When passing function parameters in Go, slices and maps pass references instead of values. Modification of the slice in the function will affect the slice in the calling function. Mapping modifications in a function will also affect the mapping in the calling function. If you need to pass a copy, you can use the copy function. When multiple goroutines access slices or maps at the same time, data competition should be considered and mutexes should be used to synchronize access.
Slicing and mapping in function parameter passing in Go
In Go, function parameters can be value types or reference types . Value types are copied when parameters are passed, while reference types are passed by reference.
Slice
Slice is a reference type, so its reference will be passed when the function parameter is passed. This means that any changes made to the slice elements in the function will be reflected in the function that calls it.
Example:
func modifySlice(slice []int) { slice[0] = 100 // 修改切片元素 } func main() { slice := []int{1, 2, 3} modifySlice(slice) // 传递切片引用 fmt.Println(slice) // 输出:[100 2 3] }
Mapping
Mapping is also a reference type, and its reference is also passed when function parameters are passed. Similar to slicing, any changes made to the map in a function will be reflected in the function that calls it.
Example:
func modifyMap(m map[string]int) { m["key"] = 100 // 修改映射元素 } func main() { m := make(map[string]int) m["key"] = 1 modifyMap(m) // 传递映射引用 fmt.Println(m["key"]) // 输出:100 }
Notes
-
Passing a copy of the slice or map: Sometimes it is more appropriate to pass a copy of a slice or map rather than a reference. A copy can be created using the
copy
function. -
Prevent data race: When multiple goroutines access the same slice or map at the same time, data race may occur. To prevent this, you can use a mutex lock (
sync.Mutex
) to synchronize access to the slice or map.
The above is the detailed content of Processing of slicing and mapping in Golang function parameter passing. For more information, please follow other related articles on the PHP Chinese website!
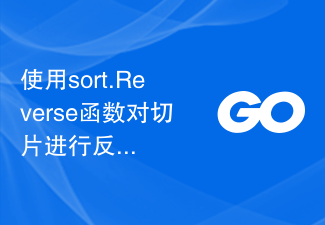
使用sort.Reverse函数对切片进行反转排序在Go语言中,切片是一个重要的数据结构,它可以动态地增加或减少元素数量。当我们需要对切片进行排序时,可以使用sort包提供的函数进行排序操作。其中,sort.Reverse函数可以帮助我们对切片进行反转排序。sort.Reverse函数是sort包中的一个函数,它接受一个sort.Interface接口类型的
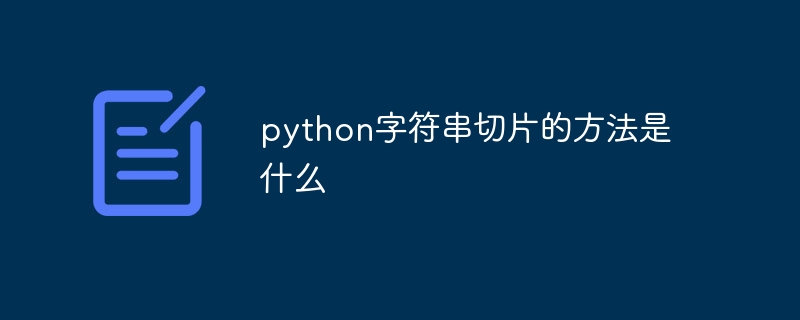
在Python中,可以使用字符串切片来获取字符串中的子串。字符串切片的基本语法为“substring = string[start:end:step]”。
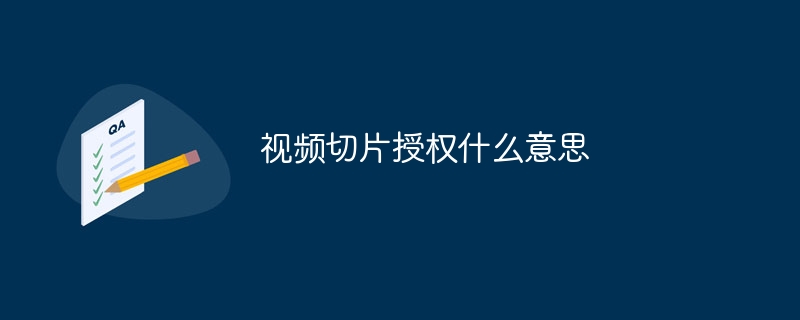
视频切片授权是指在视频服务中,将视频文件分割成多个小片段并进行授权的过程。这种授权方式能提供更好的视频流畅性、适应不同网络条件和设备,并保护视频内容的安全性。通过视频切片授权,用户可以更快地开始播放视频,减少等待和缓冲时间,视频切片授权可以根据网络条件和设备类型动态调整视频参数,提供最佳的播放效果,视频切片授权还有助于保护视频内容的安全性,防止未经授权的用户进行盗播和侵权行为。
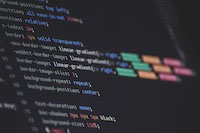
什么是JPA?它与JDBC有什么区别?JPA(JavaPersistenceapi)是一个用于对象关系映射(ORM)的标准接口,它允许Java开发者使用熟悉的Java对象来操作数据库,而无需编写直接针对数据库的sql查询。而JDBC(JavaDatabaseConnectivity)是Java用于连接数据库的标准API,它需要开发者使用SQL语句来操作数据库。JPA将JDBC封装起来,为对象-关系映射提供了更方便、更高级别的API,简化了数据访问操作。在JPA中,什么是实体(Entity)?实体
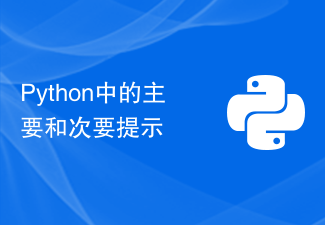
简介主要和次要提示,要求用户输入命令并与解释器进行通信,使得这种交互模式成为可能。主要提示通常由>>>表示,表示Python已准备好接收输入并执行相应的代码。了解这些提示的作用和功能对于发挥Python的交互式编程能力至关重要。在本文中,我们将讨论Python中的主要和次要提示符,强调它们的重要性以及它们如何增强交互式编程体验。我们将研究它们的功能、格式选择以及在快速代码创建、实验和测试方面的优势。开发人员可以通过理解主要和次要提示符来使用Python的交互模式,从而改善他们的
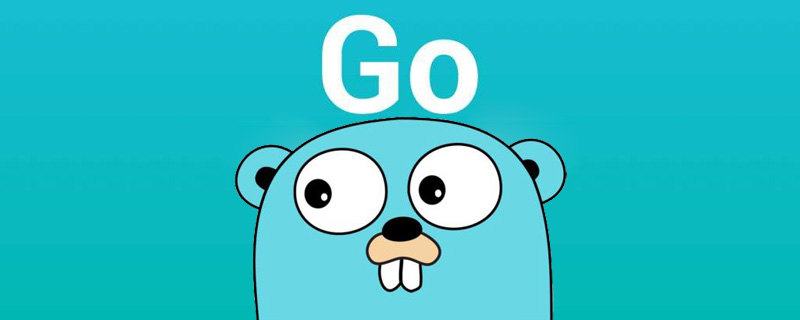
修改方法:1、使用append()函数添加新值,语法“append(切片,值列表)”;2、使用append()函数删除元素,语法“append(a[:i], a[i+N:]...)”;3、直接根据索引重新赋值,语法“切片名[索引] = 新值”。
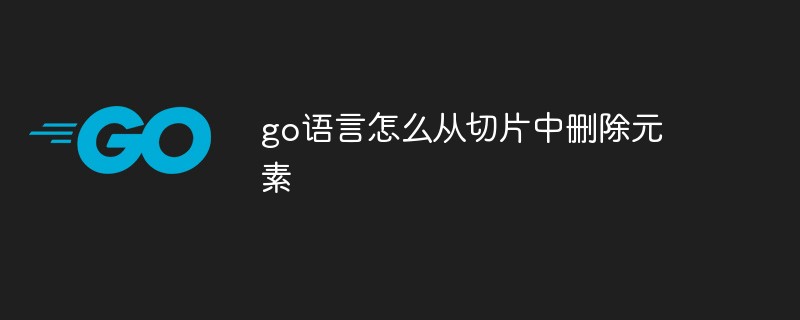
删除方法:1、对切片进行截取来删除指定元素,语法“append(a[:i], a[i+1:]...)”。2、创建一个新切片,将要删除的元素过滤掉后赋值给新切片。3、利用一个下标index,记录下一个有效元素应该在的位置;遍历所有元素,当遇到有效元素,将其移动到 index且index加一;最终index的位置就是所有有效元素的下一个位置,最后做一个截取即可。
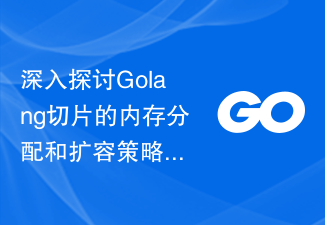
Golang切片原理深入剖析:内存分配与扩容策略引言:切片是Golang中常用的数据类型之一,它提供了便捷的方式来操作连续的数据序列。在使用切片的过程中,了解其内部的内存分配与扩容策略对于提高程序的性能十分重要。在本文中,我们将深入剖析Golang切片的原理,并配以具体的代码示例。一、切片的内存结构和基本原理在Golang中,切片是对底层数组的一种引用类型,


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
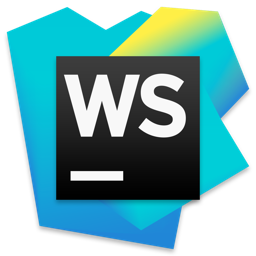
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
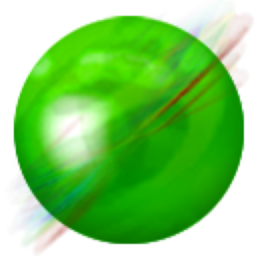
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
