Ways to simulate real environments in Go function testing: Dependency injection: Use test doubles to replace real dependencies, isolate functions and control input. Docker containers: Run code in an isolated environment, set exact dependencies and configuration, and access real external services.
Simulating the real environment in Go function testing
When unit testing Go functions, simulating the real environment helps Ensure their correct operation in various scenarios. Here's how to do it:
Using Dependency Injection
Dependency injection is a technique used to provide instances of a function's dependencies while it is running. This allows us to replace real dependencies with test doubles (such as mocks or stubs), thus isolating the function and controlling its inputs.
// 服务对象 type Service struct { repo Repository } // Repository 接口 type Repository interface { Get(id int) (*User, error) } // 测试代码 func TestService_GetUser(t *testing.T) { // 使用模拟存储库 mockRepo := &MockRepository{} mockRepo.On("Get").Return(&User{ID: 123, Name: "John Doe"}, nil) // 使用依赖项注入创建服务 service := &Service{ repo: mockRepo, } // 调用函数 user, err := service.GetUser(123) // 验证结果 if err != nil { t.Errorf("Expected nil error, got %v", err) } if user.ID != 123 || user.Name != "John Doe" { t.Errorf("Expected user with ID 123 and name 'John Doe', got %v", user) } }
When testing functions, we can replace Repository
with MockRepository
and control its return value. This allows us to test the behavior of the function under different data scenarios without having to call the real data store.
Using Docker Containers
Another way to simulate a real environment is to use Docker containers. Containers allow us to run code in an isolated environment where exact dependencies and configurations can be set.
// 测试代码 func TestHandler(t *testing.T) { // 创建 Docker 容器 container, err := docker.NewContainer(docker.Builder{Image: "redis"}) if err != nil { t.Fatalf("Could not create container: %v", err) } // 启动容器 if err := container.Start(); err != nil { t.Fatalf("Could not start container: %v", err) } // 访问 Redis 服务 client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", }) // 测试 HTTP 请求处理程序,将 Redis 客户端传递给处理程序 w := httptest.NewRecorder() req, _ := http.NewRequest("GET", "/", nil) handler(w, req, client) // 验证响应 if w.Code != http.StatusOK { t.Errorf("Expected status code 200, got %d", w.Code) } }
In this example, we start a Redis container before testing the function. This way we can access the real Redis service and verify the actual behavior of the function.
The above is the detailed content of How to simulate the real environment in Golang function testing?. For more information, please follow other related articles on the PHP Chinese website!
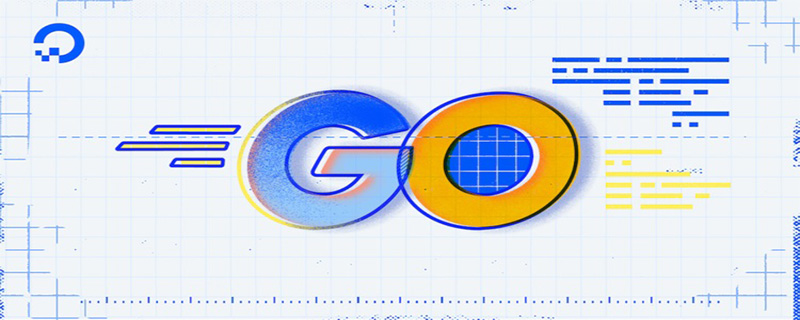
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
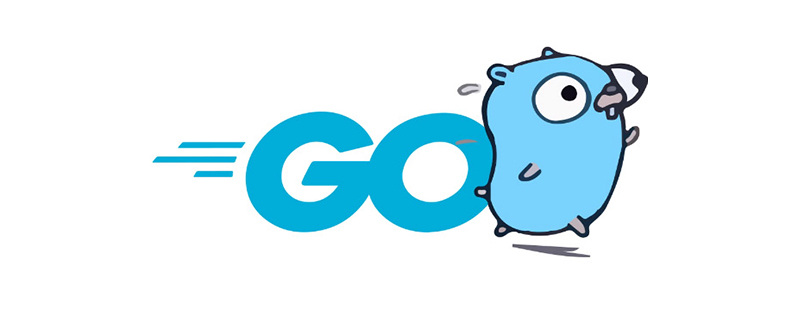
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
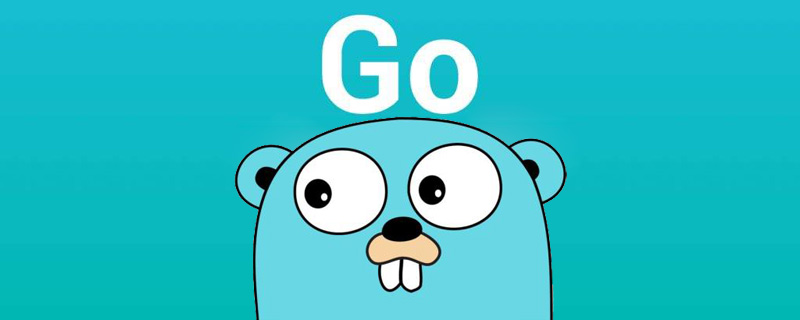
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
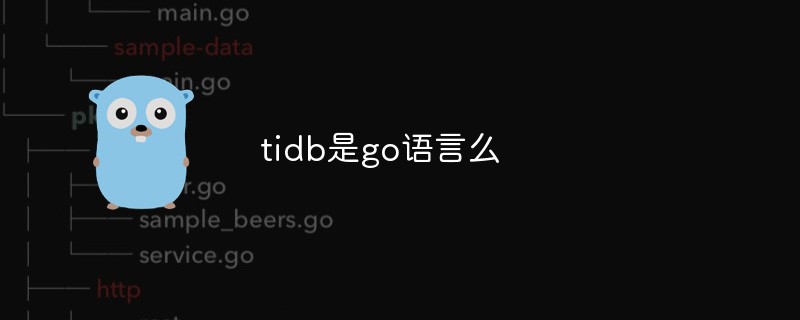
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
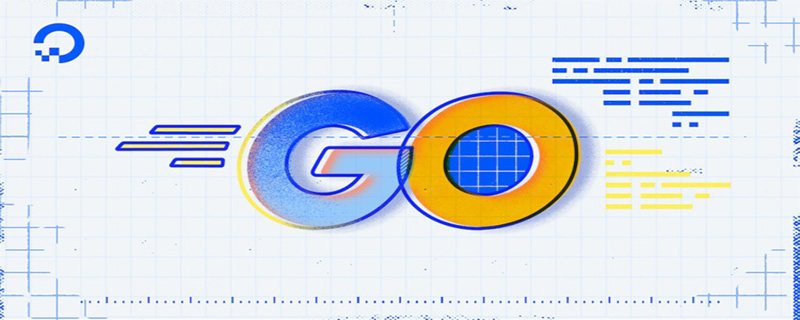
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
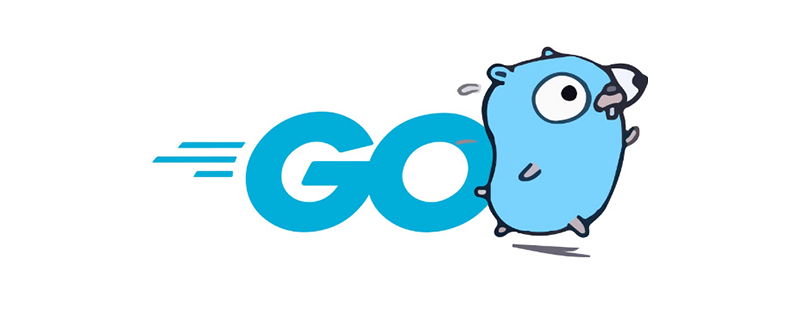
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
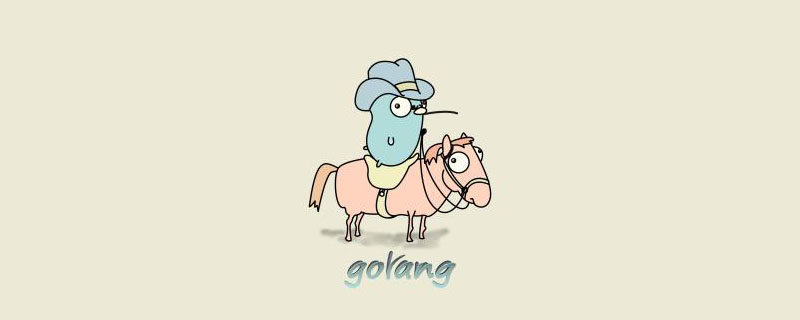
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
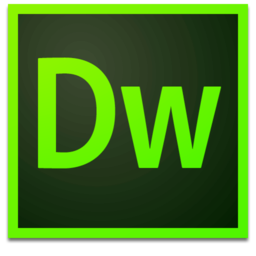
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
