A function pointer is a pointer to a function, used to implement callbacks, anonymous functions and generic programming. Syntax: returnType (*functionName)(parameterList). To use: Declare a pointer to the desired function type and use the function pointer like any other pointer. Practical case: Implement a sorting function and pass a comparison function to customize the comparison logic.
Guidelines for the use of C function pointers
Introduction
The function pointer is a A special type of pointer that points to a function. In C, function pointers are mainly used to implement callback functions, anonymous functions and generic programming.
Syntax
The syntax of a function pointer is as follows:
returnType (*functionName)(parameterList);
Among them:
-
returnType
Is the return type of the function pointed to by the pointer. -
functionName
is the name of the function pointer. -
parameterList
is the parameter list of the function pointed to by the pointer.
Usage
To use a function pointer, you need to first declare a function pointer pointing to the desired function type. For example:
int add(int a, int b) { return a + b; } int (*sumFunction)(int, int); // 声明一个指向 add 函数的函数指针
Then, function pointers can be used like other pointers. For example:
// 将 sumFunction 指向 add 函数 sumFunction = &add; // 调用 sumFunction int result = sumFunction(10, 20); // result 将等于 30
Practical case
Scenario: Implement a sorting function that allows passing a comparison function to customize the comparison logic
#include <algorithm> #include <vector> // 比较函数 bool compare(int a, int b) { return a > b; } // 排序函数,接受一个比较函数 void sortDescending(std::vector<int>& numbers, bool (*compareFunction)(int, int)) { std::sort(numbers.begin(), numbers.end(), compareFunction); } int main() { std::vector<int> numbers = {1, 5, 3, 2, 4}; // 使用比较函数排序数组 sortDescending(numbers, compare); // 输出排序后的数组 for (int number : numbers) { std::cout << number << " "; } return 0; }
In this practical case, the compare
function pointer is passed to the sortDescending
function. sortDescending
Function sorts vectors using comparison functions. Therefore, the output will be the vector elements sorted in descending order: 5 4 3 2 1
.
The above is the detailed content of How to use function pointers in C++?. For more information, please follow other related articles on the PHP Chinese website!
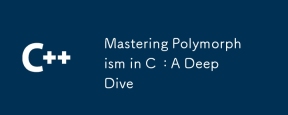
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
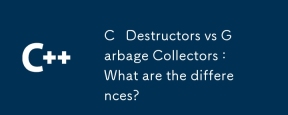
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
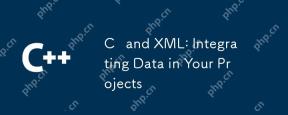
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
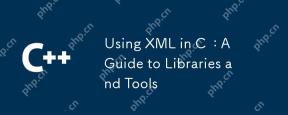
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
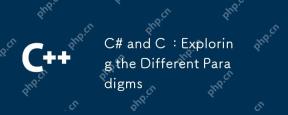
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
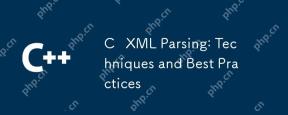
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
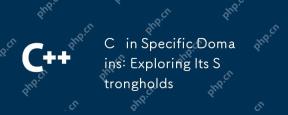
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
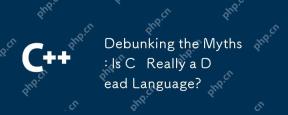
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
