The impact of serialization on Java performance: The serialization process relies on reflection, which will significantly affect performance. Serialization requires the creation of a byte stream to store object data, resulting in memory allocation and processing costs. Serializing large objects consumes a lot of memory and time. Serialized objects increase load when transmitted over the network.
The impact of Java serialization on performance
Preface
Serialization is The process of converting an object into a stream of bytes for storage or transmission. Serialization in Java is implemented using the java.io.Serializable
interface. While serialization is very convenient, it can have a significant impact on performance.
Performance issues
- Reflection: The serialization process relies on reflection, which can have a significant impact on performance.
- Byte stream creation: Serialization requires the creation of a byte stream to store object data, which incurs memory allocation and processing costs.
- Large objects: Serializing large objects consumes a lot of memory and time.
- Network transmission: Serialized objects will increase the load when transmitted over the network.
Practical Case
To demonstrate the impact of serialization on performance, let us consider the following code sample:
import java.io.*; public class SerializationBenchmark { public static void main(String[] args) throws IOException { // 创建一个要序列化的对象 Object object = new Object(); // 序列化对象 ByteArrayOutputStream out = new ByteArrayOutputStream(); ObjectOutputStream oos = new ObjectOutputStream(out); oos.writeObject(object); oos.flush(); // 反序列化对象 ByteArrayInputStream in = new ByteArrayInputStream(out.toByteArray()); ObjectInputStream ois = new ObjectInputStream(in); Object deserializedObject = ois.readObject(); // 测量序列化和反序列化时间 long serializationTime = System.nanoTime(); oos.writeObject(object); oos.flush(); serializationTime = System.nanoTime() - serializationTime; long deserializationTime = System.nanoTime(); ois.readObject(); deserializationTime = System.nanoTime() - deserializationTime; // 输出时间 System.out.println("Serialization time: " + serializationTime + " nanoseconds"); System.out.println("Deserialization time: " + deserializationTime + " nanoseconds"); } }
Run this code sample , you will see that serialization and deserialization times are significantly longer than simple object operations. For large objects, the time difference will be greater.
Best Practices
To reduce the performance impact of serialization, consider the following best practices:
- Only Serialize necessary data: Do not serialize unnecessary data.
- Using external serialization libraries: Instead of Java's built-in serialization implementation, you can use external libraries such as Kryo or Protobuf, which provide better performance.
- Avoid multiple serializations: If possible, avoid multiple serializations of the same object.
-
Use the transient keyword: For fields that do not need to be serialized, use the
transient
keyword.
By following these best practices, you can minimize the impact of serialization on your Java application performance.
The above is the detailed content of How does Java serialization affect performance?. For more information, please follow other related articles on the PHP Chinese website!
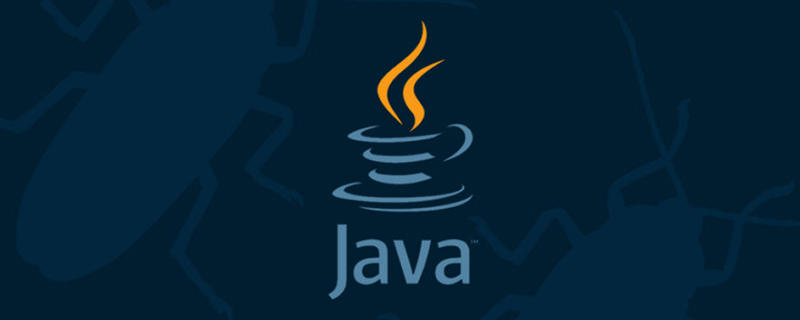
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
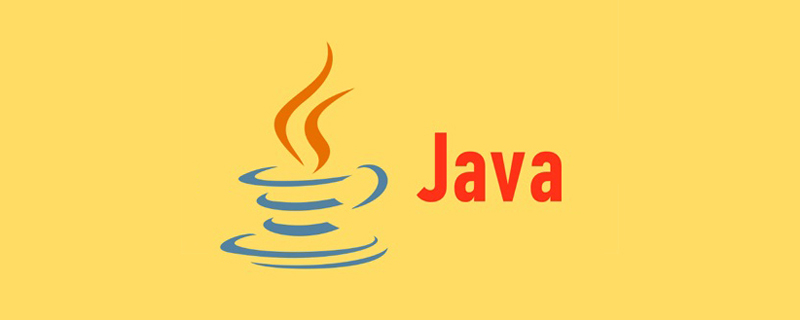
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
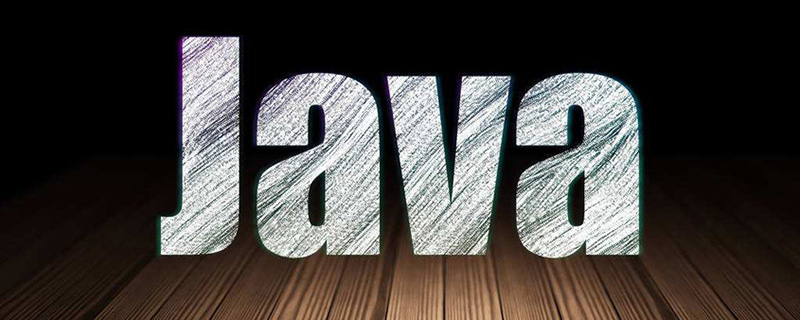
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
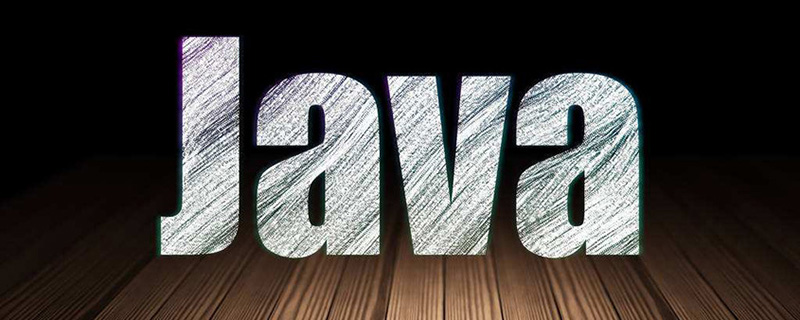
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
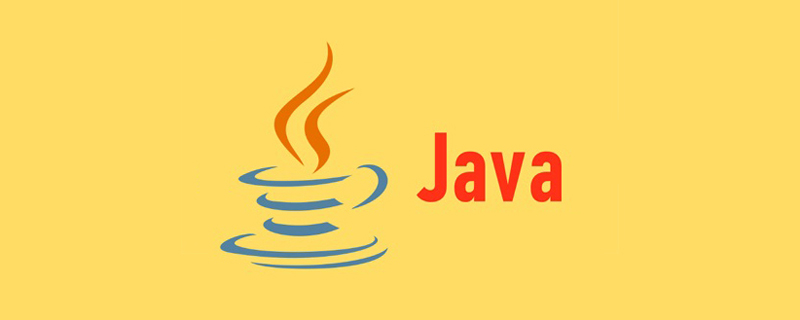
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
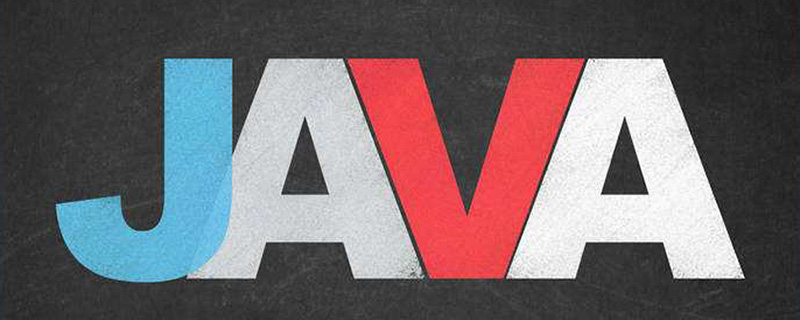
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
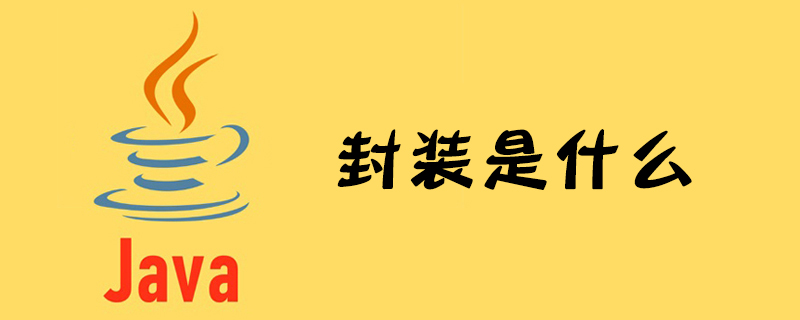
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
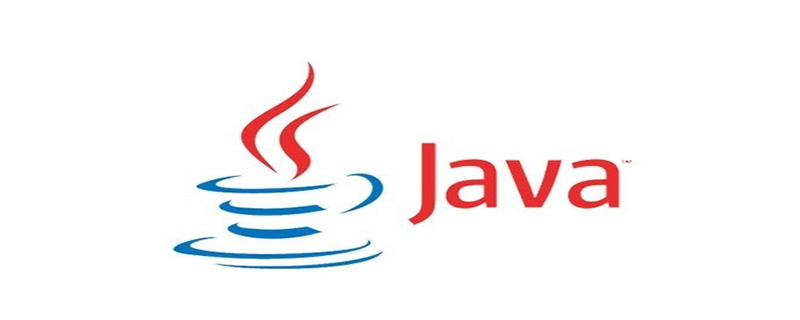
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
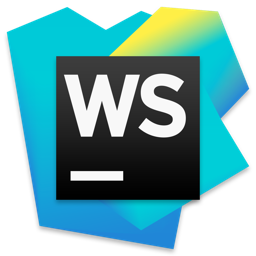
WebStorm Mac version
Useful JavaScript development tools
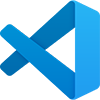
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
