In C, function parameters are exception-safe when passed by value, because the actual parameters retain their original values; passing by reference does not guarantee exception safety, and the actual parameters may be in an undefined state. Best practices recommend using pass-by-value to pass primitive types, lightweight objects, and parameters that do not affect the function call, and using pass-by-reference to pass large objects or parameters that require function modification.
C function exception safe parameter passing mechanism
In C, when calling a function, parameters can be passed by value or by Pass by reference. Passing by value means creating a copy of the actual parameter, while passing by reference uses the address of the actual parameter.
Pass by value
void foo(int x) { // 对 x 执行操作 }
Pass by reference
void foo(int& x) { // 对 x 执行操作 }
When a function throws an exception, the parameter passing mechanism has a real impact parameter status.
Pass-by-value exception safety
Pass-by-value is exception-safe because if the function throws an exception, the actual parameters will retain their original values. For example:
int main() { int x = 1; try { foo(x); } catch(...) { // x 仍为 1 } }
Pass-by-reference exception safety
Pass-by-reference does not guarantee exception safety. If a function throws an exception, the actual parameters may be in an undefined state because the function may have changed the reference. For example:
int main() { int x = 1; try { foo(x); } catch(...) { // x 可能不是 1 } }
Best Practices
To ensure exception safety, it is recommended to use pass-by-value in the following situations:
- Parameters Is a basic type (for example, int, char, double).
- The parameters are lightweight objects (for example, small structures).
- Changes to actual parameters should not affect the calling function.
Use pass-by-reference when:
- The parameter is a large object (e.g., container, complex structure).
- Changes to actual parameters need to be reflected in the calling function.
Practical Case
Consider a function that reads a stream and writes it to a file.
Pass the stream by value:
void writeToFile(std::istream& stream, std::ofstream& file) { std::string line; while (getline(stream, line)) { file << line << std::endl; } }
Pass the stream by reference:
void writeToFile(std::istream& stream, std::ofstream& file) { std::string line; while (getline(stream, line)) { file << line << std::endl; } stream.close(); // 按引用传递允许在函数退出时关闭流 }
By passing the stream by reference, we can ensure that the stream is always closed when the function exits, thus ensuring exceptions Occurs when the stream is in a closed state.
The above is the detailed content of C++ function exception safe parameter passing mechanism. For more information, please follow other related articles on the PHP Chinese website!
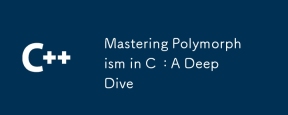
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
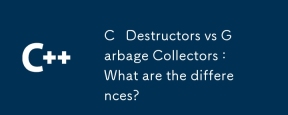
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
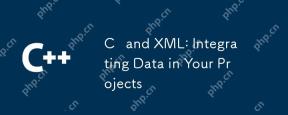
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
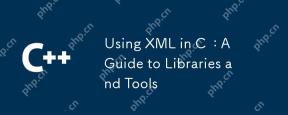
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
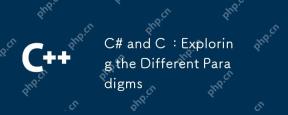
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
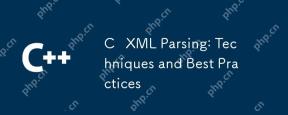
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
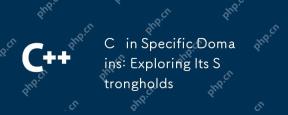
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
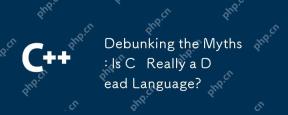
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
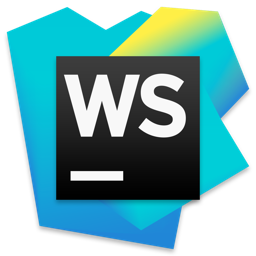
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
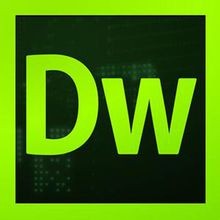
Dreamweaver CS6
Visual web development tools
