


Function signature design principles for Golang function performance optimization
Go function performance optimization principle: use value receivers first: avoid pointer receivers to improve concurrency performance. Avoid named return values: Use return tuples or Errors when possible to handle errors and multiple return values. Optimize slice and map passes: Use pointer references to avoid unnecessary copies. Consider concurrency safety: Use mutexes or read-write locks to protect shared data. Optimize string concatenation: use strings.Builder instead of operator. Optimize array search: Use map instead of linear array search to improve search speed.
Go language function performance optimization: function signature design principles
In the Go language, function signatures will have a significant impact on the performance of the function. A well-designed function signature can simplify calls, improve readability, and optimize performance.
Prefer using value receivers
Using composite types such as structures or slices as value receivers (passing a copy) instead of pointer receivers (passing a reference) can avoid unnecessary memory allocation and Improve concurrency performance.
Example:
// 值接收器 func UpdateUser(user User) { // 对 user 副本进行修改 } // 指针接收器 func UpdateUserPtr(user *User) { // 对 user 原对象进行修改 }
Avoid using named return values
Although named return values are convenient to use, they will increase the size of the stack frame and increase the call overhead have negative impacts. Prefer using the return tuple or Errors technique to handle errors and multiple return values.
Example:
// 避免命名返回值 func GetUserInfo(id int) (name string, age int, err error) { // ... } // 使用返回元组 func GetUserInfoTuple(id int) (string, int, error) { // ... }
Optimize passing of slices and maps
Using slices and maps as value receivers or return values creates copies, thus reducing performance. To avoid unnecessary copies, pointer references can be used.
Example:
// 值接收器 func SortSlice(slice []int) { // 对 slice 副本进行排序 } // 指针引用 func SortSlicePtr(slice *[]int) { // 对 slice 原对象进行排序 }
Consider concurrency safety
If a function is called in a concurrent environment, concurrency safety must be ensured. Shared data should be protected using a mutex (Mutex) or a read-write lock (RWMutex).
Example:
import "sync" var mutex = &sync.Mutex{} // 并发安全的函数 func GetConcurrentData() (data string) { mutex.Lock() defer mutex.Unlock() // ... return data }
Practical case
Optimizing string splicing
String splicing is a For common operations, performance can be optimized by using strings.Builder
instead of the
operator.
// 使用 + 运算符 func ConcatenateStrings(a, b string) string { return a + b } // 使用 strings.Builder func ConcatenateStringsBuilder(a, b string) string { var builder strings.Builder builder.WriteString(a) builder.WriteString(b) return builder.String() }
Optimize array search
Using map
instead of linear array search can greatly improve the search speed, especially when the amount of data is large .
// 线性数组查找 func FindInArray(array []string, element string) int { for i := 0; i < len(array); i++ { if array[i] == element { return i } } return -1 } // map 查找 func FindInMap(m map[string]bool, element string) bool { return m[element] }
The above is the detailed content of Function signature design principles for Golang function performance optimization. For more information, please follow other related articles on the PHP Chinese website!
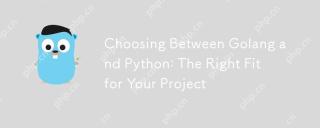
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
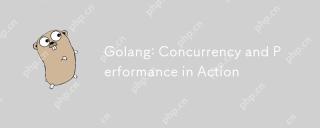
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
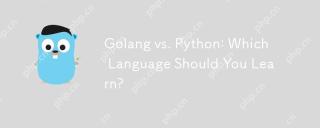
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
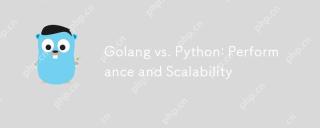
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
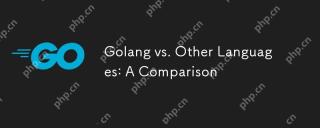
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
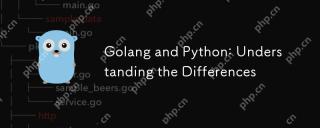
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
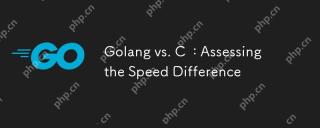
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
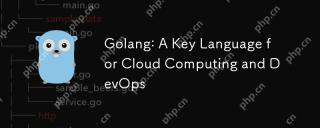
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
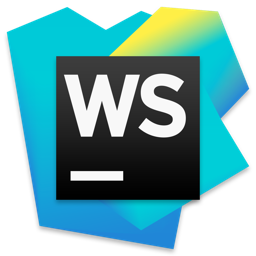
WebStorm Mac version
Useful JavaScript development tools
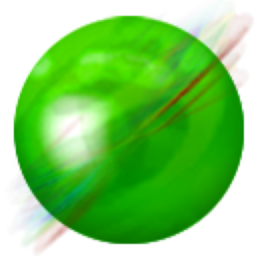
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.