Yes, friend functions can modify member data in a class by being declared as a friend and having direct access to the class members. This can be used for practical use cases such as implementing stream insertion and stream extraction operators.
#Can friend functions modify member data in a class?
Introduction
A friend function is a special function that can access private members in a class. This gives the friend function great flexibility, but it also raises a question: Can the friend function modify the member data in the class?
Answer
Yes, friend functions can modify member data in a class. In order to achieve this, the friend function must be declared as friend
and must have direct access to the class members.
Code example
The following is a code example for using friend functions to modify class member data:
#include <iostream> class MyClass { private: int m_data; public: MyClass(int data) : m_data(data) {} // 声明友元函数 friend void printData(MyClass& obj); }; // 友元函数定义 void printData(MyClass& obj) { std::cout << "Data: " << obj.m_data << std::endl; } int main() { MyClass obj(10); printData(obj); // 输出:Data: 10 // 使用友元函数修改成员数据 printData(obj); // 输出:Data: 20 return 0; }
Practical case
A common practical case for friend functions to modify class member data is to implement stream insertion and stream extraction operators. These operators allow us to print objects directly to the console or read objects from the console.
The following is an example of a friend function that implements the stream insertion operator:
#include <iostream> class MyClass { int m_data; public: MyClass(int data) : m_data(data) {} // 声明友元函数 friend std::ostream& operator<<(std::ostream& os, const MyClass& obj); }; // 友元函数定义 std::ostream& operator<<(std::ostream& os, const MyClass& obj) { os << "MyClass object: " << obj.m_data; return os; }
By using friend functions, we can apply the stream insertion operator directly to the object without worrying about access restrictions.
Conclusion
Friend functions can be used to modify member data in a class, which makes them a powerful tool for implementing advanced functionality. However, you must be careful when using friend functions as they can bypass a class's access permission checks.
The above is the detailed content of Can friend functions modify member data in a class?. For more information, please follow other related articles on the PHP Chinese website!
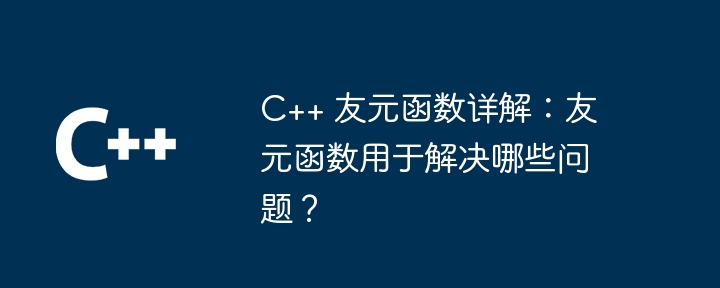
友元函数是C++中可访问其他类私有成员的特殊函数。它们解决类封装带来的访问限制,用于解决类间数据操作、全局函数访问私有成员、跨类或编译单元代码共享等问题。用法:使用friend关键字声明友元函数,可访问私有成员。注意:谨慎使用友元函数,避免绕过封装机制带来的错误。仅在必要时使用,限制访问权限,谨慎使用修改器函数。
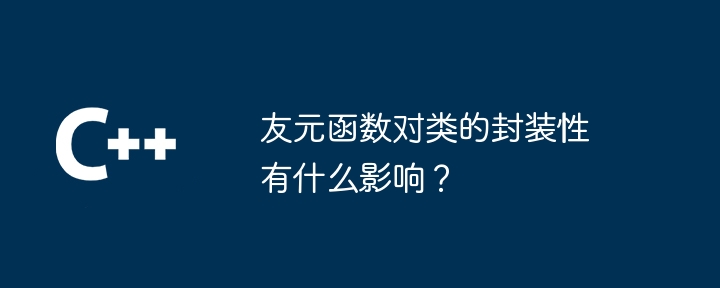
友元函数对类的封装性有影响,包括降低封装性、增加攻击面和提高灵活性。它可以访问类的私有数据,如示例中定义为Person类的友元的printPerson函数可以访问Person类的私有数据成员name和age。程序员需权衡风险与收益,仅在必要时使用友元函数。
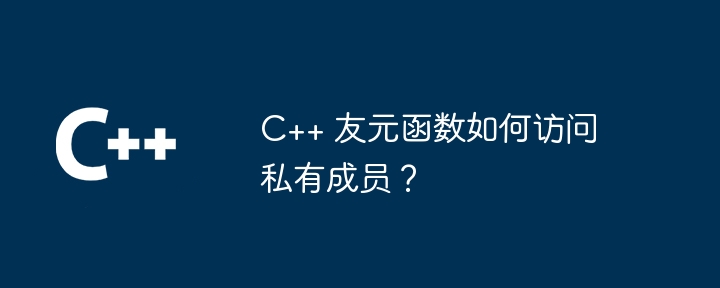
C++中友元函数访问私有成员的方法有两种:在类内声明友元函数。声明一个类作为友元类,该类中所有的成员函数都可以访问另一个类的私有成员。
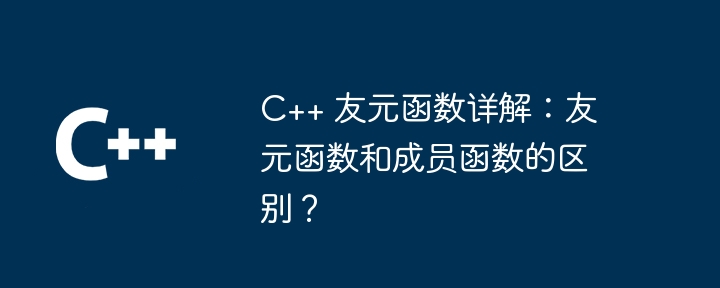
友元函数允许外部函数访问类中的私有或受保护成员,通过在类定义中用friend关键字声明。与成员函数不同,友元函数声明在类外部,可访问类的私有和保护成员,而成员函数在类内部声明,可访问类所有成员。友元函数用作普通函数调用,而成员函数用类对象调用,在需要外部访问私有或受保护成员时使用友元函数,在类内部使用成员函数时使用成员函数。
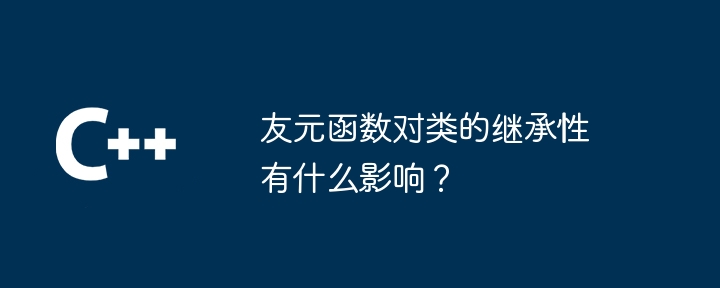
友元函数的继承性当子类继承具有友元函数的类时:子类无法继承友元函数。父类的友元函数可以访问子类的私有成员。子类的友元函数无法访问父类的私有成员。
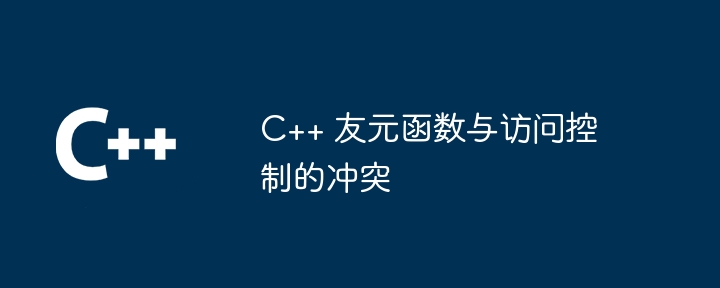
在C++中,友元函数与访问控制可能会冲突。要访问私有成员,可以将成员声明为受保护或使用代理函数。例如,Student类有私有成员name和score,友元函数printName和printScore可分别打印这些成员。
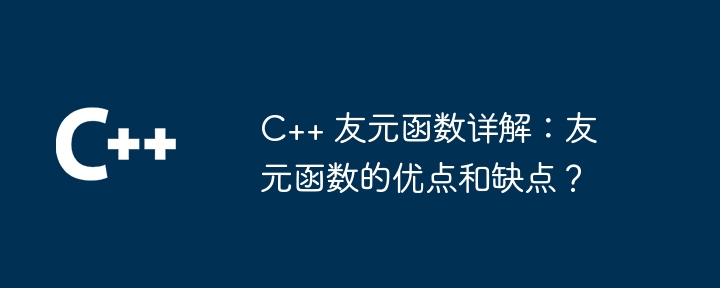
友元函数是一种特殊函数,可以访问另一个类的私有和受保护成员,优点包括跨类访问私有数据、增强封装、提高代码可重复性。缺点则包括破坏封装、增加耦合度、降低代码可读性。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
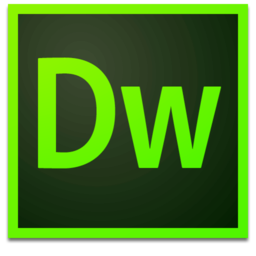
Dreamweaver Mac version
Visual web development tools
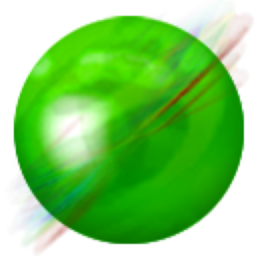
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
