


In C, lambda expressions can be used to easily filter and transform data. For example, you can use lambda expressions to filter odd elements in a container, transform elements in a container, filter and transform associative containers, use lambda expressions in algorithms, and pass lambda expressions as function arguments. These methods can make data processing tasks simpler and more efficient.
A practical case of using C lambda expressions for data filtering and transformation
Lambda expressions are very useful in C, especially It's when filtering and transforming data. Here are some examples of practical applications:
1. Filter elements in a container
std::vector<int> numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9}; // 使用 lambda 表达式过滤奇数 std::vector<int> odd_numbers = std::filter(numbers.begin(), numbers.end(), [](int n) { return n % 2 != 0; });
2. Transform elements in a container
std::vector<double> squares = std::transform(numbers.begin(), numbers.end(), [](int n) { return n * n; });
3. Filter and transform associated containers
std::map<int, std::string> name_age = { {1, "John"}, {2, "Mary"}, {3, "Bob"}, {4, "Alice"} }; // 过滤年龄大于 2 的人 auto filtered_name_age = std::map<int, std::string>(name_age.begin(), name_age.end(), [](const std::pair<int, std::string>& p) { return p.second.size() > 2; });
4. Use lambda expressions in algorithms
std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a > b; }); // 从大到小排序
5. Passing lambda expressions as function parameters
void process_data(std::vector<int>& data, std::function<void(int)> callback) { for (int& element : data) { callback(element); } } process_data(numbers, [](int n) { std::cout << n << " "; }); // 打印出容器中的每个元素
These are just some of the ways in C to use lambda expressions for data filtering and transformation. By leveraging lambda expressions, you can write concise and powerful code to handle various data manipulation tasks.
The above is the detailed content of What are some practical examples of using C++ lambda expressions for data filtering and transformation?. For more information, please follow other related articles on the PHP Chinese website!
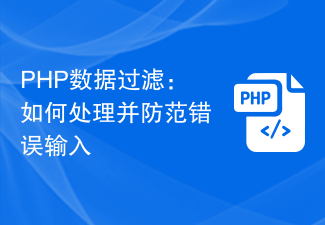
PHP数据过滤:如何处理并防范错误输入在开发Web应用程序中,用户的输入数据是无法可靠的,因此数据的过滤和验证是非常重要的。PHP提供了一些函数和方法来帮助我们处理和防范错误输入,本文将讨论一些常见的数据过滤技术,并提供示例代码。字符串过滤在用户输入中,我们经常会遇到那些包含HTML标签、特殊字符或者恶意代码的字符串。为了防止安全漏洞和脚本注入攻
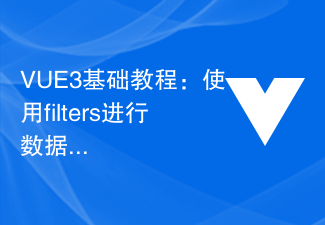
VUE3是目前前端开发中较为流行的一种框架,其所提供的基础功能能够极大的提高前端开发效率。其中filters就是VUE3中一个非常有用的工具,使用filters可以很方便地对数据进行筛选、过滤和处理。那么什么是filters呢?简单来说,filters就是VUE3中的过滤器。它们可以用于处理被渲染的数据,以便在页面中呈现出更加理想的结果。filters是一些
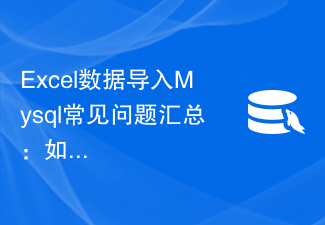
Excel数据导入Mysql常见问题汇总:如何处理导入过程中的重复数据?在数据处理的过程中,我们常常会遇到Excel数据导入到Mysql数据库的需求。然而,由于数据量庞大,很容易出现重复数据的情况,这就需要我们在导入过程中进行相应的处理。在本文中,我们将讨论如何处理导入过程中的重复数据,并提供相应的代码示例。在进行重复数据处理之前,首先需要确保数据表中存在唯
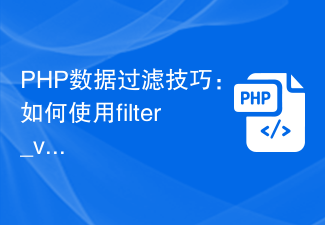
PHP数据过滤技巧:如何使用filter_var函数验证用户输入在Web开发中,用户输入数据的验证和过滤是非常重要的环节。恶意用户可能会利用不良输入来进行攻击或者破坏系统。PHP提供了一系列的过滤函数来帮助我们处理用户输入数据,其中最常用的是filter_var函数。filter_var函数是基于过滤器的一种验证用户输入的方式。它允许我们使用各种内置的过滤器
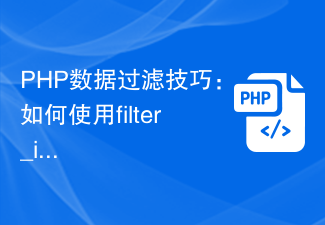
PHP数据过滤技巧:如何使用filter_input函数验证和清理用户输入在开发Web应用程序时,用户输入的数据是不可避免的。为了确保输入数据的安全性和有效性,我们需要对用户输入进行验证和清理。在PHP中,filter_input函数是一个非常有用的工具,可以帮助我们完成这个任务。本文将介绍如何使用filter_input函数验证和清理用
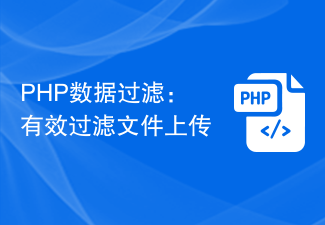
PHP数据过滤:有效过滤文件上传文件上传是Web开发中常见的功能之一,然而文件上传也是潜在的安全风险之一。黑客可能利用文件上传功能来注入恶意代码或者上传违禁文件。为了保证网站的安全性,我们需要对用户上传的文件进行有效的过滤和验证。在PHP中,我们可以使用一系列函数和技巧来过滤和验证用户上传的文件。下面是一些常用的方法和代码示例:检查文件类型在接收用户上传的文
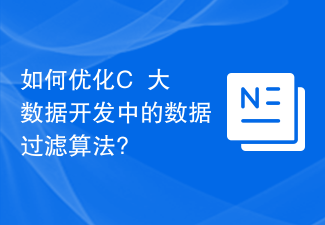
如何优化C++大数据开发中的数据过滤算法?在大数据开发中,数据过滤是一项非常常见而又重要的任务。在处理海量数据时,如何高效地进行数据过滤,是提升整体性能和效率的关键。本文将介绍如何优化C++大数据开发中的数据过滤算法,并给出相应的代码示例。使用适当的数据结构在数据过滤过程中,选择适当的数据结构是至关重要的。一种常用的数据结构是哈希表,它可以快速进行数据查找。
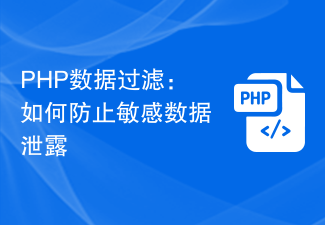
PHP数据过滤:如何防止敏感数据泄露随着互联网的快速发展,数据的泄露已经成为了一项严重的安全威胁。泄露的数据包括个人身份证号码、银行账号、密码等敏感信息,一旦被黑客获取,就会对用户的财产和隐私造成严重的损害。在开发网站或应用程序时,对于用户提交的数据必须进行过滤,以防止敏感数据的泄露和滥用。本文将介绍一些PHP数据过滤的方法和注意事项,并结合代码示例进行说明


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
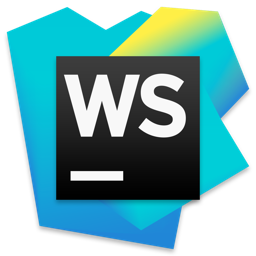
WebStorm Mac version
Useful JavaScript development tools
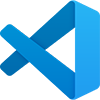
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
