JUnit is a widely used Java unit testing framework in Spring projects. It can be applied through the following steps: Add JUnit dependency:
Application of JUnit unit testing framework in Spring projects
JUnit is a widely used Java unit testing framework that can help Developers test code quickly and reliably. In Spring projects, JUnit can be used to test Spring beans and service layer logic.
Configuring JUnit
To use JUnit in a Spring project, you need to add the necessary dependencies to the project:
<dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter</artifactId> <version>5.8.1</version> <scope>test</scope> </dependency>
Write test cases
Follow the following steps to write test cases using JUnit:
- Use the
@ExtendWith
annotation in the test class to enable the JUnit extension function:@ExtendWith(SpringExtension.class)
- Use the
@Autowired
annotation to inject the Bean to be tested - Use
@BeforeEach
and@AfterEach
Annotations to perform pre- and post-test preparation and cleanup work - Use
@Test
annotations to mark test methods
Practical case
The following is a simple example of testing a Spring service:
@ExtendWith(SpringExtension.class) class UserServiceTest { @Autowired private UserService userService; @Test void shouldFindUserById() { // Arrange long userId = 1L; // Act User user = userService.findById(userId); // Assert assertThat(user).isNotNull(); assertThat(user.getId()).isEqualTo(userId); assertThat(user.getName()).isEqualTo("John Doe"); } }
In this example, the @Autowired
annotation injects the UserService
Bean into In the test class, the @Test
annotation marks a test method. The test method sets up a sample user ID, calls the findById
method of UserService
, and then asserts that the returned user object is as expected.
Extended functions
JUnit 5 provides a wide range of extended functions to simplify testing, such as:
- Parameterized testing (Parameterized Tests): Allows running the same test case with different parameter sets.
- Dependency Injection: Allows for convenient injection of beans in test classes.
- Assertion Library (Assertions): Provides various assertions to make the code more readable.
Conclusion
The JUnit unit testing framework is an indispensable tool in Spring projects, allowing developers to quickly and reliably test code. By configuring JUnit dependencies, writing test cases, and leveraging extension capabilities, developers can improve code quality and ensure that Spring applications run properly.
The above is the detailed content of Application of JUnit unit testing framework in Spring projects. For more information, please follow other related articles on the PHP Chinese website!
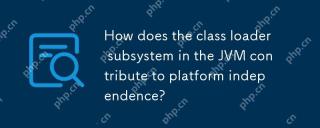
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
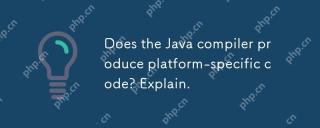
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
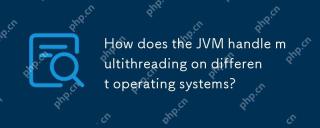
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
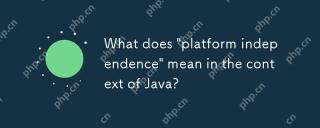
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
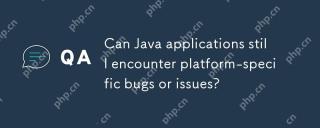
Javaapplicationscanindeedencounterplatform-specificissuesdespitetheJVM'sabstraction.Reasonsinclude:1)Nativecodeandlibraries,2)Operatingsystemdifferences,3)JVMimplementationvariations,and4)Hardwaredependencies.Tomitigatethese,developersshould:1)Conduc
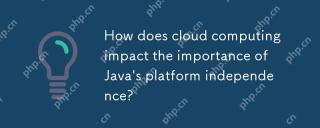
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
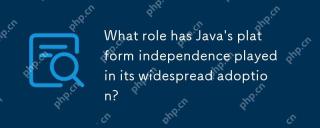
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
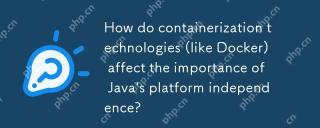
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
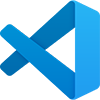
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!