In C, memory management is the responsibility of the programmer. The function library provides many tools, including: std::make_unique and std::make_shared: create smart pointers and automatically release memory. std::vector and std::array: container classes that dynamically allocate and release memory. std::allocator: a universal container for custom memory allocation strategies.
Memory Management in C Library
Memory management is crucial in C because the programmer is responsible for allocation and deallocation Dynamically allocated memory. Function libraries provide valuable tools when managing memory, helping to increase efficiency and reduce the risk of memory leaks.
std::make_unique and std::make_shared
std::make_unique
and std::make_shared
functions are used By creating a smart pointer, ownership is automatically managed by the pointer. The new
operator only allocates memory. Smart pointers automatically release memory when an object exceeds its scope to prevent memory leaks.
// 使用 std::make_unique 分配一个 unique_ptr std::unique_ptr<int> ptr1 = std::make_unique<int>(42); // 使用 std::make_shared 分配一个 shared_ptr std::shared_ptr<int> ptr2 = std::make_shared<int>(42);
std::vector and std::array
##std::vector and
std::array are Container class that can dynamically allocate and release memory.
std::vector is a dynamically sized array, while
std::array is a fixed-size array. Both release their memory automatically, eliminating the need to manually call
delete.
// 使用 std::vector 动态分配数组 std::vector<int> vec; for (int i = 0; i < 10; i++) { vec.push_back(i); } // 使用 std::array 固定大小的数组 std::array<int, 10> arr; for (int i = 0; i < 10; i++) { arr[i] = i; }
std::allocator
std::allocator is used to allocate and release memory. It is a general-purpose container that can be used with various container classes, such as
std::vector. Use
std::allocator to specify a memory allocation strategy, such as a custom memory pool or a custom release function.
// 使用 std::allocator 创建自定义分配器 std::allocator<int> my_alloc; // 使用 my_alloc 创建 std::vector std::vector<int, std::allocator<int>> vec(my_alloc);
Practical Case
Consider a game development application where the player's position is represented by thePlayer class. This class stores the location coordinates and allocates and frees memory to update the coordinates as the player moves.
// 使用 std::make_unique 创建 Player 对象 std::unique_ptr<Player> player = std::make_unique<Player>(); // 更新玩家坐标 player->move(10, 20); // 销毁 Player 对象会自动释放内存 player.reset();Use
std::make_unique to ensure that memory is released when the
player object goes out of scope, preventing memory leaks and simplifying memory management.
The above is the detailed content of How does the C++ function library perform memory management?. For more information, please follow other related articles on the PHP Chinese website!
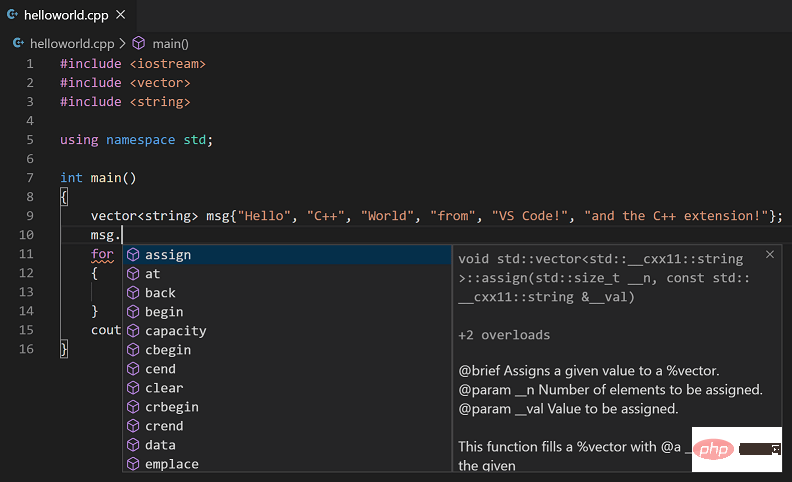
C++是一种广泛使用的面向对象的计算机编程语言,它支持您与之交互的大多数应用程序和网站。你需要编译器和集成开发环境来开发C++应用程序,既然你在这里,我猜你正在寻找一个。我们将在本文中介绍一些适用于Windows11的C++编译器的主要推荐。许多审查的编译器将主要用于C++,但也有许多通用编译器您可能想尝试。MinGW可以在Windows11上运行吗?在本文中,我们没有将MinGW作为独立编译器进行讨论,但如果讨论了某些IDE中的功能,并且是DevC++编译器的首选
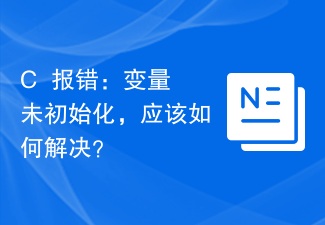
在C++程序开发中,当我们声明了一个变量但是没有对其进行初始化,就会出现“变量未初始化”的报错。这种报错经常会让人感到很困惑和无从下手,因为这种错误并不像其他常见的语法错误那样具体,也不会给出特定的代码行数或者错误类型。因此,下面我们将详细介绍变量未初始化的问题,以及如何解决这个报错。一、什么是变量未初始化错误?变量未初始化是指在程序中声明了一个变量但是没有
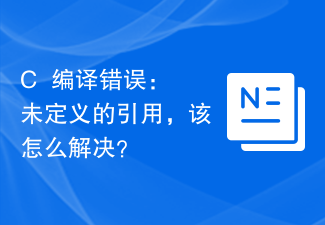
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
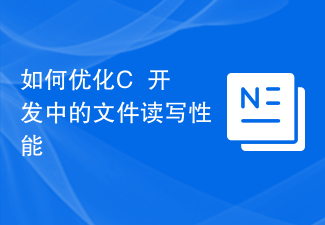
如何优化C++开发中的文件读写性能在C++开发过程中,文件的读写操作是常见的任务之一。然而,由于文件读写是磁盘IO操作,相对于内存IO操作来说会更为耗时。为了提高程序的性能,我们需要优化文件读写操作。本文将介绍一些常见的优化技巧和建议,帮助开发者在C++文件读写过程中提高性能。使用合适的文件读写方式在C++中,文件读写可以通过多种方式实现,如C风格的文件IO
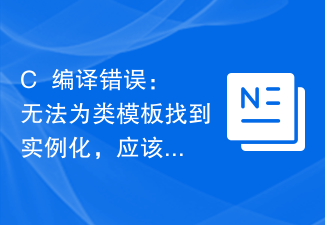
C++是一门强大的编程语言,它支持使用类模板来实现代码的复用,提高开发效率。但是在使用类模板时,可能会遭遇编译错误,其中一个比较常见的错误是“无法为类模板找到实例化”(error:cannotfindinstantiationofclasstemplate)。本文将介绍这个问题的原因以及如何解决。问题描述在使用类模板时,有时会遇到以下错误信息:e
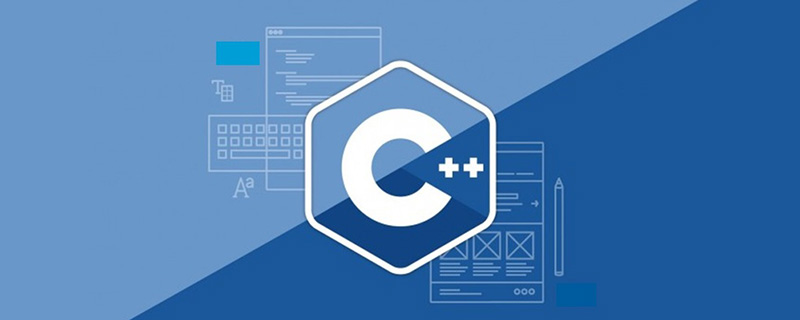
iostream头文件包含了操作输入输出流的方法,比如读取一个文件,以流的方式读取;其作用是:让初学者有一个方便的命令行输入输出试验环境。iostream的设计初衷是提供一个可扩展的类型安全的IO机制。
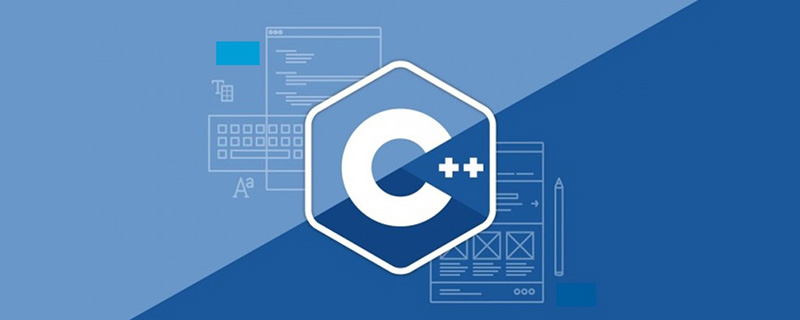
c++初始化数组的方法:1、先定义数组再给数组赋值,语法“数据类型 数组名[length];数组名[下标]=值;”;2、定义数组时初始化数组,语法“数据类型 数组名[length]=[值列表]”。
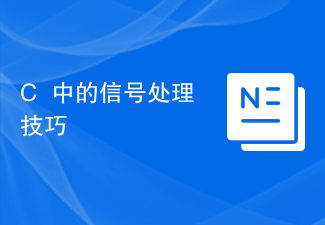
C++是一种流行的编程语言,它强大而灵活,适用于各种应用程序开发。在使用C++开发应用程序时,经常需要处理各种信号。本文将介绍C++中的信号处理技巧,以帮助开发人员更好地掌握这一方面。一、信号处理的基本概念信号是一种软件中断,用于通知应用程序内部或外部事件。当特定事件发生时,操作系统会向应用程序发送信号,应用程序可以选择忽略或响应此信号。在C++中,信号可以


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
