How to optimize Java exception handling performance?
Best practices for optimizing Java exception handling performance include throwing exceptions only when necessary. Use specific exception classes. Cache exception messages. Consider using a try-with-resources statement.
How to optimize Java exception handling performance
Exception handling is an essential part of Java, but if not used correctly, it may have a negative impact on the application Performance is negatively affected. You can optimize exception handling performance by adopting the following best practices:
Throw exceptions only when necessary
Throw only when a real error occurs or a condition where the problem must be reported to the caller abnormal. Avoid throwing unnecessary exceptions, as this increases the overhead of creating and throwing exceptions.
Use specific exception classes
Do not use the generic Exception
class, but create specific classes based on exception conditions. This will enable the caller to take appropriate action based on the exception type. Additionally, it allows the Java Virtual Machine (JVM) to recognize and handle exceptions faster.
Cache Exception Messages
Exception messages are often generated dynamically, which may negatively impact performance. This can be mitigated by caching these messages ahead of time and retrieving them when needed.
Consider using the try-with-resources
statement
try-with-resources
The statement can automatically close resources (such as files or database connections), This eliminates the possibility of forgetting to close these resources. Shutting down resources can be an expensive operation, so automating this process can improve performance.
Practical Case
The following example demonstrates how to optimize Java exception handling performance:
// 使用特定异常类 class MyException extends Exception { public MyException(String message) { super(message); } } // 缓存异常消息 Map<String, String> exceptionMessages = new HashMap<>(); // 使用 try-with-resources 语句关闭资源 try (BufferedReader reader = new BufferedReader(new FileReader("test.txt"))) { // ... } catch (IOException e) { // 处理异常 }
By following these best practices, you can optimize exception handling performance in Java applications, Improve the overall performance and responsiveness of your application.
The above is the detailed content of How to optimize Java exception handling performance?. For more information, please follow other related articles on the PHP Chinese website!
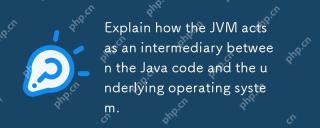
JVM works by converting Java code into machine code and managing resources. 1) Class loading: Load the .class file into memory. 2) Runtime data area: manage memory area. 3) Execution engine: interpret or compile execution bytecode. 4) Local method interface: interact with the operating system through JNI.

JVM enables Java to run across platforms. 1) JVM loads, validates and executes bytecode. 2) JVM's work includes class loading, bytecode verification, interpretation execution and memory management. 3) JVM supports advanced features such as dynamic class loading and reflection.

Java applications can run on different operating systems through the following steps: 1) Use File or Paths class to process file paths; 2) Set and obtain environment variables through System.getenv(); 3) Use Maven or Gradle to manage dependencies and test. Java's cross-platform capabilities rely on the JVM's abstraction layer, but still require manual handling of certain operating system-specific features.
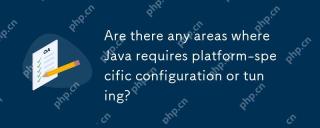
Java requires specific configuration and tuning on different platforms. 1) Adjust JVM parameters, such as -Xms and -Xmx to set the heap size. 2) Choose the appropriate garbage collection strategy, such as ParallelGC or G1GC. 3) Configure the Native library to adapt to different platforms. These measures can enable Java applications to perform best in various environments.
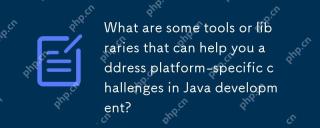
OSGi,ApacheCommonsLang,JNA,andJVMoptionsareeffectiveforhandlingplatform-specificchallengesinJava.1)OSGimanagesdependenciesandisolatescomponents.2)ApacheCommonsLangprovidesutilityfunctions.3)JNAallowscallingnativecode.4)JVMoptionstweakapplicationbehav
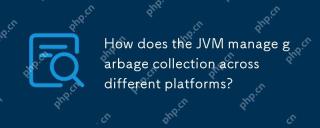
JVMmanagesgarbagecollectionacrossplatformseffectivelybyusingagenerationalapproachandadaptingtoOSandhardwaredifferences.ItemploysvariouscollectorslikeSerial,Parallel,CMS,andG1,eachsuitedfordifferentscenarios.Performancecanbetunedwithflagslike-XX:NewRa
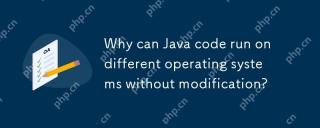
Java code can run on different operating systems without modification, because Java's "write once, run everywhere" philosophy is implemented by Java virtual machine (JVM). As the intermediary between the compiled Java bytecode and the operating system, the JVM translates the bytecode into specific machine instructions to ensure that the program can run independently on any platform with JVM installed.
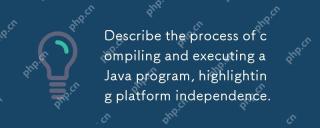
The compilation and execution of Java programs achieve platform independence through bytecode and JVM. 1) Write Java source code and compile it into bytecode. 2) Use JVM to execute bytecode on any platform to ensure the code runs across platforms.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
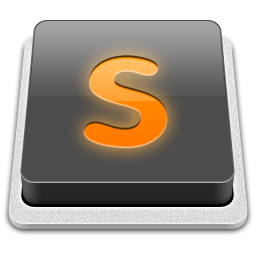
SublimeText3 Mac version
God-level code editing software (SublimeText3)
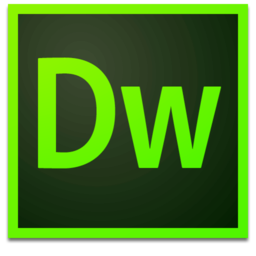
Dreamweaver Mac version
Visual web development tools
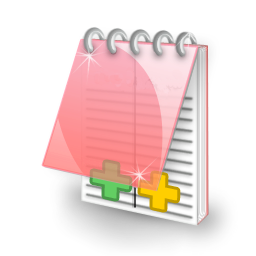
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
