C Function templates and generic programming allow the creation of generic code that accepts different types of data, achieving type independence through type parameters and template classes. Benefits include code reusability, type safety, and performance optimization. Function templates (like "print") and generic classes (like "Vector") allow you to write code that is type-free, efficient, and reusable.
C Function Templates and Generic Programming
Introduction
Function templates allow you to create Generic functions that accept different types of data. Generic programming extends this concept further, allowing you to write type-independent, efficient, and reusable code.
Function Template
Function template uses type parameters to represent placeholder data types. For example:
template<typename T> void print(T value) { std::cout << value << std::endl; }
This template function accepts a value of any data type T
and prints it to the console.
Generic programming
Generic programming separates type parameters from functions by using template classes. For example:
template<typename T> class Vector { public: T* data; int size; Vector() : data(nullptr), size(0) {} ~Vector() { delete[] data; } // ...其他方法... };
This Vector
class can store any type of data element.
Practical case
Sort vector
The following is an example of a generic sorting algorithm:
template<typename T> void sort(Vector<T>& v) { for (int i = 0; i < v.size - 1; i++) { for (int j = i + 1; j < v.size; j++) { if (v.data[j] < v.data[i]) { std::swap(v.data[i], v.data[j]); } } } }
This algorithm can be used for any A vector of type sort elements.
Benefits
- Code reusability: Function templates and generic programming allow you to write generic functions that can be used with multiple data types code.
- Type safety: The compiler enforces type consistency, thus preventing type errors.
- Performance optimization: Generic code uses inline technology for compile-time optimization to improve runtime speed.
With function templates and generic programming, you can write more flexible, robust, and efficient C code.
The above is the detailed content of C++ function templates and generic programming. For more information, please follow other related articles on the PHP Chinese website!
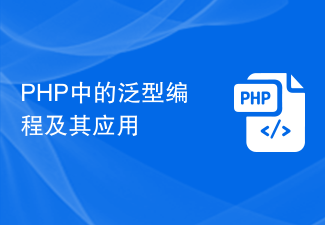
一、什么是泛型编程泛型编程是指在编程语言中实现一种通用的数据类型,使得这种数据类型能够适用于不同的数据类型,从而实现代码的复用和高效。PHP是一种动态类型语言,不像C++、Java等语言有强类型机制,因此在PHP中实现泛型编程不是一件容易的事情。二、PHP中的泛型编程方式PHP中有两种方式实现泛型编程:分别是使用接口和使用Trait。使用接口在PHP中创建一
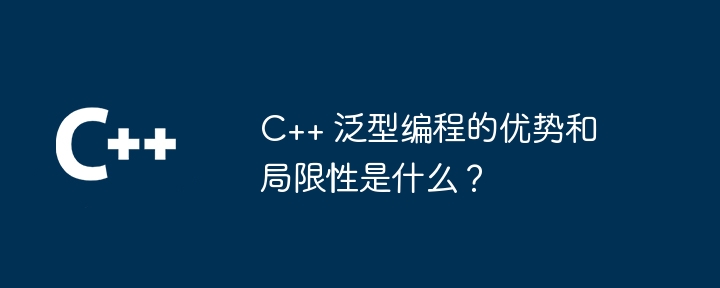
泛型编程是一种C++技术,具有如下优势:提高代码重用性,可处理多种数据类型。代码更简洁易读。在某些情况下可提高效率。但它也存在局限性:编译时需要更多时间。编译后代码会更大。可能产生运行时开销。
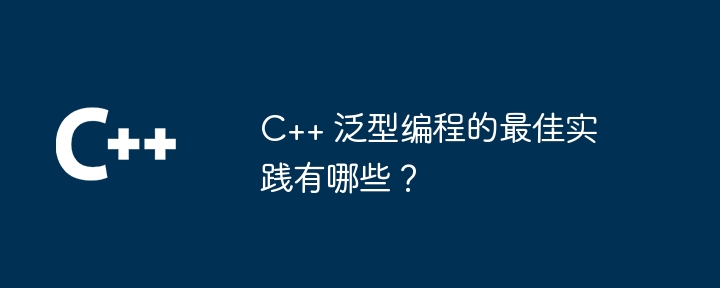
C++泛型编程的最佳实践包括:明确指定类型参数的类型要求。避免使用空类型参数。遵循Liskov替换原则,确保子类型与父类型具有相同的接口。限制模板参数的数量。谨慎使用特化。使用泛型算法和容器。使用命名空间组织代码。
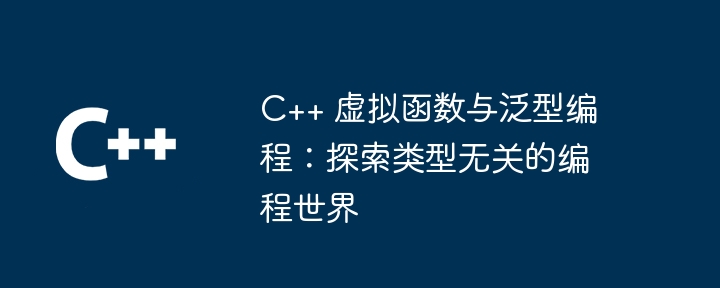
虚拟函数和泛型编程是C++中用于创建类型无关且可扩展代码的功能。虚拟函数允许派生类覆盖基类中的方法,从而实现多态行为。泛型编程涉及创建不受特定类型约束的算法和数据结构,使用类型参数来表示抽象类型。通过使用虚拟函数实现多态和使用泛型编程实现类型无关操作,开发者可以构建灵活且可维护的软件。
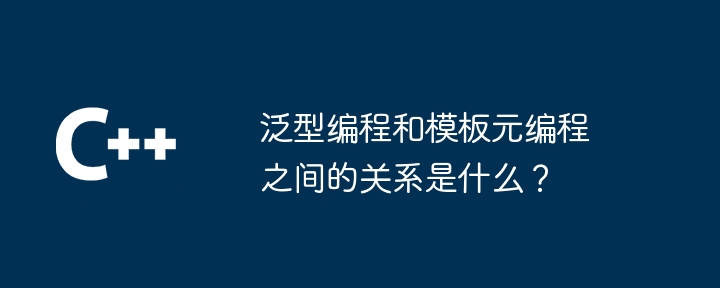
泛型编程和模板元编程在现代C++中是两个强有力的技术,分别用于在运行时处理不同类型的数据(泛型编程)和在编译时创建和计算代码(模板元编程)。尽管它们都基于模板,但它们在功能和使用上却有很大不同。在实践中,这两种技术经常一起使用,例如,可以将泛型代码与模板元编程结合来在运行时创建和实例化数据结构。
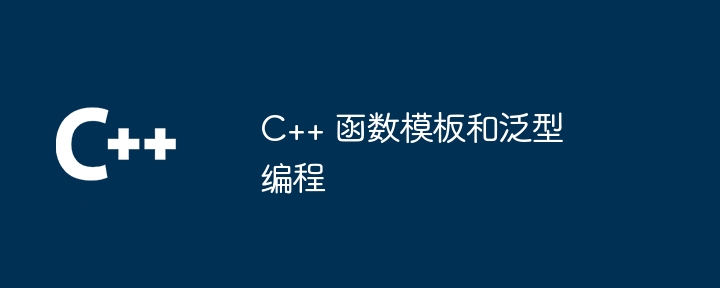
C++函数模板和泛型编程允许创建可接受不同类型数据的通用代码,通过类型参数和模板类实现类型无关性。优点包括代码可重用性、类型安全和性能优化。通过函数模板(如“print”)和泛型类(如“Vector”),您可以编写无类型依赖、高效且可重用的代码。
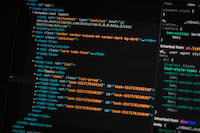
语法基础数据类型:Java提供了丰富的基本数据类型(如int、double和boolean)以及引用类型(如对象和数组)。变量:您使用变量来存储数据。它们由类型和名称标识,例如:intage=25;运算符:Java提供了各种运算符,用于进行算术、比较和逻辑运算。控制流:使用if-else、switch和for循环来控制程序执行流。对象和类类:Java中的对象是封装数据的实例。类是对象的模板,定义其状态和行为。对象:对象是类的一个实例,它包含根据类定义存储的数据。继承:子类可以继承父类的属性和方法
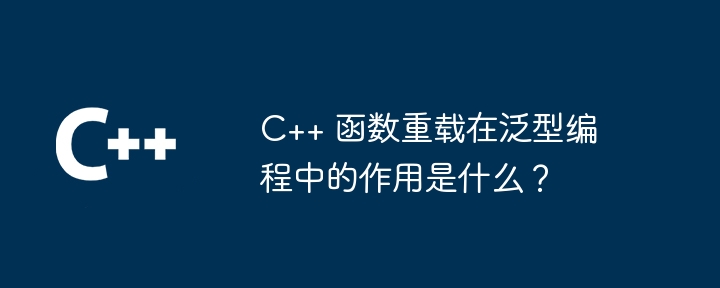
函数重载在泛型编程中,函数重载允许创建具有相同名称但不同参数类型的多个函数,以创建灵活、可重用的代码:语法:返回值类型函数名(参数类型1,参数类型2,...){...}应用:使用函数模板创建与数据类型无关的代码。提供特定类型的优化实现。优点:可重用性:为不同类型提供特定实现。灵活:处理各种数据类型。效率:提供优化实现,提高性能。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
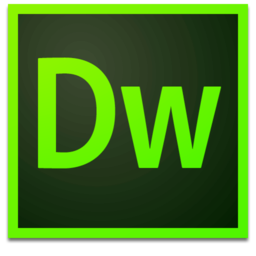
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
