C++ function overloading and function inlining
Function overloading and function inlining Function overloading allows the creation of multiple functions with the same name but different parameter lists, writing specific code for different input types. Function inlining is a compiler optimization that inserts function code directly into the call point to improve program speed.
C Function Overloading and Function Inlining
Function Overloading
Function Overloading allows you to create Multiple functions with the same name but different parameter lists. This allows you to write target-specific code based on different types or amounts of input.
Syntax:
returnType functionName(parameter1, parameter2, ...); returnType functionName(parameter1, parameter2, ..., parameterN);
Example:
int sum(int a, int b) { return a + b; } double sum(double a, double b) { return a + b; }
Function inline
Function inlining is a compiler optimization technique that inserts function code directly into the call site (rather than jumping to the function through a function call). This can improve the speed of your program, especially if the function is called frequently.
Grammar:
For functions:
inline returnType functionName(parameter1, parameter2, ...);
For member functions:
inline returnType className::memberFunctionName(parameter1, parameter2, ...);
Actual cases:
Suppose you want to calculate the area of different shapes. You can use function overloading to create specific area calculation functions for each shape.
Example:
#include <iostream> using namespace std; double area(int radius) { return 3.14 * radius * radius; } double area(int length, int width) { return length * width; } double area(int base, int height) { return 0.5 * base * height; } int main() { cout << "圆的面积: " << area(5) << endl; cout << "矩形的面积: " << area(4, 7) << endl; cout << "三角形的面积: " << area(3, 6) << endl; }
By using function inlining, the efficiency of the program can be further improved:
#include <iostream> using namespace std; inline double area(int radius) { return 3.14 * radius * radius; } inline double area(int length, int width) { return length * width; } inline double area(int base, int height) { return 0.5 * base * height; } int main() { cout << "圆的面积: " << area(5) << endl; cout << "矩形的面积: " << area(4, 7) << endl; cout << "三角形的面积: " << area(3, 6) << endl; }
The above is the detailed content of C++ function overloading and function inlining. For more information, please follow other related articles on the PHP Chinese website!
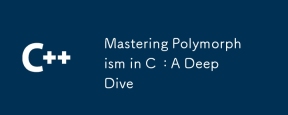
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
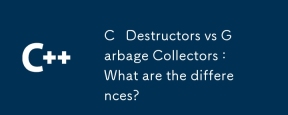
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
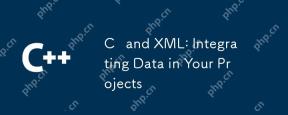
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
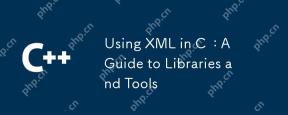
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
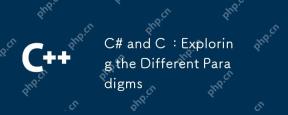
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
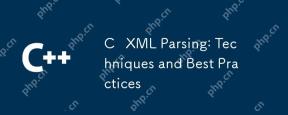
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
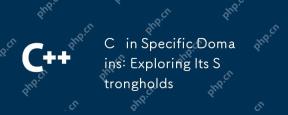
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
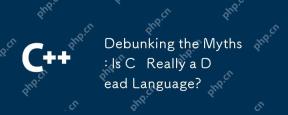
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
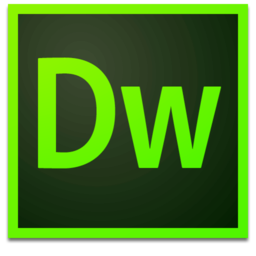
Dreamweaver Mac version
Visual web development tools
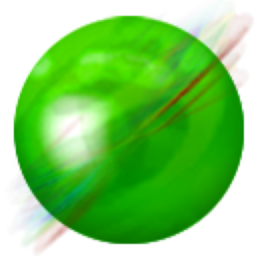
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
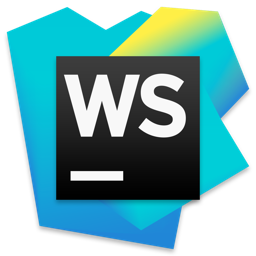
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
