The case of function pointers and closures in Golang web development
Application of function pointers and closures in Go Web development: Function pointers: allow the called function to be dynamically changed, improving flexibility. Decouple code and simplify maintenance. Practical example: Handling HTTP routing, binding controller handlers to different routes. Closure: access variables outside the creation scope and capture data. Practical example: Create private data structures to share data between handlers.
The case of function pointers and closures in Go Web development
In Go Web development, function pointers and closures are powerful mechanisms that can Improve code flexibility and reusability.
Function pointer
The function pointer is a variable pointing to the memory address of the function. Using a function pointer has the following advantages over calling a function directly:
- Flexibility: Function pointers allow you to dynamically change the function to be called at runtime.
- Decoupling: Passing function pointers as parameters can decouple different parts of the code, making them easier to maintain.
Practical case: Processing HTTP routing
We can use function pointers to create controllers that handle HTTP routing:
// 定义一组不同的控制器处理程序。 var handlers = map[string]func(http.ResponseWriter, *http.Request){ "/home": handleHome, "/about": handleAbout, "/contact": handleContact, } // 路由请求到不同的处理程序。 func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { handler, ok := handlers[r.URL.Path] if ok { handler(w, r) } else { http.Error(w, "404 Not Found", http.StatusNotFound) } }) }
Closure
A closure is a function that can access variables in a scope outside the scope in which it was created. This allows us to capture data in a specific context, even after the function itself has returned.
Practical Case: Creating Private Data Structures
We can use closures to create private data structures in Go Web handlers:
// 提供用于在处理程序之间共享数据的私有结构。 type Context struct { user *User cart *Cart } // 创建一个工厂函数来创建带有私有上下文的闭包。 func WithContext(ctx *Context) func(http.ResponseWriter, *http.Request) { return func(w http.ResponseWriter, r *http.Request) { // ... 使用处理程序中的私有上下文 ... } }
By using function pointers and closures, You can create flexible and scalable code in Go web development.
The above is the detailed content of The case of function pointers and closures in Golang web development. For more information, please follow other related articles on the PHP Chinese website!
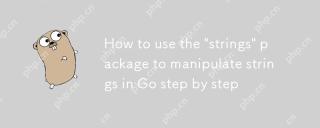
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
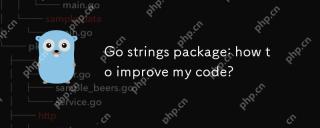
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
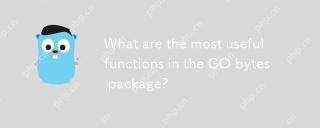
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
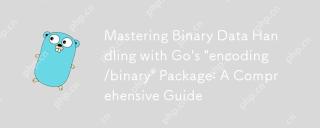
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
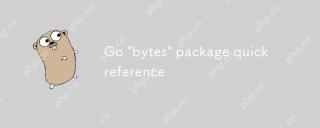
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
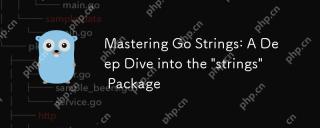
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
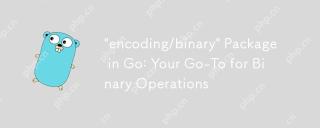
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
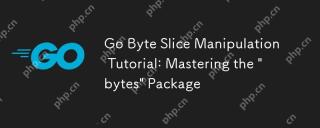
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
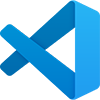
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
