The order of best practices: 1. Prioritize passing by value; 2. Pass mutable objects by reference; 3. Pass large objects through pointers; 4. Avoid passing basic types through pointers; 5. Clearly state the transfer method.
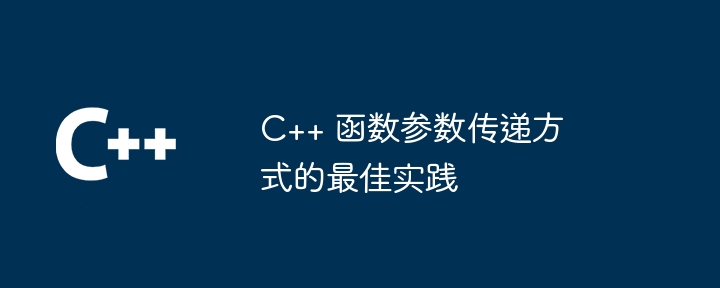
C Best practices for passing function parameters
Introduction
C A variety of function parameter passing methods are provided, each method has its own advantages and disadvantages. In order to improve code efficiency, readability, and maintainability, it is important to understand and use these delivery methods correctly.
Transmission method
-
Pass-by-value: Copy the parameter value provided when the function is called to In a function, any modifications within the function will not affect the original value.
-
Pass-by-reference: Pass the reference of the parameter provided when the function is called into the function, so any modification to the parameter inside the function will be reflected in the original value middle.
-
Pass-by-pointer: Pass the pointer of the parameter provided when the function is called into the function, so any modifications to the object pointed to by the pointer inside the function will be reflected in the original object.
Best Practice
-
Pass by value first: This is the safest and most efficient way, because It does not cause unexpected side effects.
-
Pass mutable objects by reference: When an object needs to be modified in a function, it should be passed by reference.
-
Passing large objects by pointer: When objects are large (more than a few kilobytes), they can be passed by pointer to avoid copy overhead.
-
Avoid passing basic types through pointers: For basic types (such as int, float), they should be passed by value because pointer overhead is relatively high.
-
Explicitly specify the transfer method: Use modifiers such as const, & and * in the function declaration to explicitly specify the transfer method.
Practical Case
Consider the following function, which calculates the sum of two integers:
int sum(int a, int b) {
return a + b;
}
If we call this function passing by value :
int main() {
int x = 5;
int y = 10;
int result = sum(x, y);
}
The result is 15, and x and y remain unchanged.
If we call this function by reference:
int sum(int &a, int &b) {
a += 5;
b -= 2;
return a + b;
}
int main() {
int x = 5;
int y = 10;
int result = sum(x, y);
}
The result is 18, and x and y become 10 and 8 respectively.
The above is the detailed content of Best practices for passing function parameters in C++. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn