In Go, the dependency injection (DI) pattern is implemented through function parameter passing, including value passing and pointer passing. In the DI pattern, dependencies are typically passed as pointers to improve decoupling, reduce lock contention, and support testability. By using pointers, the function is decoupled from the concrete implementation because it only depends on the interface type. Pointer passing also reduces the overhead of passing large objects, thereby reducing lock contention. Additionally, DI pattern makes it easy to write unit tests for functions that use DI pattern because dependencies can be easily mocked.
Function parameter passing dependency injection mode in Go language
Introduction
Dependency injection (DI) is a design pattern that allows an object to obtain its dependencies in a decoupled manner. In Go, DI is usually implemented through function parameter passing.
Types of parameter passing
There are two types of function parameter passing in Go:
- Value passing: The parameter variable is a copy of the original value, and any changes to the parameter variable will not affect the original value.
- Pointer passing: The parameter variable is a pointer to the original value, and changes to the parameter variable will also affect the original value.
Parameter passing in DI mode
In DI mode, dependencies are usually passed as pointers. The benefits of doing this are as follows:
- Improve decoupling: By using pointers, the function is decoupled from the specific implementation because it only depends on the interface type.
- Reduce lock contention: Passing pointers can reduce the overhead of passing large objects, thereby reducing lock contention.
- Support testability: It is easier to write unit tests for functions that use the DI pattern because dependencies can be easily mocked.
Practical case
Consider a UserService, which needs to access the User Repository:
type UserService struct { userRepository UserRepository } func (s *UserService) CreateUser(user *User) error { return s.userRepository.Create(user) }
We can use the DI pattern to provide a UserRepository instance for the UserService :
func main() { // 创建 UserRepository 实例 userRepository := NewUserRepository() // 创建 UserService 实例并注入 UserRepository userService := UserService{ userRepository: userRepository, } // 使用 UserService user := &User{Name: "John"} err := userService.CreateUser(user) if err != nil { // 处理错误 } }
By using a pointer to pass UserRepository, UserService is decoupled from the specific implementation of UserRepository. We can easily create different implementations for UserRepository and inject them into UserService.
Conclusion
The DI pattern in function argument passing is a powerful and flexible technique in Go for managing dependencies between objects. It improves decoupling, reduces lock contention, and supports testability.
The above is the detailed content of Dependency injection pattern in Golang function parameter passing. For more information, please follow other related articles on the PHP Chinese website!
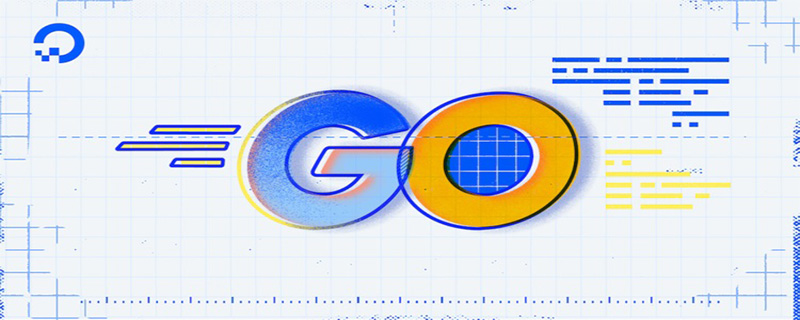
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
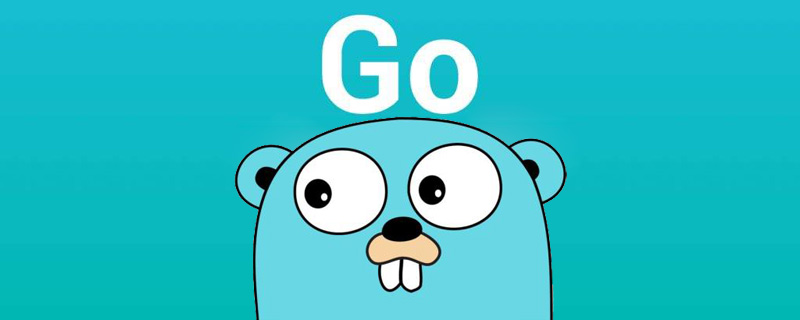
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
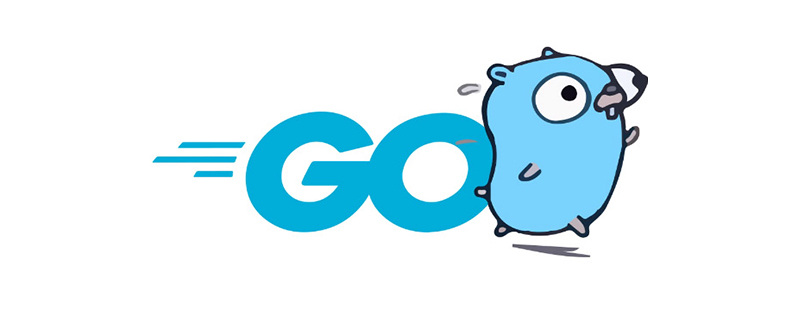
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
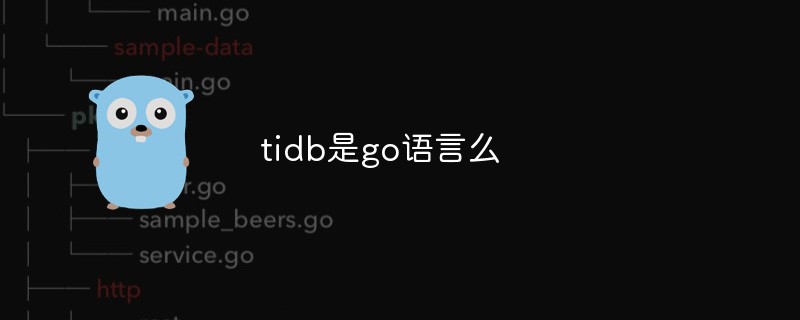
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
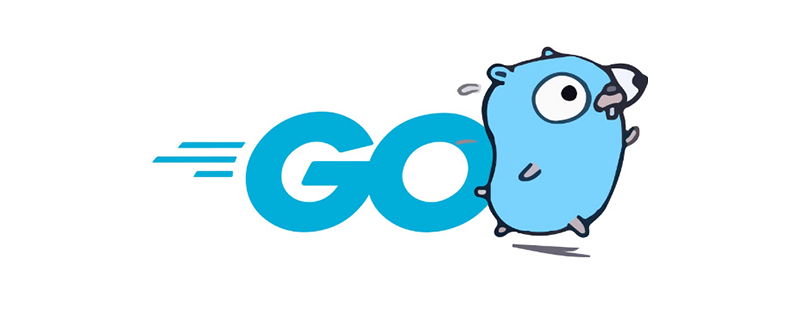
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
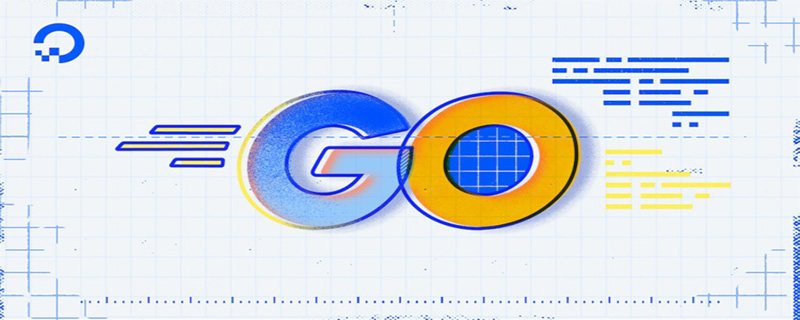
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
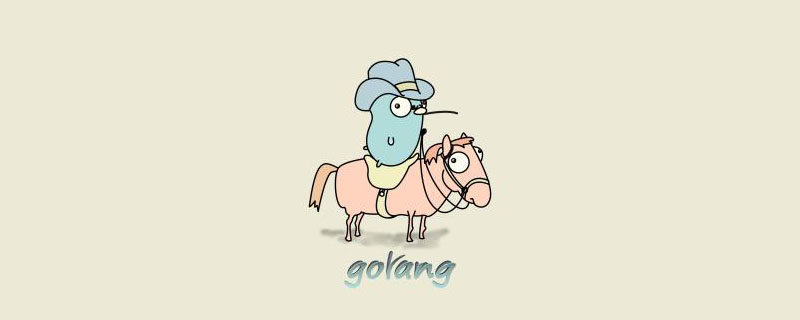
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
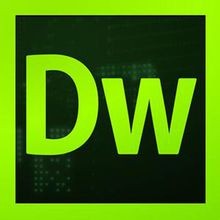
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
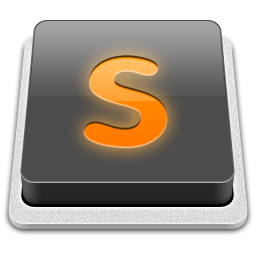
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
