


Go function return values play a key role in concurrent programming: making it possible to exchange data between goroutines through communication channels. Allows functions to return the results of concurrent tasks so that the main program can process the results. Control the flow of concurrent execution, such as waiting for a goroutine to complete or collecting results.
The role of Go function return value in concurrent programming
In Go concurrent programming, function return value plays an important role in managing parallel tasks and plays a vital role in handling concurrency.
How to use function return values for concurrent processing
Go functions can be executed concurrently through goroutine
. goroutine
is a lightweight concurrent execution unit that allows multiple tasks to run at the same time without blocking the main program.
// startGoroutine 启动一个 goroutine func startGoroutine(task func()) { go task() }
To use function return values for concurrent processing, you need to use Goroutine communication channels (channels). Channels allow safe and efficient data exchange between goroutines.
// createChannel 创建一个 channel func createChannel() chan int { return make(chan int) } // goroutineTask 在 goroutine 中执行任务并返回结果 func goroutineTask(ch chan int, task func() int) { ch <- task() }
Practical Case: Calculating the Fibonacci Sequence
Consider an example for calculating the Fibonacci Sequence:
// fibonacci 计算斐波那契数列 func fibonacci(n int) int { if n == 0 || n == 1 { return 1 } ch1 := createChannel() ch2 := createChannel() startGoroutine(func() { goroutineTask(ch1, func() int { return fibonacci(n - 1) }) }) startGoroutine(func() { goroutineTask(ch2, func() int { return fibonacci(n - 2) }) }) return <-ch1 + <-ch2 }
In this In the example, the fibonacci
function uses a recursive method to calculate the Fibonacci sequence. It starts two goroutines, each goroutine computes a subproblem and returns the result through a channel. The main program waits for both goroutines to complete and returns the sum.
Function return values are crucial in concurrent programming for:
- Enable communication between goroutines
- Process the results of concurrent tasks
- Control concurrent execution flow
The above is the detailed content of What is the role of Golang function return value in concurrent programming?. For more information, please follow other related articles on the PHP Chinese website!

提到线程,你的大脑应该有这样的印象:我们可以控制它何时开始,却无法控制它何时结束,那么如何获取线程的返回值呢?今天就分享一下自己的一些做法。方法一:使用全局变量的列表,来保存返回值ret_values = [] def thread_func(*args): ... value = ... ret_values.append(value)选择列表的一个原因是:列表的 append() 方法是线程安全的,CPython 中,GIL 防止对它们的并发访问。如果你使用自定义的数据结构,在并
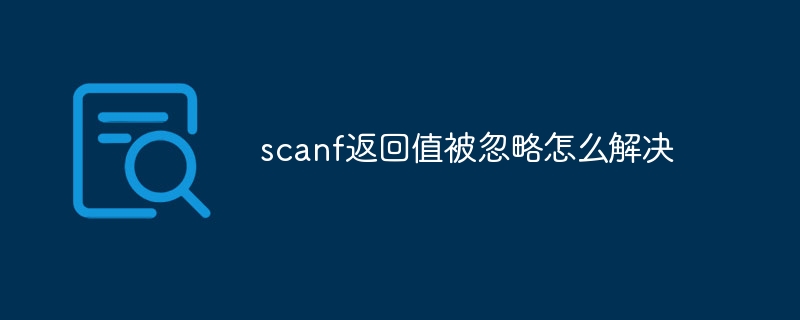
scanf返回值被忽略的解决办法有检查scanf的返回值、清除输入缓冲区和使用fgets替代scanf等。详细介绍:1、检查scanf的返回值,应该始终检查scanf函数的返回值,scanf函数的返回值是成功读取的参数的数量,如果返回值与期望的不一致,就意味着输入有误;2、清除输入缓冲区,在使用scanf函数时,如果输入的数据与期望的格式不匹配,将导致输入缓冲区中的数据等等。
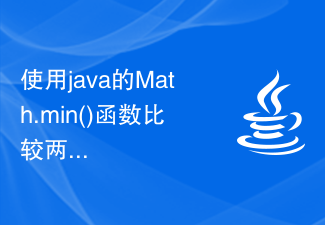
使用Java的Math.min()函数比较两个数值的大小并返回较小值在开发Java应用程序时,有时我们需要比较两个数值的大小,并返回较小的那个数。Java提供了Math.min()函数来实现这个功能。Math.min()函数是JavaMath类的一个静态方法,它用于比较两个数值的大小并返回较小的那个数。它的语法如下:publicstaticintmi
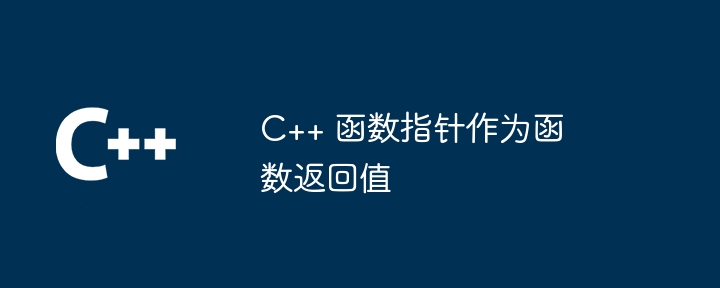
函数指针可以作为函数返回值,允许我们在运行时确定要调用的函数。语法为:returntype(*function_name)(param1,param2,...)。优点包括动态绑定和回调机制,使我们可以根据需要调整函数调用。
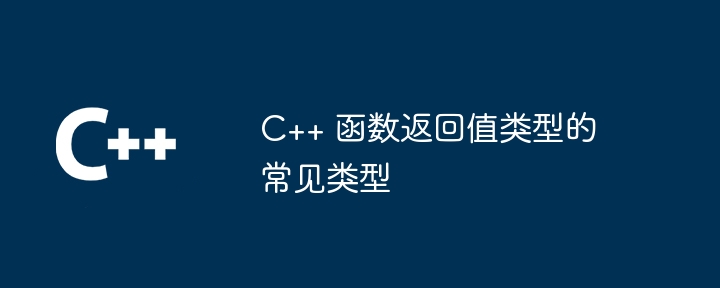
C++函数返回类型包括:void(无返回值)、基本类型(整数、浮点数、字符和布尔值)、指针、引用、类和结构。选择时,应考虑功能、效率和接口。如计算阶乘的factorial函数,返回整数类型以满足功能需求和避免额外操作。
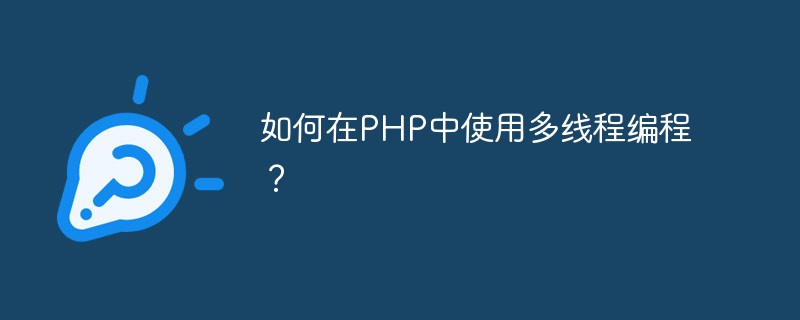
随着Web应用程序变得越来越庞大和复杂,传统的单线程PHP开发模式不再适用于高并发处理。在这种情况下,使用多线程技术可以提高Web应用程序处理并发请求的能力。本文将介绍如何在PHP中使用多线程编程。一、多线程概述多线程编程是指在一个进程中并发执行多个线程,每个线程都能单独访问进程中的共享内存和资源。多线程技术可以提高CPU和内存的使用效率,同时可以处理更多的
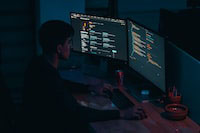
这篇文章将为大家详细讲解有关PHP返回数组内部指针当前指向的键名,小编觉得挺实用的,因此分享给大家做个参考,希望大家阅读完这篇文章后可以有所收获。PHP返回数组内部指针当前指向的键名php提供了一个叫做key()的函数,用于返回数组内部指针当前指向的键名。此函数适用于索引数组和关联数组。语法key(array)参数array:要从中获取键名的数组。返回值内部指针当前指向的键名,如果是索引数组,则返回整数索引;如果是关联数组,则返回字符串键名。如果数组为空或者内部指针指向数组末尾,则返回NULL。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
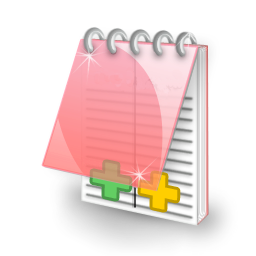
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
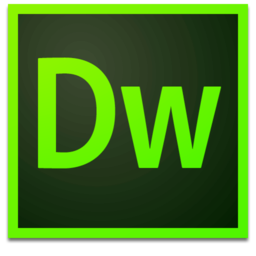
Dreamweaver Mac version
Visual web development tools
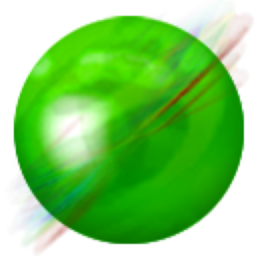
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
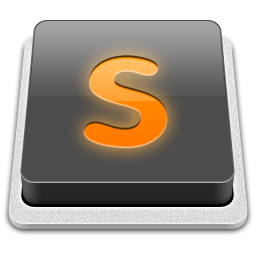
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
