


What are the advantages of using C++ lambda expressions for multi-threaded programming?
The advantages of lambda expressions in C multi-threaded programming include simplicity, flexibility, ease of parameter passing, and parallelism. Practical case: Use lambda expressions to create multi-threads and print thread IDs in different threads, demonstrating the simplicity and ease of use of this method.
Advantages of using C lambda expressions to implement multi-threaded programming
Introduction
lambda expressions are a powerful feature introduced in C 11 that can represent function objects within a block. In multi-threaded programming, lambda expressions provide a concise and powerful way to define parallel tasks.
Advantages
The main advantages of using lambda expressions for multi-threaded programming include:
- Simplicity: Lambda expressions are concise and easy to read, thus simplifying multi-threaded code.
- Flexibility: lambda expressions can capture external variables, allowing dynamic access to data.
- Easy to pass parameters: Lambda expressions can be easily passed as parameters to other functions and threads.
- Parallelism: Lambda expressions can be executed simultaneously, improving application performance.
Practical case
The following example shows how to use lambda expressions to create a multi-threaded program in C:
#include <iostream> #include <thread> #include <vector> int main() { // 创建一个 vector 来存储线程 std::vector<std::thread> threads; // 使用 lambda 表达式定义并行任务 auto task = [](const int &n) { std::cout << "Thread " << n << " is running." << std::endl; }; // 创建并启动线程 for (int i = 0; i < 10; i++) { threads.emplace_back(std::thread(task, i)); } // 等待线程完成 for (auto &thread : threads) { thread.join(); } return 0; }
Run Result :
Thread 0 is running. Thread 1 is running. Thread 2 is running. ... Thread 9 is running.
In this example, a lambda expression is used to define a parallel task that prints the ID of the thread. The program creates 10 threads and uses lambda expressions to execute these tasks in parallel. Using lambda expressions, we write concise and understandable multi-threaded code.
The above is the detailed content of What are the advantages of using C++ lambda expressions for multi-threaded programming?. For more information, please follow other related articles on the PHP Chinese website!
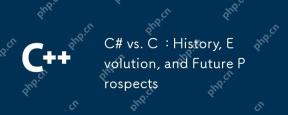
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
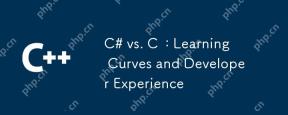
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
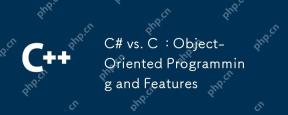
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
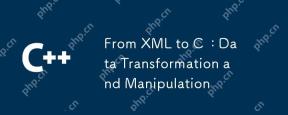
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
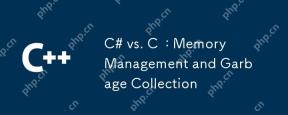
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
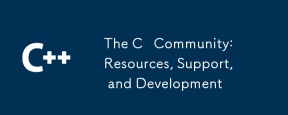
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
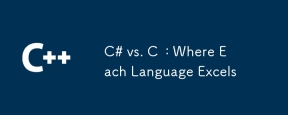
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
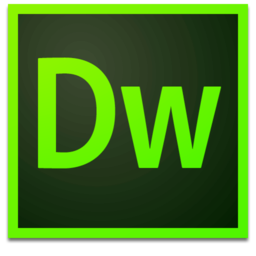
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
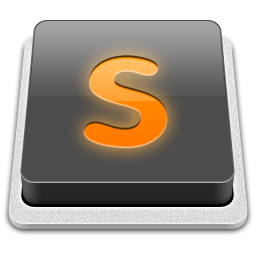
SublimeText3 Mac version
God-level code editing software (SublimeText3)
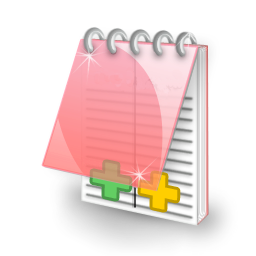
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function