allocated in the global data area. Scope: Local variables are within a function, while global variables are throughout the program. Initialization: Local variables are initialized when a function is called, while global variables are initialized when the program starts. Recreation: Local variables are recreated on every function call, while global variables are created only when the program starts.
Local variables and global variables of C functions: big difference
In C, there are important differences between local variables and global variables. Understand these The distinction is critical to writing efficient, maintainable code.
Local variables
- are defined within a function and are only visible within the function scope.
- Created when the function is called and destroyed when the function returns.
- Local variables will be recreated every time the function is called.
Sample code:
void myFunction() { int localVariable = 5; // 局部变量 // ... 使用 localVariable } int main() { myFunction(); // localVariable 无法访问,因为它不在 main() 函数的范围内 }
Global variables
- are defined outside the function and are visible throughout the entire program.
- Created when the program starts and destroyed when the program terminates.
- Global variables are visible to all functions in the program.
Sample code:
int globalVariable = 10; // 全局变量 void myFunction() { // ... 使用 globalVariable } int main() { // ... 使用 globalVariable }
Difference
Features | Local variables | Global variables |
---|---|---|
Visibility | Limited to the function in which they are defined | Entire program |
Life cycle | During function call | During program running |
Memory allocation | On the stack | In the global data area |
Scope | In the function | In the entire program |
Initialization | When the function is called | When the program starts |
Recreate | Every time the function When called | Only when the program starts |
Actual case
Examples of local variables
In the following example , the local variable name
is only used within the greet()
function, and is recreated each time the function is called:
void greet(std::string name) { std::cout << "Hello, " << name << "!" << std::endl; } int main() { greet("John"); greet("Mary"); // 局部变量 name 将重新创建 }
Example of global variables
In the following example, the global variable g_count
is visible program-wide and is updated every time the function is called:
int g_count = 0; // 全局变量 void incrementCount() { g_count++; } int main() { incrementCount(); std::cout << "Count: " << g_count << std::endl; // 输出 1 incrementCount(); std::cout << "Count: " << g_count << std::endl; // 输出 2 }
The above is the detailed content of What is the difference between local variables and global variables of a C++ function?. For more information, please follow other related articles on the PHP Chinese website!
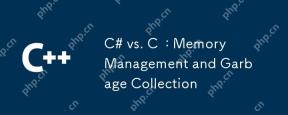
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
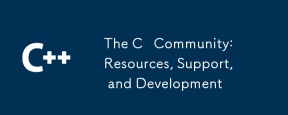
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
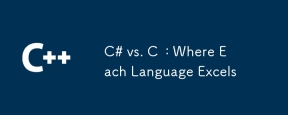
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
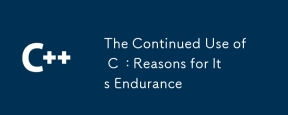
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
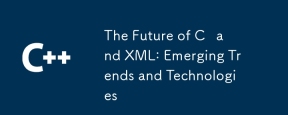
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
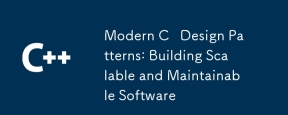
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
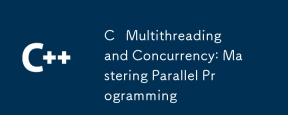
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.