Methods to identify performance bottlenecks in PHP functions include using performance analysis tools and viewing application logs. Once you analyze a performance bottleneck, you can determine the root cause by examining the function call stack, using performance analysis tools, and manually analyzing the code. Optimization suggestions include avoiding unnecessary function calls, caching results, optimizing database queries and using parallel processing. In practical cases, using multi-threading to process block arrays significantly improves performance.
Analysis of performance bottlenecks of PHP functions
Performance bottlenecks of PHP functions often affect the performance of applications. This article will discuss how to identify and analyze performance bottlenecks of PHP functions, and provide optimization suggestions and practical cases.
Identify performance bottlenecks
Here are some ways to identify performance bottlenecks:
- Use a performance analysis tool such as XHProf or Blackfire.io.
- Enable PHP's built-in debugging tools, such as
xdebug
ortideways
. - View application logs for exceptions or errors.
Analyze performance bottlenecks
Once a performance bottleneck is identified, its root cause needs to be analyzed. You can use the following tips:
- Examine the stack trace of the function call to determine which function is causing the bottleneck.
- Use performance analysis tools to obtain information about function execution times and memory allocations.
- Manually analyze function code to find potential bottlenecks, such as nested loops or unnecessary I/O operations.
Optimization suggestions
- Avoid unnecessary function calls: only call functions when needed.
- Caching results: If the output of a function does not change frequently, consider caching its results to avoid double calculations.
- Optimize database queries: use indexes, limit result set size, and use precompiled queries when possible.
- Use parallel processing: Break up tasks and use multiple threads or processes to process them simultaneously.
Practical case
Problem: A loop traverses a large array and calculates each element.
Bottleneck: Array traversal is a performance bottleneck.
Optimization: Performance can be significantly improved by using the array_chunk()
function to split the array into smaller chunks and using multiple threads to process these chunks simultaneously.
// 原始代码 $array = range(1, 10000); foreach ($array as $item) { // 执行计算 } // 优化代码 $chunks = array_chunk($array, 100); $threads = []; foreach ($chunks as $chunk) { $threads[] = new Thread(function() use ($chunk) { // 执行计算 }); } foreach ($threads as $thread) { $thread->start(); } foreach ($threads as $thread) { $thread->join(); }
Conclusion
By following these steps, you can identify and analyze performance bottlenecks in your PHP functions. By implementing optimization recommendations and working on real-life examples, application performance can be significantly improved.
The above is the detailed content of Analyzing performance bottlenecks of PHP functions. For more information, please follow other related articles on the PHP Chinese website!
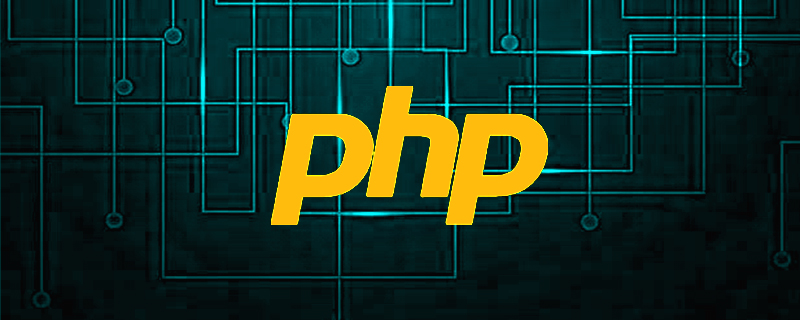
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
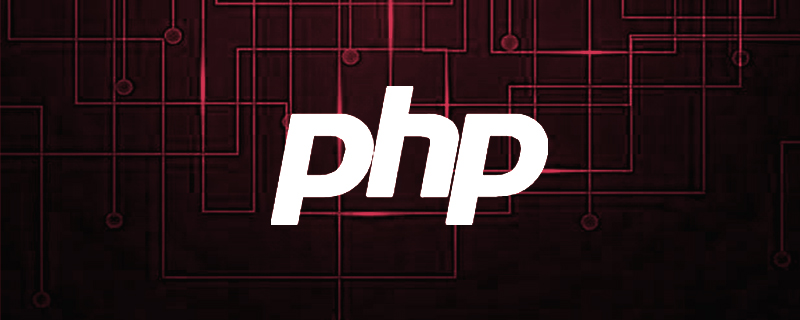
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
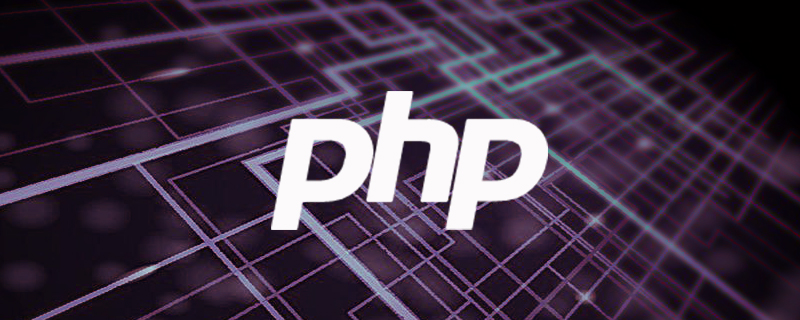
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
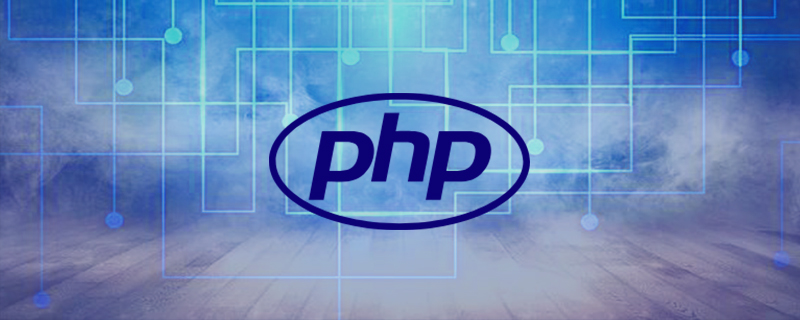
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
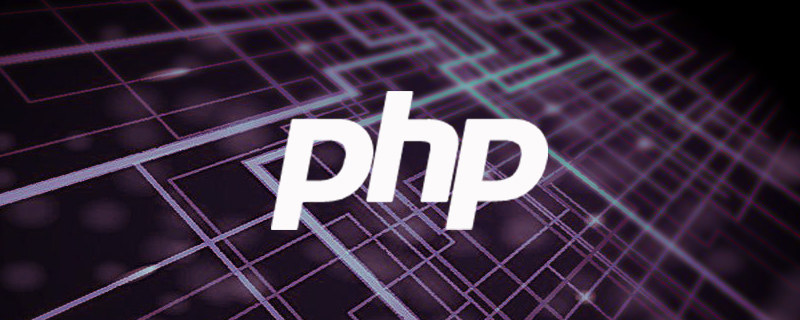
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
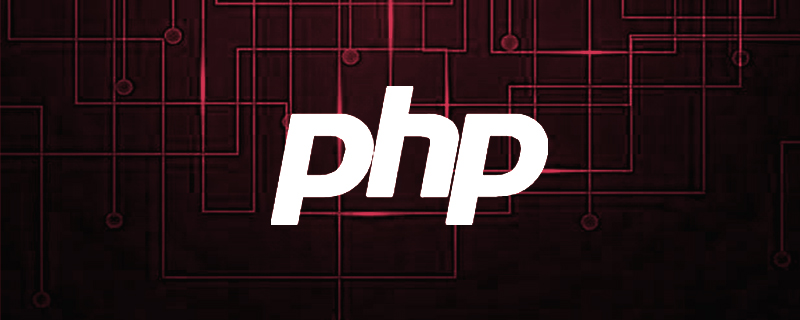
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
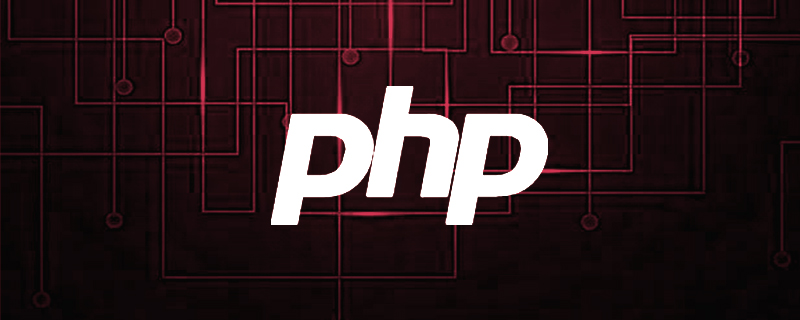
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
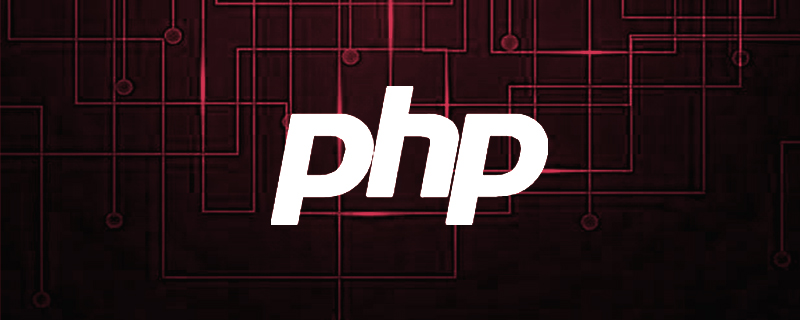
查找方法:1、用strpos(),语法“strpos("字符串值","查找子串")+1”;2、用stripos(),语法“strpos("字符串值","查找子串")+1”。因为字符串是从0开始计数的,因此两个函数获取的位置需要进行加1处理。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
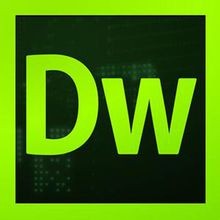
Dreamweaver CS6
Visual web development tools
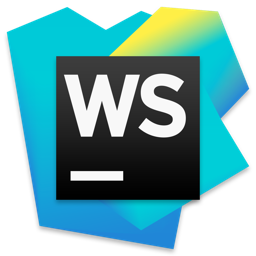
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.