For modern software, function security (input/output validation, secure data handling, exception handling) and permission management (authorization/authentication, RBAC, principle of least privilege) are crucial to prevent malicious input, achieve data protection, and Control access. Validating inputs (such as email addresses) and role-based access control (such as user permissions being restricted by roles) are real examples of implementing best practices.
Best Practices for Function Security and Permission Management
Introduction
In modern software development, security is of paramount importance. Function security and permission management are key aspects of ensuring application security. This article will explore the best practices for function security and permission management, and illustrate the application of these best practices through real-life cases.
Function Safety
- Input and Output Validation: Verify all function inputs and outputs to ensure they conform to expected scopes. This prevents malicious input from causing application crashes or data corruption.
- Secure Data Handling: Handle sensitive data such as passwords and personally identifiable information with care. Use appropriate encryption techniques and secure storage mechanisms to protect this data.
- Exception handling: Write robust exception handlers to deal with unexpected conditions. Avoid using exceptions for process control as this may lead to security vulnerabilities.
Practical case: verify input
def is_valid_email(email): """ 验证电子邮件地址是否有效。 参数: email: 要验证的电子邮件地址(字符串)。 返回: True 如果电子邮件有效,否则为 False。 """ import re # 定义电子邮件正则表达式模式 pattern = r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$" # 使用正则表达式验证电子邮件 return bool(re.match(pattern, email))
Permission management
- Authorization and authentication : Implement authorization and authentication mechanisms to control which users can access which resources. Use security credentials, such as tokens or keys, to authenticate users.
- Role-based access control (RBAC): Control access permissions based on the user's role. This enables you to customize access levels based on user responsibilities.
- Principle of least privilege: Grant users only the minimum permissions they need to perform their jobs. This helps limit potential harm.
Practical case: role-based access control
class User: def __init__(self, username, role): self.username = username self.role = role def has_permission(self, permission): return permission in self.role.permissions class Role: def __init__(self, name, permissions): self.name = name self.permissions = permissions # 创建用户和角色 admin_role = Role("Admin", ["create_user", "delete_user"]) user_role = Role("User", ["view_user"]) admin_user = User("admin", admin_role) user_user = User("user", user_role) # 检查用户的权限 print(admin_user.has_permission("create_user")) # True print(user_user.has_permission("delete_user")) # False
Conclusion
Function security and permission management are Critical security practices to help protect your applications from attacks. By following these best practices, you can improve application security and build a strong security posture.
The above is the detailed content of Best practices for function security and permission management. For more information, please follow other related articles on the PHP Chinese website!
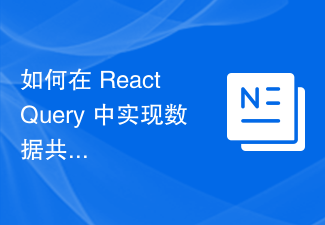
如何在ReactQuery中实现数据共享和权限管理?技术的进步使得前端开发中的数据管理变得更加复杂。传统的方式中,我们可能使用Redux或者Mobx等状态管理工具来处理数据的共享和权限管理。然而,在ReactQuery的出现之后,我们可以通过它来更加方便地处理这些问题。在本文中,我们将介绍如何在ReactQuery中实现数据共享和权
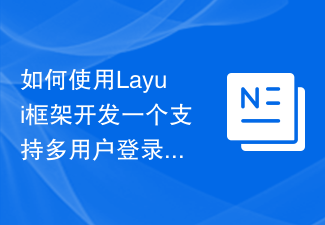
如何使用Layui框架开发一个支持多用户登录的权限管理系统引言:在现代的互联网时代,越来越多的应用程序需要支持多用户登录,以实现个性化的功能和权限管理。为了保护系统的安全性和数据的私密性,开发者需要使用一定的手段来实现多用户登录和权限管理的功能。本文将介绍如何使用Layui框架来开发一个支持多用户登录的权限管理系统,并给出具体的代码示例。准备工作在开始开发之
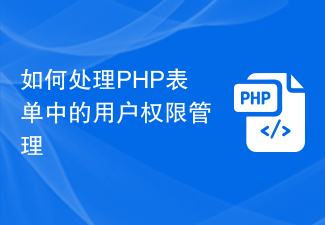
如何处理PHP表单中的用户权限管理随着Web应用程序的不断发展,用户权限管理是一个重要的功能之一。用户权限管理可以控制用户在应用程序中的操作权限,保证数据的安全性和合法性。在PHP表单中,用户权限管理可以通过一些简单的代码来实现。本文将介绍如何处理PHP表单中的用户权限管理,并给出相应的代码示例。一、用户角色的定义和管理首先,对用户角色进行定义和管理是用户权
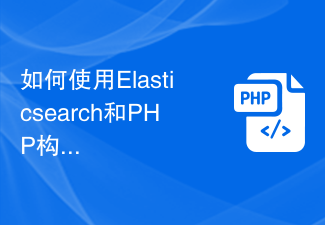
如何使用Elasticsearch和PHP构建用户登录和权限管理系统引言:在当前的互联网时代,用户登录和权限管理是每个网站或应用程序必备的功能之一。Elasticsearch是一个强大而灵活的全文搜索引擎,而PHP是一种广泛使用的服务器端脚本语言。本文将介绍如何结合Elasticsearch和PHP来构建一个简单的用户登录和权限管理系统
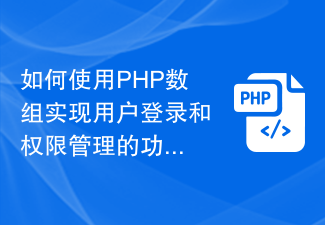
如何使用PHP数组实现用户登录和权限管理的功能在开发网站时,用户登录和权限管理是非常重要的功能之一。通过用户登录,我们可以验证用户身份并保护网站的安全性。而权限管理则能够控制用户在网站中的操作权限,确保用户只能访问他们被授权的功能。在本文中,我们将介绍如何使用PHP数组来实现用户登录和权限管理的功能。我们将使用一个简单的示例来演示这个过程。首先,我们需要创建
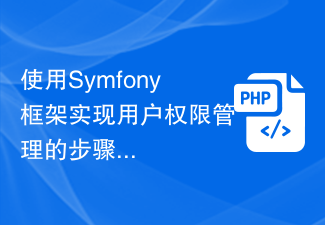
使用Symfony框架实现用户权限管理的步骤Symfony框架是一个功能强大的PHP开发框架,使用它可以快速开发出高质量的Web应用程序。在开发Web应用程序时,用户权限管理是一个不可忽视的重要部分。本文将介绍使用Symfony框架实现用户权限管理的步骤,并附带代码示例。第一步:安装Symfony框架首先,我们需要在本地环境中安装Symfony框架。可以通过
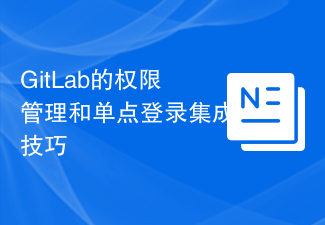
GitLab的权限管理和单点登录集成技巧,需要具体代码示例概述:在GitLab中,权限管理和单点登录(SSO)是非常重要的功能。权限管理可以控制用户对代码仓库、项目和其他资源的访问权限,而单点登录集成可以提供更方便的用户认证和授权方式。本文将介绍如何在GitLab中进行权限管理和单点登录集成。一、权限管理项目访问权限控制在GitLab中,项目可以被设置为私有
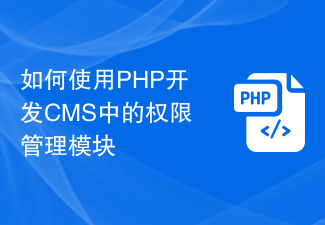
随着互联网的普及和发展,更多的网站和应用程序需要实现权限管理模块,以保护自身的安全和保密性。而作为一种流行的后端编程语言,PHP也广泛应用于各种CMS(ContentManagementSystem)中,如WordPress、Joomla、Drupal等。因此,在本文中我们将探讨如何使用PHP开发CMS中的权限管理模块。一、CMS中的权限管理模块在CMS


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
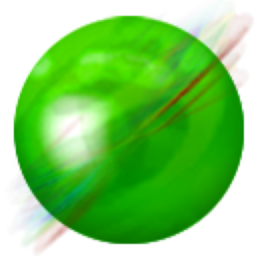
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
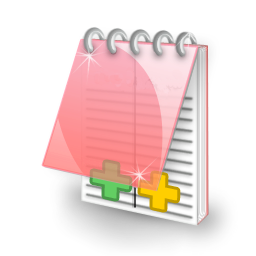
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
