Go 中的正则表达式提供了一种强大的字符串处理工具:使用 regexp 包进行正则表达式操作。利用正则表达式语法来匹配和操作字符串。可匹配字符类、重复字符、分组、锚点和边界符。通过 MatchString 匹配字符串、FindStringSubmatch 提取匹配和 ReplaceAllString 替换字符串。应用场景包括验证电子邮件地址、提取 HTML 链接等。
Golang 正则表达式的使用指南
简介
正则表达式(regex)是一种强大的工具,用于匹配和操作字符串。Go 提供了内置的 regexp
包,可以帮助你轻松使用正则表达式。
正则表达式语法
正则表达式通常使用以下语法:
-
字符类: 用于匹配单个字符,例如
[abc]
匹配 'a'、'b' 或 'c'。 -
重复字符: 限定符用于指定字符重复的次数,例如
+
表示一次或多次。 -
分组: 圆括号用于分组字符,例如
(abc)
匹配字符串 "abc"。 -
锚点: 用于匹配字符串的开头或结尾,例如
^
和$
。 -
边界符: 用于匹配字符串的单词或行边界,例如
\b
。
Go 中的正则表达式
要使用 regexp
包,请导入它:
import "regexp"
以下是一些在 Go 中使用正则表达式的示例代码:
匹配字符串
re := regexp.MustCompile("abc") fmt.Println(re.MatchString("abcdef")) // 输出:true
提取匹配
re := regexp.MustCompile("(\\d+)-([a-z]+)") fmt.Println(re.FindStringSubmatch("123-abc")) // 输出:["123-abc", "123", "abc"]
替换字符串
re := regexp.MustCompile("abc") fmt.Println(re.ReplaceAllString("abcdef", "xyz")) // 输出:"xyzdef"
实战案例
验证电子邮件地址
re := regexp.MustCompile("^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$") fmt.Println(re.MatchString("username@example.com")) // 输出:true
从 HTML 中提取链接
re := regexp.MustCompile(`<a href="([^"]+)">`) for _, link := range re.FindAllStringSubmatch(`<a href="/page1">Page 1</a> <a href="/page2">Page 2</a>`, -1) { fmt.Println(link[1]) // 输出:"page1" 和 "page2" }
The above is the detailed content of Golang regular expression usage guide. For more information, please follow other related articles on the PHP Chinese website!
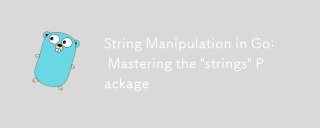
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
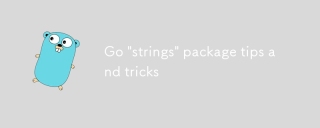
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
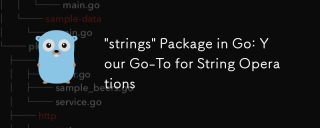
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
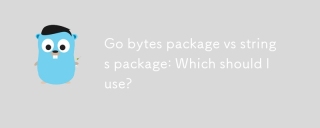
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
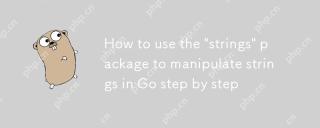
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
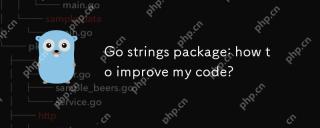
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
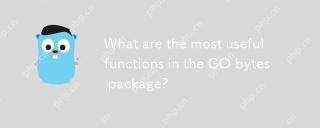
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
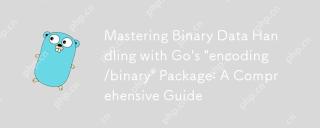
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
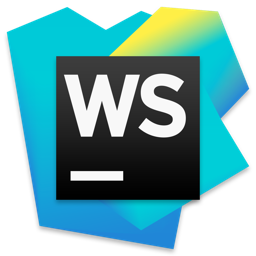
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
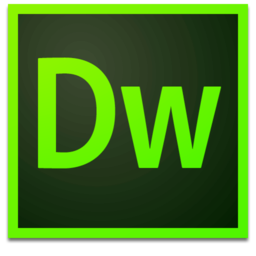
Dreamweaver Mac version
Visual web development tools
