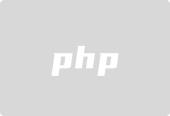
Best strategies for function maintainability, extensibility, and testability
Write maintainability, extensibility, and testability Functions are critical to ensuring high quality and long-term scalability of your code. The following strategies will guide you in creating more robust functions:
Modularity and Reusability
- Divide functions into small, specific modules that facilitate Understand and maintain.
- Improve reusability by extracting common functionality into reusable functions.
Clear documentation
- Use comments and docstrings to clearly describe a function’s purpose, parameters, and return values.
- Define input and output specifications to ensure correct use.
Single Principle
- Each function performs only one task, which simplifies debugging and testing.
- Avoid using complex or nested logic in functions.
Input Validation and Error Handling
- Validate function input to ensure validity and completeness.
- Use exceptions or error codes to handle errors gracefully, making it easier for callers to diagnose problems.
Unit Testing
- Write unit tests to cover different branches and scenarios of the function.
- Use the assertion library to verify expected results.
Scalability
- Use parameters to pass configurable options, such as log level or number of threads.
- Design functions through interfaces or abstract classes to promote extensibility, allowing components to be easily added or replaced.
Maintainability
- Keep your code style consistent and follow best practices.
- Use a version control system to track code changes.
- Write automated tests to quickly detect regressions.
Practical case: Customer verification function
This is an example of a function that verifies customer email and password:
def validate_customer(email: str, password: str) -> bool:
"""验证客户凭据。
Args:
email (str): 客户电子邮件
password (str): 客户密码
Returns:
bool: 客户凭据是否有效
"""
if not email or not password:
raise ValueError("Email or password cannot be empty")
db_user = get_user_from_database(email)
if not db_user:
return False
return db_user.check_password(password)
Advantages:
- The modularity is clear and easy to understand.
- The documentation describes the purpose and parameters of the function in detail.
- Single Principle only verifies customer credentials.
- Validate input to ensure the input is valid.
- Exceptions are used for error handling.
- Written unit tests to verify the correctness of the function.
The above is the detailed content of Best strategies for function maintainability, scalability, and testability. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn