What are the usage scenarios and advantages of C++ function pointers?
Function pointers allow storing references to functions, providing additional flexibility. Usage scenarios include event processing, algorithm sorting, data transformation and dynamic polymorphism. Benefits include flexibility, decoupling, code reuse, and performance optimization. Practical applications include event processing, algorithmic sorting, and data transformation. Function pointers allow C programmers to create flexible and dynamic code.
Usage scenarios and advantages of C function pointers
Function pointers allow you to store references to functions in the form of variables. This provides additional flexibility to the code, allowing it to dynamically call functions at runtime.
Usage scenarios:
- Event handling: Register event handlers with callback-based systems.
- Algorithmic sorting: Use function pointers as comparison functions to specify custom sorting rules.
- Data conversion: Convert data from one format to another through function pointers.
- Dynamic polymorphism: Determine the function to be called at runtime without using virtual functions.
Advantages:
- Flexibility: Allows function calls to be modified dynamically while the code is executing.
- Decoupling: Decouple function implementation from call points to improve modularity and code maintainability.
- Code reuse: Function pointers can be passed to other functions or stored in data structures to facilitate code reuse.
- Performance Optimization: In some cases, function pointers can improve performance because they eliminate the need for virtual function table lookups.
Practical case:
Event processing:
class Button { public: void setOnClick(void(*handler)()) { onClickHandler = handler; } void click() { if (onClickHandler) { onClickHandler(); } } private: void(*onClickHandler)() = nullptr; };
Algorithmic sorting:
struct Person { string name; int age; }; int compareByName(const Person& a, const Person& b) { return a.name.compare(b.name); } void sortPeopleByName(vector<Person>& people) { sort(people.begin(), people.end(), compareByName); }
Data conversion:
double toCelsius(double fahrenheit) { return (fahrenheit - 32) * 5 / 9; } double convertTemperature(double value, double(*conversionFunction)(double)) { return conversionFunction(value); }
Using the flexibility of function pointers, C programmers can create highly flexible and dynamic code to meet a variety of different programming needs.
The above is the detailed content of What are the usage scenarios and advantages of C++ function pointers?. For more information, please follow other related articles on the PHP Chinese website!
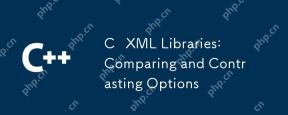
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
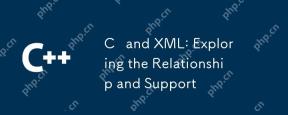
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
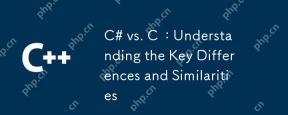
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
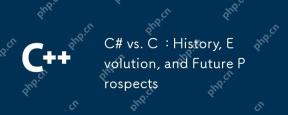
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
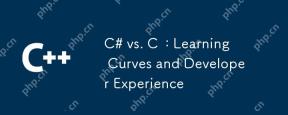
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
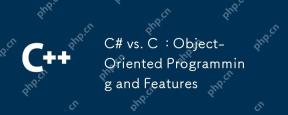
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
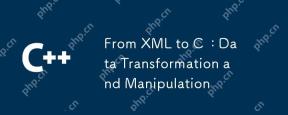
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
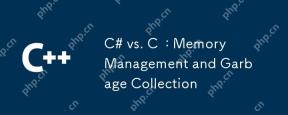
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
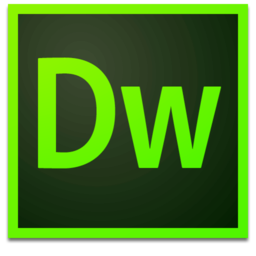
Dreamweaver Mac version
Visual web development tools
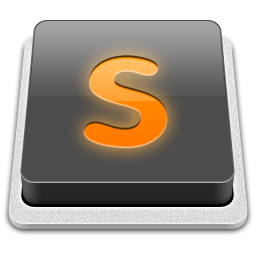
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
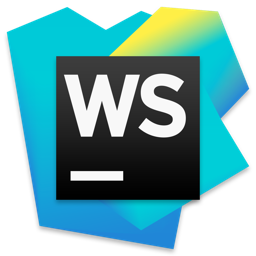
WebStorm Mac version
Useful JavaScript development tools