C Unit testing is the process of verifying the behavior of a single function. Available frameworks include: Google Test (Googletest) Catch2Boost.Test unit testing provides advanced features such as mock objects, data-driven testing, and parameterized testing. Use cases can be used to isolate and test functions, such as those that calculate a user's account balance. Functional unit testing is a key practice for improving C code quality and simplifying maintenance.
C Functional Unit Testing Guide
Introduction
Functional unit testing is isolation and the process of validating the behavior of a single function or module without relying on other components. In C, unit testing can help you improve code quality, increase confidence in errors, and simplify code maintenance.
Framework Selection
There are many C unit testing frameworks to choose from, such as:
- Google Test (Googletest) : A popular and widely used framework that provides rich functionality.
- Catch2: A modern and lightweight framework with a clear and easy-to-use API.
- Boost.Test: Part of the Boost C library, providing a variety of unit testing tools.
HelloWorld Example
Suppose we have a function called add
that adds two numbers. Let’s write a unit test using Googletest:
#include <gtest/gtest.h> TEST(AddFunctionTest, SimpleAddition) { EXPECT_EQ(add(1, 2), 3); }
-
TEST
The macro creates a test case namedAddFunctionTest
. -
SimpleAddition
is a test method. -
EXPECT_EQ
Assert function result is3
.
Advanced features
The unit testing framework also provides various advanced features, such as:
- Mock Object: Used to simulate dependent objects.
- Data-driven testing: Run tests using different data sets.
- Parameterized testing: Run the same origin with different parameter values.
Practical Case
In the online banking system, there is a key function used to calculate the user account balance. This function reads the balance in the database and adds it to the transaction history.
Using unit testing, we can isolate and test the function. We can create the following test cases:
TEST(AccountBalanceTest, GetBalance) { Account account(123); EXPECT_EQ(account.getBalance(), 1000); } TEST(AccountBalanceTest, AddTransaction) { Account account(123); account.addTransaction(500); EXPECT_EQ(account.getBalance(), 1500); }
These tests verify that the function calculates the balance correctly and handles transactions correctly.
Conclusion
Functional unit testing is a key practice to improve the quality of your C code. It enables you to isolate and verify the behavior of individual functions, increasing confidence in errors and simplifying code maintenance. By using a unit testing framework and advanced features, you can create powerful and maintainable code.
The above is the detailed content of A guide to unit testing C++ functions. For more information, please follow other related articles on the PHP Chinese website!

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
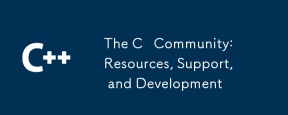
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
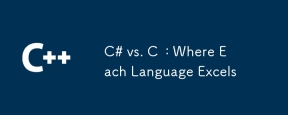
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
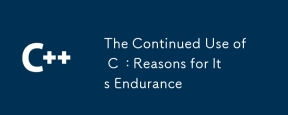
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
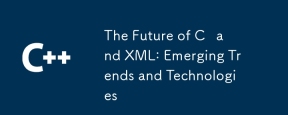
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
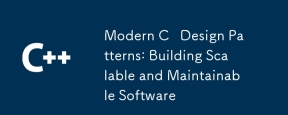
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
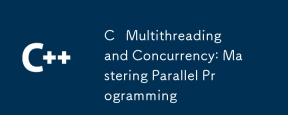
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
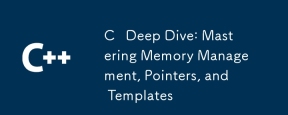
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
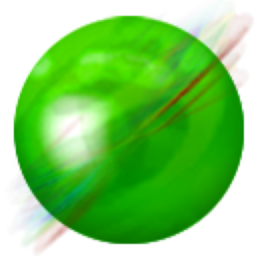
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
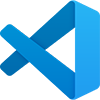
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
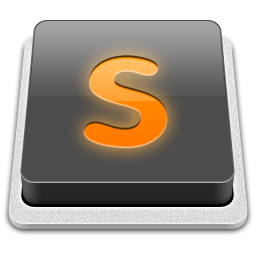
SublimeText3 Mac version
God-level code editing software (SublimeText3)
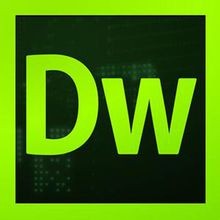
Dreamweaver CS6
Visual web development tools