


Limitations of Go functions include: 1) The inability to pass functions as parameters, limiting the use of callbacks and closures; 2) The lack of tail recursion optimization, which affects the performance of recursive functions; 3) The use of pointer receivers may lead to data races ;4) The use of closures is limited, which may lead to memory leaks and concurrency issues. By understanding these limitations and designing your functions appropriately, you can minimize the impact on performance.
Limitations of Go functions and their impact on performance
The Go language is known for its powerful concurrency. Functional design aims to provide efficient and scalable code. However, Go functions also have some limitations compared to other languages, which may affect their performance.
Cannot pass functions as parameters
Functions in Go cannot be passed to other functions as parameters. This makes features such as callbacks and closures more difficult to implement, requiring the use of channels or goroutines to emulate these features. This limitation reduces code readability and increases complexity.
Practical Case
Suppose we want to create a function that processes a list of files and performs a specific operation on each file. In other languages, we can pass operation functions as parameters as follows:
def process_files(files: list, operation: function) -> None: for file in files: operation(file)
In Go, we have to use goroutines to simulate similar behavior:
func processFiles(files []string, operation func(string)) { for _, file := range files { go operation(file) } }
With passing functions as parameters In comparison, this approach introduces additional complexity and needs to deal with goroutine synchronization.
Missing tail recursion optimization
Go does not have tail recursion optimization, which may affect the performance of some recursive functions. If a function calls itself as the last call, tail recursion optimization can improve efficiency by converting the recursive call into a loop. In Go, the lack of this optimization increases the stack usage of functions.
Practical Case
When calculating Fibonacci numbers, tail recursion optimization can significantly improve efficiency. In Go, we need to use a loop to simulate tail recursion, as shown below:
func fib(n int) int { a, b := 0, 1 for i := 0; i < n; i++ { a, b = b, a + b } return a }
This loop version is less efficient compared to the tail recursive version, especially when dealing with large values of n.
Pointer Receivers
Methods in Go use pointer receivers, which means they can modify the receiver's value. While this can be useful in some situations, it can also result in code that is difficult to understand and debug. Especially in concurrent environments, pointer receivers can cause data races.
Restricted use of closures
Closures are restricted in Go because they must survive based on the lifetime of the variable last referenced by the closure. This can cause memory leaks and limit the use of closures in concurrent environments.
By understanding these limitations and designing functions carefully, Go programmers can mitigate their impact on performance. In some cases, it may be necessary to weigh the trade-offs of these limitations and use alternatives such as goroutines or callbacks.
The above is the detailed content of What are the disadvantages of Golang functions relative to functions in other languages?. For more information, please follow other related articles on the PHP Chinese website!
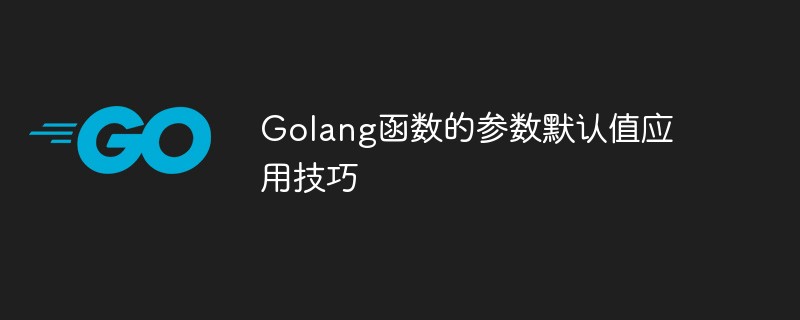
Golang是一门现代化的编程语言,拥有很多独特且强大的功能。其中之一就是函数参数的默认值应用技巧。本文将深入探讨如何使用这一技巧,以及如何优化代码。一、什么是函数参数默认值?函数参数默认值是指定义函数时为其参数设置默认值,这样在函数调用时,如果没有给参数传递值,则会使用默认值作为参数值。下面是一个简单的例子:funcmyFunction(namestr
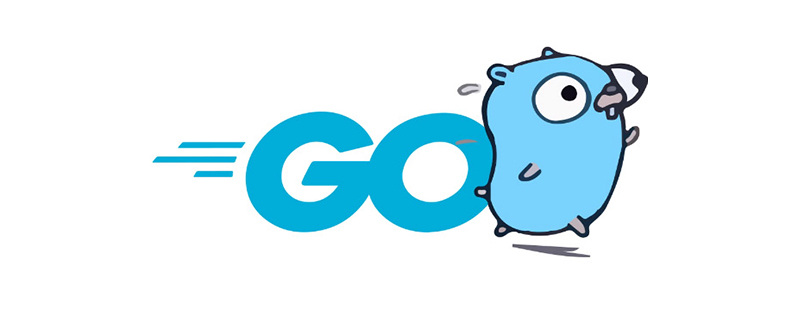
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
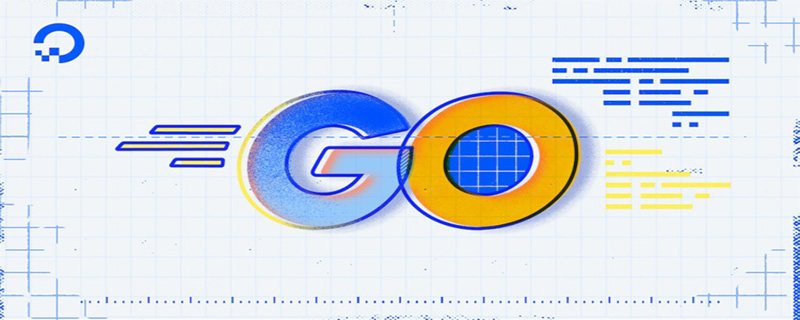
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
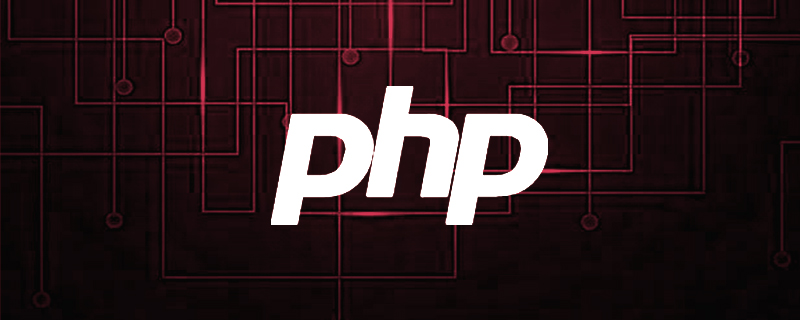
发现 Go 不仅允许我们创建更大的应用程序,并且能够将性能提高多达 40 倍。 有了它,我们能够扩展使用 PHP 编写的现有产品,并通过结合两种语言的优势来改进它们。
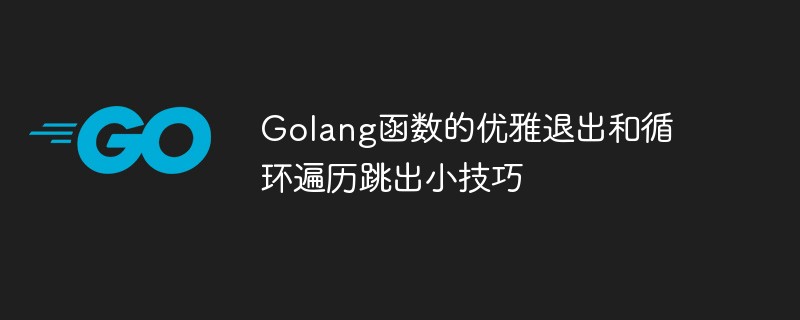
Golang作为一门开发效率高、性能优异的编程语言,其强大的函数功能是其关键特性之一。在开发过程中,经常会遇到需要退出函数或循环遍历的情况。本文将介绍Golang函数的优雅退出和循环遍历跳出小技巧。一、函数的优雅退出在Golang编程中,有时候我们需要在函数中优雅地退出。这种情况通常是因为我们在函数中遇到了一些错误或者函数的执行结果与预期不符的情况。有以下两
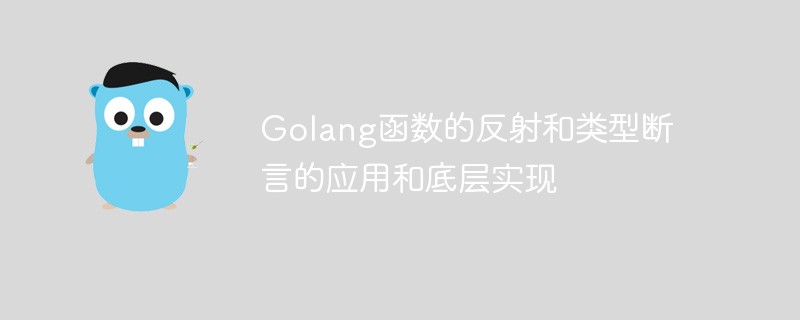
Golang函数的反射和类型断言的应用和底层实现在Golang编程中,函数的反射和类型断言是两个非常重要的概念。函数的反射可以让我们在运行时动态的调用函数,而类型断言则可以帮助我们在处理接口类型时进行类型转换操作。本文将深入讨论这两个概念的应用以及他们的底层实现原理。一、函数的反射函数的反射是指在程序运行时获取函数的具体信息,比如函数名、参数个数、参数类型等
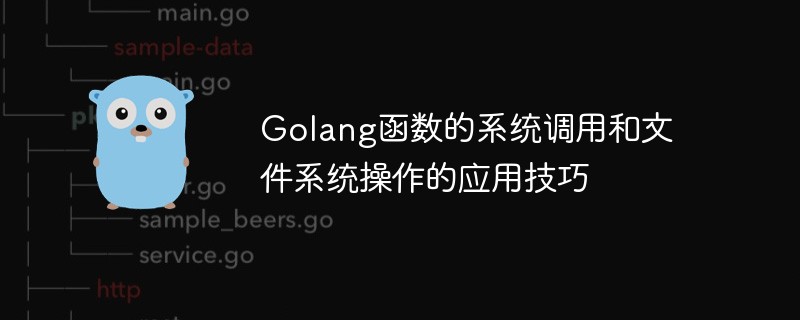
随着计算机技术的不断发展,各种语言也应运而生。其中,Golang(又称GO语言)因为其高效、简单、易于学习的特点,在近年来越来越受到开发者们的青睐。在Golang中,函数的系统调用和文件系统操作是常见的应用技巧。本文将详细介绍这些技巧的应用方法,以帮助大家更好地掌握Golang的开发技能。一、函数的系统调用1.系统调用是什么?系统调用是操作系统内核提供的服务


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
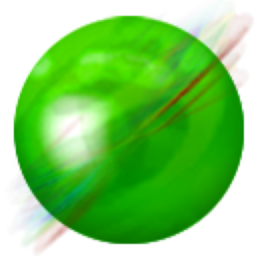
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
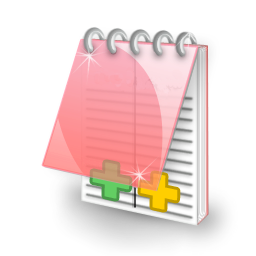
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
