The event publish-subscribe model in Spring Framework is a design pattern that allows objects to communicate by publishing and subscribing to events without direct references. Publishers publish events, while subscribers receive and process events. Spring provides an out-of-the-box event model based on Java's java.util.EventListener and java.util.EventObject interfaces. Publishing events is accomplished through the ApplicationEventPublisher interface, and subscribing to events is accomplished by implementing the ApplicationListener interface and using the @EventListener annotation. In practice, the event publish-subscribe model can be used to achieve decoupled communication without directly coupling application components, such as sending email notifications after a user is created.
Implementation of event publishing-subscription model in Spring Framework
Concept introduction
Event publishing-subscription model Is a design pattern that allows objects to communicate with each other without direct references. In the example, the publisher publishes events, and the subscribers receive and process these events.
Event model in Spring
Spring Framework provides an out-of-the-box event publish-subscribe model, which is based on Java's java.util. EventListener
and java.util.EventObject
interfaces.
Event publishing
Event publishing is completed by the ApplicationEventPublisher
interface. It allows publishers to publish events by calling the publishEvent()
method.
Code example: Publishing events
// 事件定义 class MyEvent extends ApplicationEvent { public MyEvent(Object source) { super(source); } } // 发布器 ApplicationEventPublisher publisher = ...; publisher.publishEvent(new MyEvent(this));
Event subscription
Subscribers implement the ApplicationListener
interface And use the @EventListener
annotation to subscribe to events.
Code example: Subscription event
// 订阅者类 public class MyEventListener implements ApplicationListener<MyEvent> { @Override public void onApplicationEvent(MyEvent event) { // 处理事件 } } // 使用 @EventListener 注解订阅 @Component public class EventListenerRegistrar { @EventListener public void handleEvent(MyEvent event) { // 处理事件 } }
Practical case
Consider a sample application that needs to be sent after the user is created Email notification. To do this, create a CreateUserEvent
event and add the sendEmail()
method to it.
Code Example: Practical Case
// 事件定义 class CreateUserEvent extends ApplicationEvent { // ... 其他代码 public void sendEmail() { // 发送电子邮件 } } // 发布器 void onCreateUser(User user) { // ... 其他代码 publisher.publishEvent(new CreateUserEvent(user)); } // 订阅者 @EventListener public void handleEvent(CreateUserEvent event) { event.sendEmail(); }
In this way, Spring's event publish-subscribe model provides a flexible and scalable way to allow our Application components communicate with each other without being directly coupled.
The above is the detailed content of How is the event publish-subscribe model implemented in Spring Framework?. For more information, please follow other related articles on the PHP Chinese website!
![事件 ID 4660:已删除对象 [修复]](https://img.php.cn/upload/article/000/887/227/168834320512143.png)
我们的一些读者遇到了事件ID4660。他们通常不确定该怎么做,所以我们在本指南中解释。删除对象时通常会记录事件ID4660,因此我们还将探索一些实用的方法在您的计算机上修复它。什么是事件ID4660?事件ID4660与活动目录中的对象相关,将由以下任一因素触发:对象删除–每当从ActiveDirectory中删除对象时,都会记录事件ID为4660的安全事件。手动更改–当用户或管理员手动更改对象的权限时,可能会生成事件ID4660。更改权限设置、修改访问级别或添加或删除人员或组时,可能会发生这种情
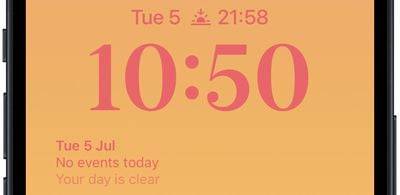
在运行iOS16或更高版本的iPhone上,您可以直接在锁定屏幕上显示即将到来的日历事件。继续阅读以了解它是如何完成的。由于表盘复杂功能,许多AppleWatch用户习惯于能够看一眼手腕来查看下一个即将到来的日历事件。随着iOS16和锁定屏幕小部件的出现,您可以直接在iPhone上查看相同的日历事件信息,甚至无需解锁设备。日历锁定屏幕小组件有两种风格,允许您跟踪下一个即将发生的事件的时间,或使用更大的小组件来显示事件名称及其时间。若要开始添加小组件,请使用面容ID或触控ID解锁iPhone,长按
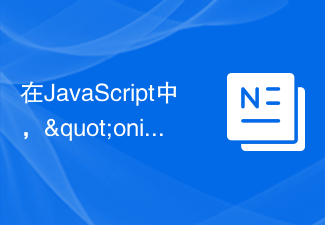
当在输入框中添加值时,就会发生oninput事件。您可以尝试运行以下代码来了解如何在JavaScript中实现oninput事件-示例<!DOCTYPEhtml><html> <body> <p>Writebelow:</p> <inputtype="text"
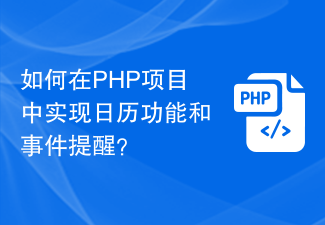
如何在PHP项目中实现日历功能和事件提醒?在开发Web应用程序时,日历功能和事件提醒是常见的需求之一。无论是个人日程管理、团队协作,还是在线活动安排,日历功能都可以提供便捷的时间管理和事务安排。在PHP项目中实现日历功能和事件提醒可以通过以下步骤来完成。数据库设计首先,需要设计数据库表来存储日历事件的相关信息。一个简单的设计可以包含以下字段:id:事件的唯一
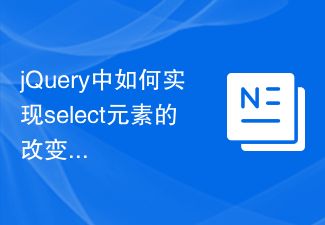
jQuery是一个流行的JavaScript库,可以用来简化DOM操作、事件处理、动画效果等。在web开发中,经常会遇到需要对select元素进行改变事件绑定的情况。本文将介绍如何使用jQuery实现对select元素改变事件的绑定,并提供具体的代码示例。首先,我们需要使用标签来创建一个包含选项的下拉菜单:
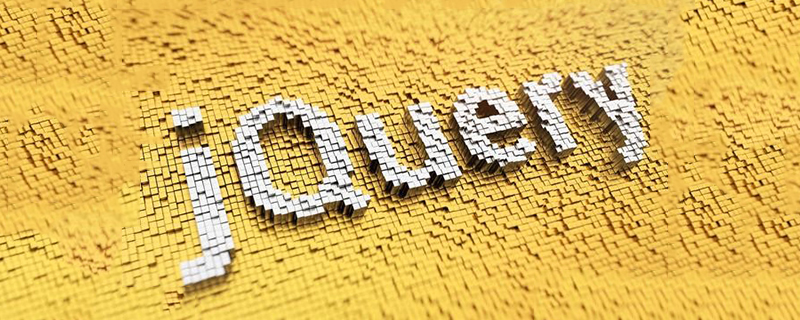
jquery中常用的事件有:1、window事件;2、鼠标事件,是当用户在文档上面移动或单击鼠标时而产生的事件,包括鼠标单击、移入事件、移出事件等;3、键盘事件,是用户每次按下或者释放键盘上的按键时都会产生事件,包括按下按键事件、释放按键按键等;4、表单事件,例如当元素获得焦点时会触发focus()事件,失去焦点时会触发blur()事件,表单提交时会触发submit()事件。
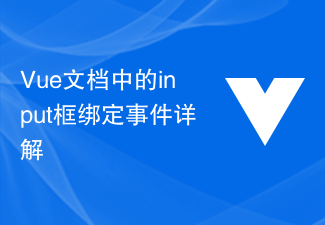
Vue.js是一种轻量级的JavaScript框架,具有易用、高效和灵活的特点,是目前广受欢迎的前端框架之一。在Vue.js中,input框绑定事件是一个十分常见的需求,本文将详细介绍Vue文档中的input框绑定事件。一、基础概念在Vue.js中,input框绑定事件指的是将输入框的值绑定到Vue实例的数据对象中,从而实现输入和响应的双向绑定。在Vue.j
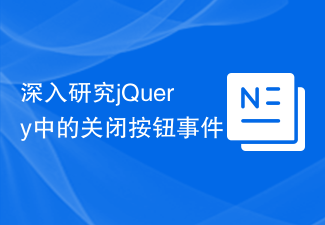
深入理解jQuery中的关闭按钮事件在前端开发过程中,经常会遇到需要实现关闭按钮功能的情况,比如关闭弹窗、关闭提示框等。而在使用jQuery这个流行的JavaScript库时,实现关闭按钮事件也变得异常简单和方便。本文将深入探讨如何利用jQuery来实现关闭按钮事件,并提供具体的代码示例,帮助读者更好地理解和掌握这个技术。首先,我们需要了解在HTML中如何定


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
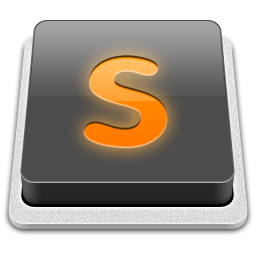
SublimeText3 Mac version
God-level code editing software (SublimeText3)
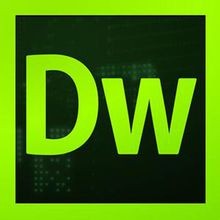
Dreamweaver CS6
Visual web development tools
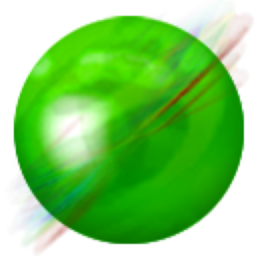
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
