Java I/O streams implement asynchronous I/O operations by using classes provided by the java.nio package, including AsynchronousChannel and CompletionHandler. These classes provide methods for initiating asynchronous read (readAsync) and write (writeAsync) operations. This mechanism improves I/O performance by allowing applications to continue executing without waiting for I/O operations to complete.
How Java I/O streams implement asynchronous I/O operations
Introduction
In Java, Asynchronous I/O operations allow an application to continue executing while waiting for an I/O operation (such as reading or writing a file) to complete. This article explores how Java I/O streams implement asynchronous I/O operations.
Java NIO
Asynchronous I/O is implemented in Java through the java.nio
package. This package provides the following classes for asynchronous I/O:
-
AsynchronousChannel
: Represents a channel that supports asynchronous I/O operations. -
CompletionHandler
: Callback interface for handling completed asynchronous I/O operations.
Asynchronous I/O stream
The Java I/O stream class provides methods to interact with AsynchronousChannel
to support asynchronous I/O /O operations. These methods include:
-
readAsync
: Initiates an asynchronous read operation. -
writeAsync
: Initiate an asynchronous write operation.
Practical case
The following is an asynchronous I/O operation using the readAsync
and writeAsync
methods Example:
import java.io.FileInputStream; import java.io.FileOutputStream; import java.nio.ByteBuffer; import java.nio.channels.AsynchronousFileChannel; import java.nio.channels.CompletionHandler; public class AsyncIOExample { public static void main(String[] args) throws Exception { // 创建 AsynchronousFileChannel AsynchronousFileChannel inChannel = AsynchronousFileChannel.open(new FileInputStream("input.txt")); AsynchronousFileChannel outChannel = AsynchronousFileChannel.open(new FileOutputStream("output.txt")); // 创建缓冲区 ByteBuffer buffer = ByteBuffer.allocate(1024); // 发起异步读取操作 inChannel.read(buffer, 0, null, new CompletionHandler<Integer, Void>() { @Override public void completed(Integer result, Void attachment) { // 读取数据成功 // ... // 发起异步写入操作 outChannel.write(buffer, 0, null, new CompletionHandler<Integer, Void>() { @Override public void completed(Integer result, Void attachment) { // 写入数据成功 // ... } @Override public void failed(Throwable exc, Void attachment) { // 写入数据失败 // ... } }); } @Override public void failed(Throwable exc, Void attachment) { // 读取数据失败 // ... } }); } }
Conclusion
By using the java.nio
package, Java I/O streams can implement asynchronous I/O operations. This enables applications to improve I/O performance, thereby improving overall performance.
The above is the detailed content of How does Java I/O stream implement asynchronous I/O operations?. For more information, please follow other related articles on the PHP Chinese website!
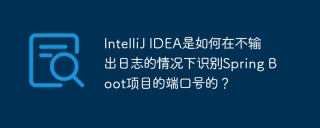
Start Spring using IntelliJIDEAUltimate version...
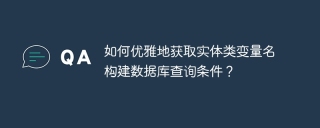
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
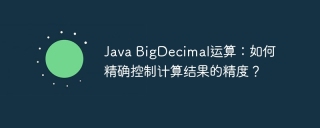
Java...
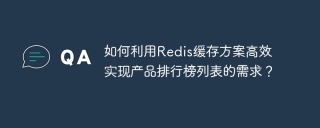
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
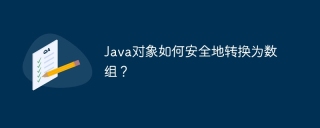
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
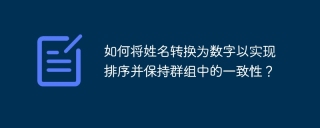
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
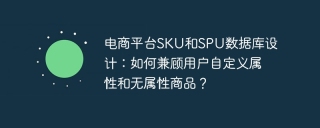
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
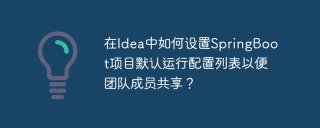
How to set the SpringBoot project default run configuration list in Idea using IntelliJ...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
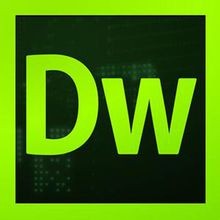
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor