The role of C++ function return value types in polymorphism
In polymorphism, the function return value type specifies the specific object type returned when a derived class overrides a base class method. The return value type of a derived class method can be the same as the base class or more specific, allowing more derived types to be returned, thereby increasing flexibility.
The role of C function return value type in polymorphism
Introduction
Polymorphism is an important feature in object-oriented programming, which allows parent class references to point to objects of its subclasses. In polymorphism, the function return value type plays a key role, which determines the specific object type returned when a derived class overrides a base class method.
Polymorphism and return value types
When a derived class inherits a base class, the derived class can override the methods of the base class. If a base class method has a return value, the derived class method must have the same or more specific return value type as the base class method.
- Same return value type: Derived class methods return the same type as base class methods. This is the most common situation.
- More specific return value types: Derived class methods return more specific types than base class methods. This allows derived class methods to return more derived types, thus increasing flexibility.
For example, consider the following base and derived classes:
class Shape { public: virtual Shape* clone() = 0; }; class Circle : public Shape { public: virtual Circle* clone() override; };
Shape
The base class defines a clone
method that returns a Shape
Object. Derived class Circle
overrides the clone
method and returns a more specific Circle
object.
Practical Case
The following is a practical case that shows the role of C function return value type in polymorphism:
#include <iostream> class Animal { public: virtual std::string speak() = 0; }; class Dog : public Animal { public: std::string speak() override { return "Woof!"; } }; class Cat : public Animal { public: std::string speak() override { return "Meow!"; } }; int main() { Animal* animal = new Dog; std::cout << animal->speak() << std::endl; // 输出: Woof! animal = new Cat; std::cout << animal->speak() << std::endl; // 输出: Meow! return 0; }
In this example , the Animal
base class defines a speak
method, which returns a string representing an animal's sound. Derived classes Dog
and Cat
override the speak
method and return the specific bark string.
The main function creates a Animal
pointer and points to the above derived class object. Due to the polymorphic nature, the program can call the speak
method of the derived class and get the correct scream output.
The above is the detailed content of The role of C++ function return value types in polymorphism. For more information, please follow other related articles on the PHP Chinese website!
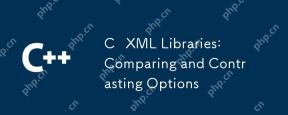
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
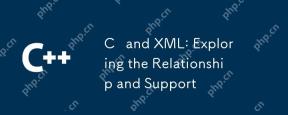
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
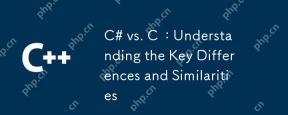
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
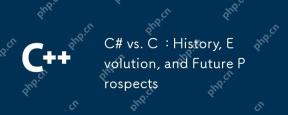
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
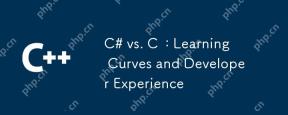
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
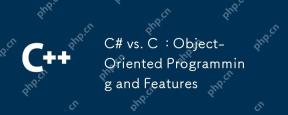
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
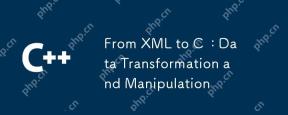
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
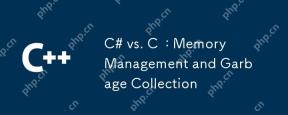
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
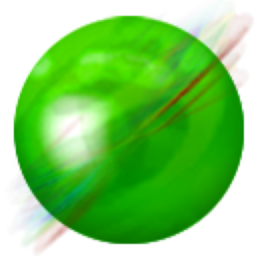
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
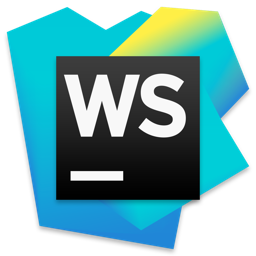
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor