Methods to implement functional programming in Go include using anonymous functions, closures, and higher-order functions. These functions allow defining unbound functions, accessing variables in the outer scope, and accepting or returning other functions. With functional programming, Go code can become more concise, readable, and reusable.
Introduction to functional programming in Go
Functional programming is a software development paradigm that emphasizes the use of mathematical functions and inexhaustible Change data. In Go, functional programming can be achieved through the use of anonymous functions, closures, and higher-order functions.
Anonymous functions
Anonymous functions are unbound functions that can be defined and used when needed. For example:
func main() { func() { fmt.Println("Hello world!") }() }
Closure
A closure is a function that can access variables outside the scope of its definition. For example:
func main() { x := 10 f := func() int { return x + 1 } fmt.Println(f()) // 输出:11 }
Higher-order functions
Higher-order functions are functions that accept functions as parameters or return functions. For example:
Receive function as parameter
func mapFunc(f func(int) int, nums []int) []int { res := make([]int, len(nums)) for i, num := range nums { res[i] = f(num) } return res }
Return function
func makeIncrementer(x int) func() int { return func() int { x++ return x } }
Practical example
The following is an example of using functional programming in Go to write a simple password hash function:
package main import ( "crypto/sha256" "fmt" "log" ) func main() { // 定义密码哈希函数 hashFunc := func(password string) string { return fmt.Sprintf("%x", sha256.Sum256([]byte(password))) } // 使用高阶函数 mapFunc 将哈希函数应用到密码列表上 passwords := []string{"password1", "password2", "password3"} hashedPasswords := mapFunc(hashFunc, passwords) // 输出哈希后的密码 for _, hashedPassword := range hashedPasswords { fmt.Println(hashedPassword) } }
By using functional programming techniques, we can write a simpler, more readable and More reusable Go code.
The above is the detailed content of How to use Golang functional programming?. For more information, please follow other related articles on the PHP Chinese website!
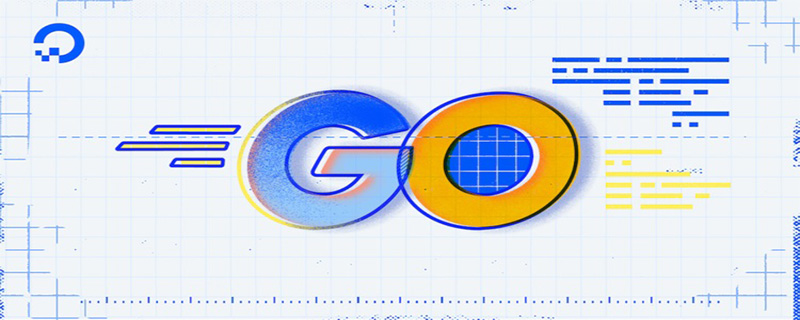
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
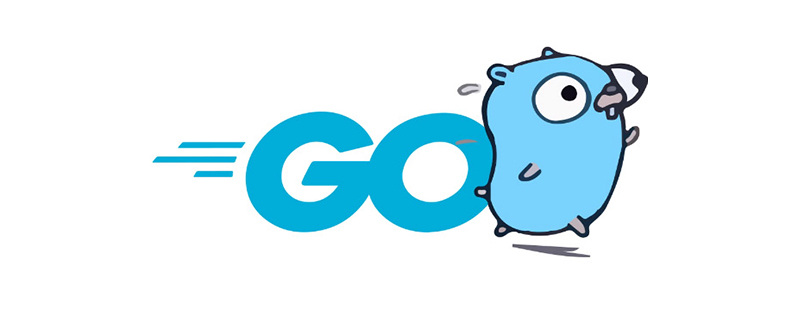
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
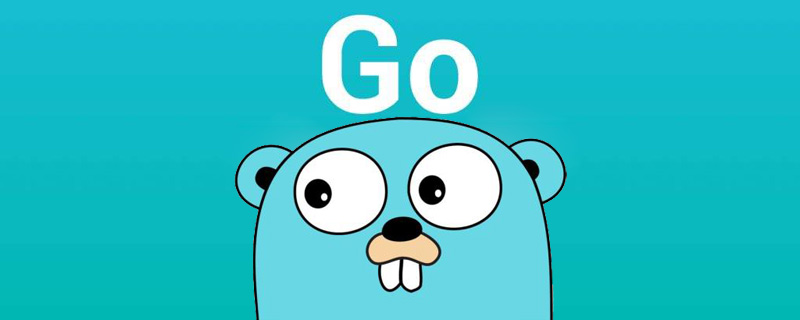
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
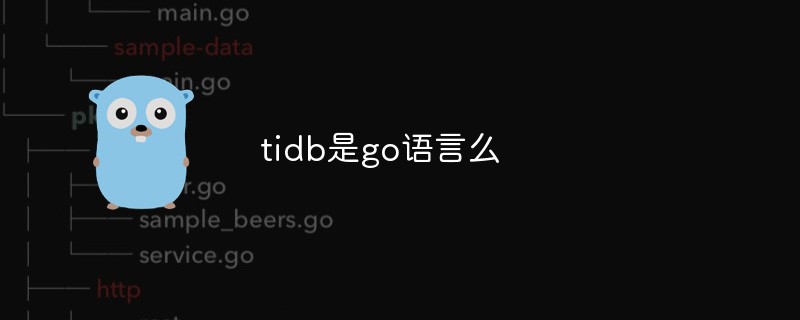
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
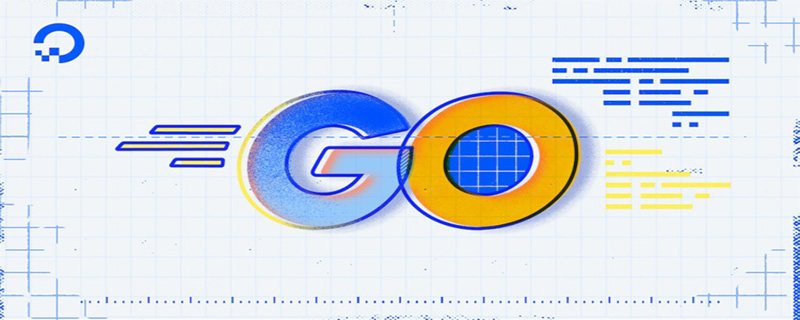
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
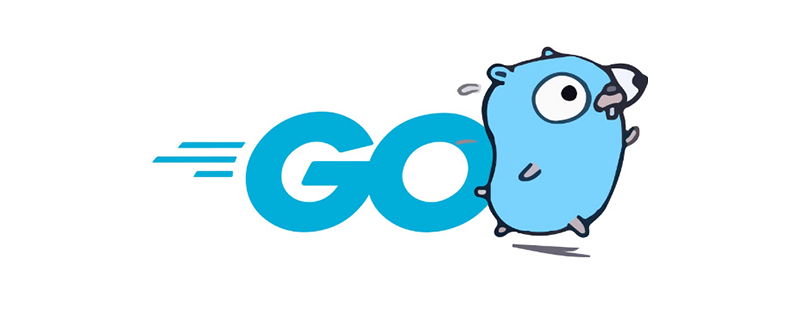
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
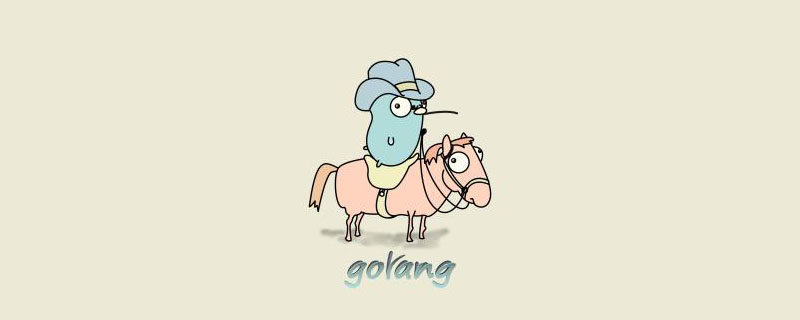
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
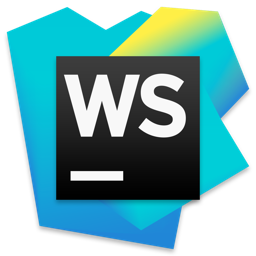
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
