To use HTML to read JSON files, you need to: create JavaScript objects to parse JSON strings; use JavaScript to access JSON data, including properties, array elements, and nested objects.
How to read a JSON file using HTML
To read a JSON file using HTML, you can use the following steps:
1. Create a JavaScript object to parse JSON
const obj = JSON.parse(jsonString);
where jsonString
is a string containing JSON data.
2. Use JavaScript to access JSON data
After parsing the JSON string, you can use JavaScript to access the data in it. Here are some examples:
// 访问 JSON 对象的属性 console.log(obj.name); // 访问 JSON 数组的元素 console.log(obj[0]); // 访问 JSON 嵌套对象 console.log(obj.nested.value);
Sample code:
<script> // 假设 JSON 数据已存储在 JSONString 变量中 // 解析 JSON 字符串 const obj = JSON.parse(jsonString); // 访问 JSON 数据 console.log(obj.name); // 输出:John console.log(obj[0]); // 输出:{name: "Alice", age: 25} console.log(obj.nested.value); // 输出:100 </script>
Other notes:
- JSON data is required is a valid JSON format.
- If the JSON data contains special characters, the string may need to be escaped.
- You can use the
XMLHttpRequest
orfetch
API to get JSON data from the server side.
The above is the detailed content of How to read json file in html. For more information, please follow other related articles on the PHP Chinese website!

HTML is not only the skeleton of web pages, but is more widely used in many fields: 1. In web page development, HTML defines the page structure and combines CSS and JavaScript to achieve rich interfaces. 2. In mobile application development, HTML5 supports offline storage and geolocation functions. 3. In emails and newsletters, HTML improves the format and multimedia effects of emails. 4. In game development, HTML5's Canvas API is used to create 2D and 3D games.

TheroottaginanHTMLdocumentis.Itservesasthetop-levelelementthatencapsulatesallothercontent,ensuringproperdocumentstructureandbrowserparsing.
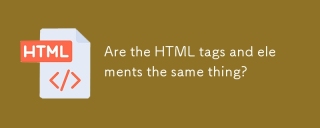
The article explains that HTML tags are syntax markers used to define elements, while elements are complete units including tags and content. They work together to structure webpages.Character count: 159
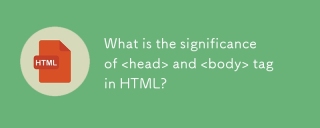
The article discusses the roles of <head> and <body> tags in HTML, their impact on user experience, and SEO implications. Proper structuring enhances website functionality and search engine optimization.
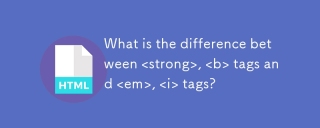
The article discusses the differences between HTML tags , , , and , focusing on their semantic vs. presentational uses and their impact on SEO and accessibility.
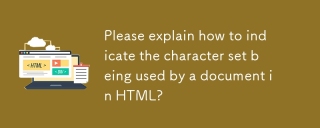
Article discusses specifying character encoding in HTML, focusing on UTF-8. Main issue: ensuring correct display of text, preventing garbled characters, and enhancing SEO and accessibility.
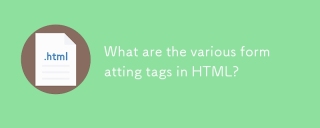
The article discusses various HTML formatting tags used for structuring and styling web content, emphasizing their effects on text appearance and the importance of semantic tags for accessibility and SEO.
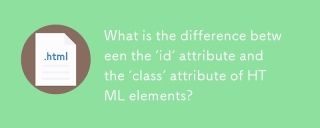
The article discusses the differences between HTML's 'id' and 'class' attributes, focusing on their uniqueness, purpose, CSS syntax, and specificity. It explains how their use impacts webpage styling and functionality, and provides best practices for


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment
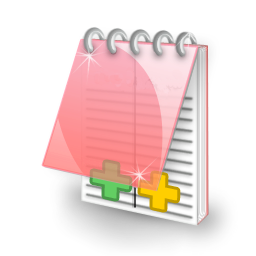
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
