What is the life cycle of Java objects?
Java object life cycle includes: object creation, initialization, reachability, invalidation, and recycling. Objects are created through the new keyword, initialization is performed in the constructor, reachability refers to access through reference variables, invalidation refers to no longer reachable, and recycling refers to the garbage collector releasing the memory of the invalid object.
The life cycle of Java objects
Java objects go through the following stages in their life cycles:
1. Create a
- object first created through the
new
keyword. - When the
new
keyword is executed, the JVM will allocate memory and call the object's constructor.
2. Initialization
- When the constructor is executed, the object will be initialized.
- This is the stage of setting the initial value of the object.
3. Reachability
- When an object is created, it is reachable.
- Reachability means that the object can be accessed through a reference variable.
4. Invalidation
- When an object is no longer reachable, it is considered invalid.
- Invalid objects are managed by the garbage collector (GC).
5. Recycling
- The garbage collector will reclaim the memory of expired objects.
- This helps prevent memory leaks and frees resources for use by new objects.
Practical case:
public class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } // ... } public class Main { public static void main(String[] args) { // 创建一个 Person 对象 Person person = new Person("John", 30); // 对象可达并且可以访问 System.out.println(person.name); // 打印 "John" // 将 person 引用赋值给 null,对象失活 person = null; // 垃圾回收器将回收 person 对象使用的内存 } }
In this case, when the person
reference is assigned the value null
, the Person
object will become invalid. The object's memory will then be reclaimed by the garbage collector.
The above is the detailed content of What is the life cycle of Java objects?. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
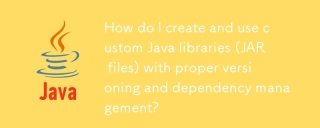
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
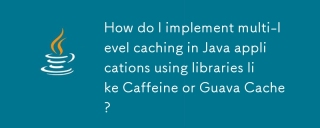
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
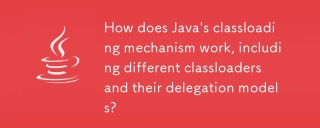
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
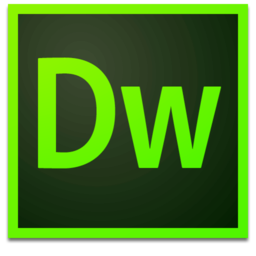
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.