When processing large amounts of data in Go function testing, you can use Mock for simulation through the following strategies: 1. Use third-party libraries (Mockery, go-mockgen, wiremocksvc); 2. Use built-in interfaces. For example, when using Mock to simulate a large number of users, you can define a UserMock structure and provide mock behavior for its GetUsers method. By using mocks, you can ensure that functions run as expected without actually affecting the database.
Data volume Mock strategy in Go function testing
In Go function testing, it is often necessary to process a large amount of data Case. To avoid impact on the actual database or service, you can use Mock to simulate the amount of data.
1. Use third-party libraries
- Mockery: A tool to generate type-safe Mock.
- go-mockgen: Able to generate Mock based on the interface.
- wiremocksvc: A Go library for creating and managing WireMock servers.
2. Use the built-in interface
You can define an interface in Go and use an empty structure as its type:
type User struct{}
Then, you can Use the following code to mock like using the Mock library:
var mockUser = User{}
3. Practical case: simulate a large number of users
Consider a functionGetUsers()
, this function acquires a large number of users. Using mocks ensures that functions run as expected without actually getting data from the database.
Mock Definition:
import "context" // UserMock mocks the User interface. type UserMock struct { GetUsersFunc func(ctx context.Context) ([]User, error) } // GetUsers provides mock implementation for User.GetUsers. func (u *UserMock) GetUsers(ctx context.Context) ([]User, error) { return u.GetUsersFunc(ctx) }
Function Test:
import ( "context" "testing" "your_module/pkg/users" ) func TestGetUsers(t *testing.T) { // Create a User mock. mockUser := &UserMock{} // Define the mock behavior. mockUser.GetUsersFunc = func(ctx context.Context) ([]users.User, error) { return []users.User{ {ID: 1}, {ID: 2}, }, nil } // Perform the test with the mock. users := users.GetUsersWithMock(context.Background(), mockUser) if len(users) != 2 { t.Errorf("Expected 2 users, got %d...", len(users)) } }
Tips:
- When using Mocks, be sure to clearly define the mock behavior to avoid unexpected results.
- Close the Mock service early during testing to release resources.
- Regularly check the Mock library to ensure compatibility.
The above is the detailed content of Data volume Mock strategy in Golang function testing. For more information, please follow other related articles on the PHP Chinese website!
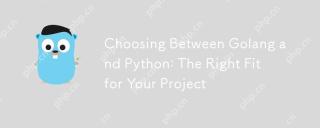
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
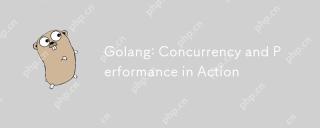
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
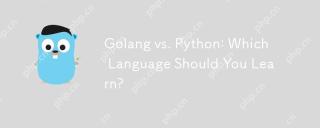
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
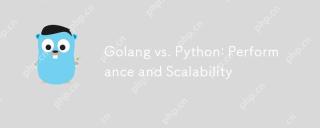
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
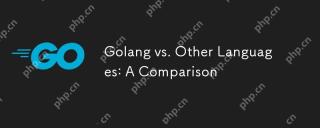
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
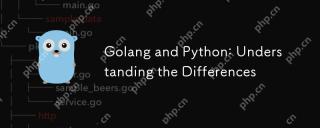
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
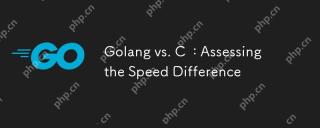
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
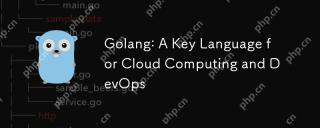
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
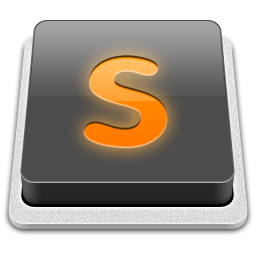
SublimeText3 Mac version
God-level code editing software (SublimeText3)