


The best application scenarios of functional programming in Go are: Concurrency and parallel computing: FP immutable data and side-effect-free functions ensure that concurrent tasks do not interfere with each other. Event handling: FP focuses on immutability and is suitable for handling events without worrying about side effects. Data transformations and pipelines: Go's first-class functions allow easy writing and composition of data pipelines to transform and process data. Testing: Immutable data and side-effect-free functions make FP code easier to test because the functions do not change the data.
The best application scenarios of functional programming in Go
Functional programming (FP) is the use of mathematics in programming A paradigmma of the function concept that emphasizes immutability and no side effects. The Go language supports FP through its powerful concurrency features and first-class functions, making it well-suited for certain application scenarios.
Best application scenarios:
Concurrency and parallel computing
Go’s concurrency model is a natural fit for FP. Immutable data and side-effect-free functions ensure that concurrent tasks do not interfere with each other, simplifying reasoning and implementation of parallel computing.
Event processing
The focus of FP is immutability, which is very suitable for event processing systems. Events can be handled without worrying about side effects, making code easier to reason about and debug.
Data transformations and pipes
Functional pipes allow data to be transformed from one form to another through a chain of functions. Go's first-class functions and anonymous functions make it easy to write and compose these pipelines to create powerful data processing systems.
Testing
Immutable data and side-effect-free functions make FP code easier to test. Because the function does not change the data passed to it, the test can run independently without affecting other parts.
Practical Case: Concurrent Web Service
Consider a concurrent Web service that needs to handle requests from multiple clients. The following code shows how to implement this service using FP principles:
package main import ( "fmt" "log" "net/http" ) type Request struct { Data string } type Response struct { Code int Body string } // 处理函数(纯函数,可并发执行) func handleRequest(r Request) Response { log.Printf("Handling request with data: %s", r.Data) return Response{Code: 200, Body: fmt.Sprintf("Processed data: %s", r.Data)} } func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { // 处理请求 request := Request{Data: r.FormValue("data")} response := handleRequest(request) // 响应请求 w.WriteHeader(response.Code) fmt.Fprintf(w, response.Body) }) log.Printf("Listening on port 8080") http.ListenAndServe(":8080", nil) }
In this example, the handleRequest
function is a pure function and does not modify the data passed to it. Therefore, it can be executed safely in a concurrent environment, where multiple Goroutines can handle requests simultaneously without worrying about data races.
The above is the detailed content of What are the best application scenarios of functional programming in Golang?. For more information, please follow other related articles on the PHP Chinese website!
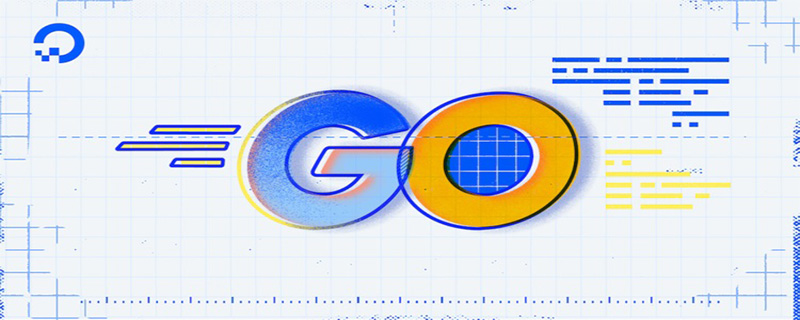
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
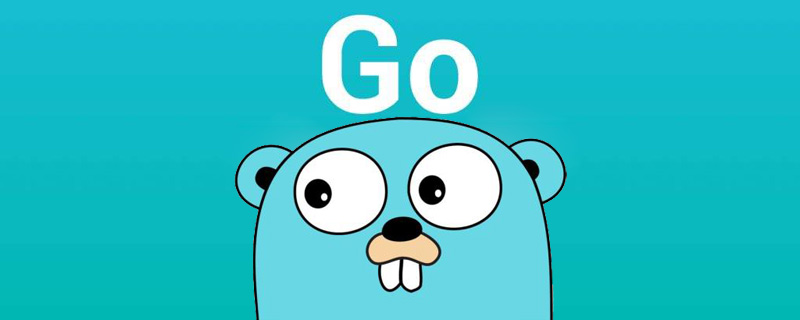
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
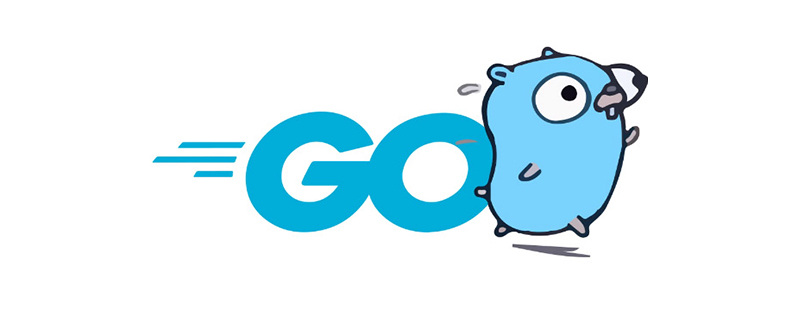
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
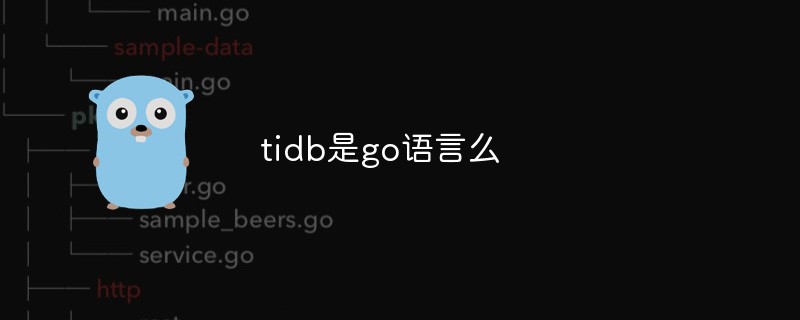
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
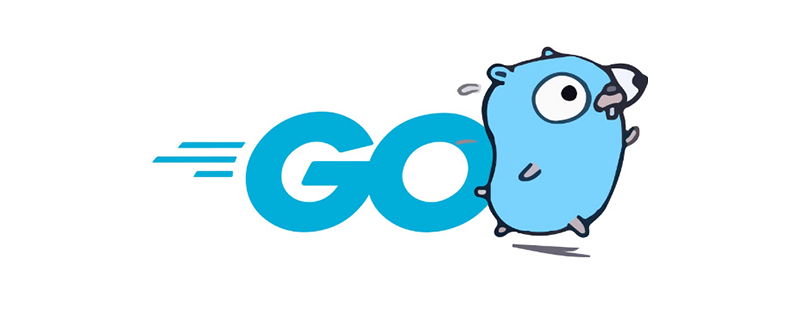
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
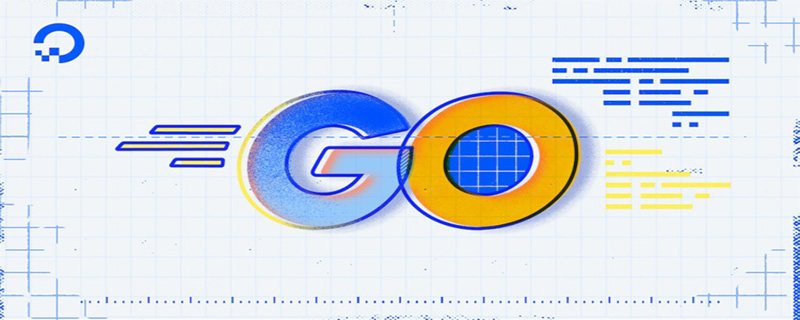
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
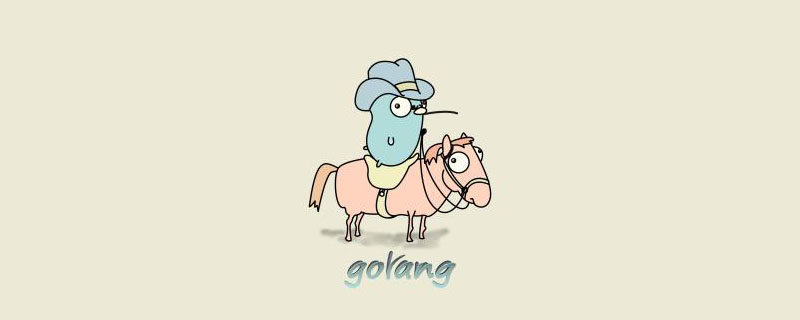
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
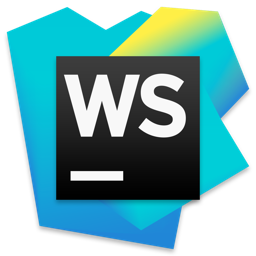
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
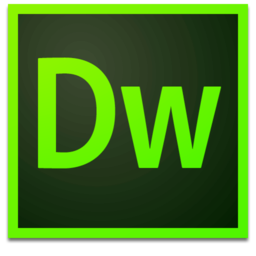
Dreamweaver Mac version
Visual web development tools