How do lambda expressions handle exceptions in C++?
In C, there are two ways to handle exceptions using Lambda expressions: catch the exception using a try-catch block, and handle or rethrow the exception in the catch block. Use a wrapper function of type std::function
Using Lambda expressions to handle exceptions in C
Introduction
Lambda An expression is an anonymous function that captures external variables and passes parameters by value or reference. In C, lambda expressions can be used for a variety of purposes, including handling exceptions.
Using try-catch blocks
The try-catch block is the standard way to handle exceptions in Lambda expressions. The catch block allows catching specific types of exceptions or all exceptions. The following example demonstrates how to use a try-catch block in a Lambda expression to handle exceptions:
#include <functional> #include <iostream> int main() { auto lambda = [](int x) -> int { try { return x / 0; // 将引发 std::runtime_error 异常 } catch (const std::exception& e) { std::cout << "Exception caught: " << e.what() << std::endl; return -1; } }; int result = lambda(10); std::cout << "Result: " << result << std::endl; return 0; }
Using std::function
Another way to handle exceptions in a Lambda expression The exception method is to use std::function
. std::function
is a wrapper function that can accept different function types, including Lambda expressions. std::function
provides a try_emplace
method that allows exceptions to be caught in Lambda expressions. The following example demonstrates how to use std::function
to handle exceptions:
#include <functional> #include <iostream> int main() { std::function<int(int)> lambda; try { lambda = [](int x) -> int { return x / 0; }; // 将引发 std::runtime_error 异常 } catch (const std::exception& e) { std::cout << "Exception caught: " << e.what() << std::endl; lambda = [](int x) -> int { return -1; }; } int result = lambda(10); std::cout << "Result: " << result << std::endl; return 0; }
Practical example
Consider a function with the following interface:
int do_something(const std::string& input);
This function may raise a std::invalid_argument
exception if the input
is invalid. We can use a Lambda expression and a try-catch
block to handle this exception as follows:
auto do_something_safe = [](const std::string& input) -> int { try { return do_something(input); } catch (const std::invalid_argument& e) { // 处理异常并返回 -1 std::cout << "Invalid input: " << e.what() << std::endl; return -1; } };
We can then safely call do_something_safe
in our code , without explicitly handling the exception.
The above is the detailed content of How do lambda expressions handle exceptions in C++?. For more information, please follow other related articles on the PHP Chinese website!
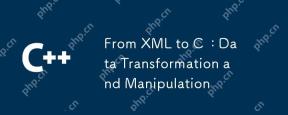
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
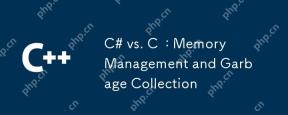
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
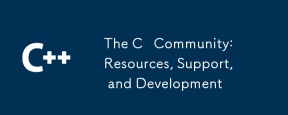
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
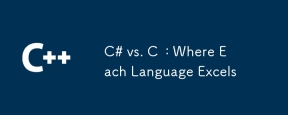
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
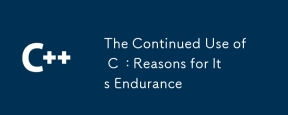
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
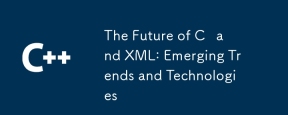
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
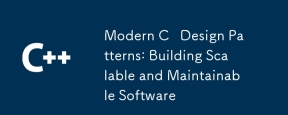
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
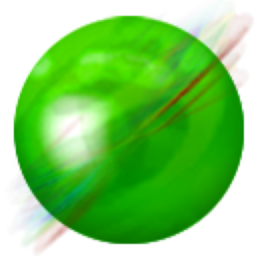
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
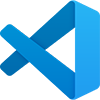
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft