The impact of function pointers and closures on concurrent programming
The impact of function pointers and closures on concurrent programming: Function pointers are used to create callback functions, which are easy to pass and call, and reduce code redundancy. Closures are used to create shared state and simplify parallel programming by capturing references to external variables. When using function pointers and closures, you need to be aware of thread safety, memory leaks, and performance overhead.
The impact of function pointers and closures on concurrent programming
Function pointers and closures are powerful features in C that can significantly impact concurrent programming .
Function pointer
Function pointer is a pointer to a function. In concurrent programming, they are very useful for creating callback functions. A callback function is a function that is called when an event occurs. Using function pointers, you can easily create and pass callback functions without duplicating code in many places.
Practical example:
// 回调函数 void callback(int x) { std::cout << "回调函数被调用,参数为:" << x << std::endl; } // 创建线程,传递回调函数 std::thread t(callback, 10);
Closure
A closure is a function object that references external variables. In concurrent programming, closures are useful for creating shared state. Shared state refers to variables accessed by multiple threads. Closures achieve this by capturing a reference to shared state into their internal state.
Practical example:
// 闭包 auto counter = []() { static int count = 0; return ++count; }; // 创建线程,并行调用闭包 std::vector<std::thread> threads; for (int i = 0; i < 10; i++) { threads.emplace_back([&counter]() { std::cout << "线程 " << std::this_thread::get_id() << ":计数为 " << counter() << std::endl; }); }
Closures and function pointers can greatly simplify concurrent programming, but you need to pay attention to the following:
- Thread safety: Ensure that callback functions and closures are thread-safe in a multi-threaded environment.
- Memory leaks: Avoid catching circular references to external variables, which may cause memory leaks.
- Performance: Using function pointers and closures may incur a slight performance overhead, especially when called frequently.
The above is the detailed content of The impact of function pointers and closures on concurrent programming. For more information, please follow other related articles on the PHP Chinese website!
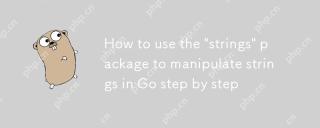
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
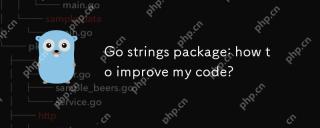
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
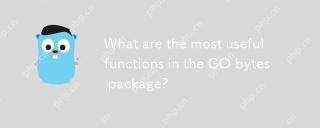
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
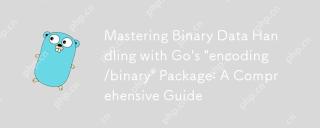
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
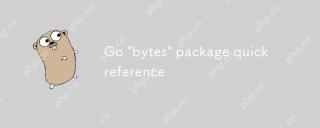
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
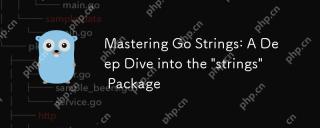
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
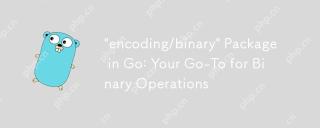
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
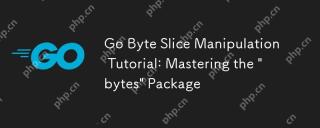
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
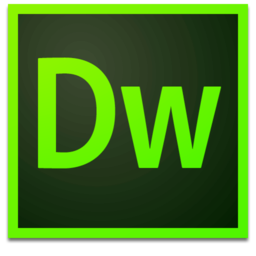
Dreamweaver Mac version
Visual web development tools
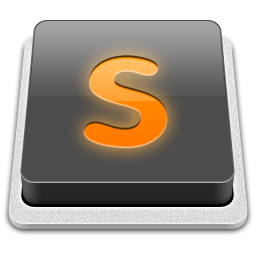
SublimeText3 Mac version
God-level code editing software (SublimeText3)
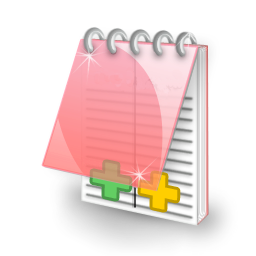
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
