Implement data serialization/deserialization in Golang using Gob
Using the gob package to serialize data in Golang requires the following steps: implement the GobEncode and GobDecode interfaces for the type to be serialized. Use gob.NewEncoder and gob.NewDecoder to create encoders and decoders. Use an encoder to serialize data and a decoder to deserialize it. Serialized data can be written to a file or transferred over the network.
Use Gob to implement data serialization in Golang
In Golang, encoding/gob
package Provides a convenient and efficient way to serialize and deserialize data. This article will introduce the steps to implement data serialization using the gob
package, and provide some practical cases.
Installation gob
Package
gob
The package is part of the Go standard library, so there is no need to install it manually.
Type preparation
In order to use gob to serialize data, your type must implement the GobEncode
and GobDecode
interfaces. These interfaces define how types are serialized and deserialized into sequences of bytes.
Type implementation
The following is an example implementation of the Person type:
package main import ( "encoding/gob" "log" ) type Person struct { Name string Age int } func (p Person) GobEncode(w gob.Encoder) error { if err := w.Encode(p.Name); err != nil { return err } return w.Encode(p.Age) } func (p *Person) GobDecode(r gob.Decoder) error { if err := r.Decode(&p.Name); err != nil { return err } return r.Decode(&p.Age) } func main() { // 序列化 Person var buff bytes.Buffer enc := gob.NewEncoder(&buff) if err := enc.Encode(Person{"Alice", 25}); err != nil { log.Fatal(err) } // 反序列化 Person dec := gob.NewDecoder(&buff) var p Person if err := dec.Decode(&p); err != nil { log.Fatal(err) } fmt.Println(p) // {Alice 25} }
Practical case
Case 1: Serializing data to a file
Data can be serialized to a file for later use.
// 将 Person 序列化到文件中 func WriteToFile(p Person, filename string) error { f, err := os.Create(filename) if err != nil { return err } defer f.Close() enc := gob.NewEncoder(f) return enc.Encode(p) } // 从文件中反序列化 Person func ReadFromFile(filename string) (*Person, error) { f, err := os.Open(filename) if err != nil { return nil, err } defer f.Close() dec := gob.NewDecoder(f) var p Person if err := dec.Decode(&p); err != nil { return nil, err } return &p, nil }
Case 2: Transmitting serialized data over the network
Data serialization can also be used to transmit data over the network.
// 通过网络发送序列化数据 func SendOverNetwork(conn *net.Conn, p Person) error { enc := gob.NewEncoder(conn) return enc.Encode(p) } // 从网络接收反序列化数据 func ReceiveOverNetwork(conn *net.Conn) (*Person, error) { dec := gob.NewDecoder(conn) var p Person if err := dec.Decode(&p); err != nil { return nil, err } return &p, nil }
The above is the detailed content of Implement data serialization/deserialization in Golang using Gob. For more information, please follow other related articles on the PHP Chinese website!
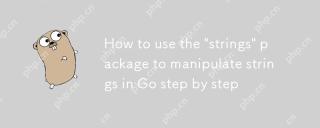
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
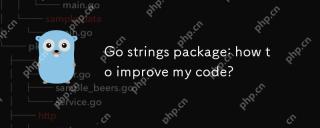
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
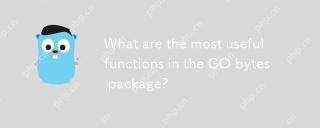
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
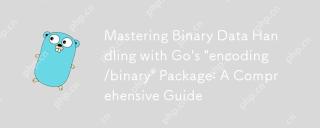
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary
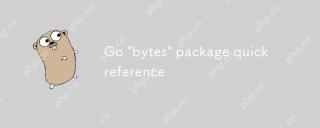
ThebytespackageinGoiscrucialforhandlingbyteslicesandbuffers,offeringtoolsforefficientmemorymanagementanddatamanipulation.1)Itprovidesfunctionalitieslikecreatingbuffers,comparingslices,andsearching/replacingwithinslices.2)Forlargedatasets,usingbytes.N
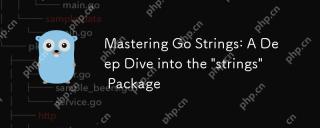
You should care about the "strings" package in Go because it provides tools for handling text data, splicing from basic strings to advanced regular expression matching. 1) The "strings" package provides efficient string operations, such as Join functions used to splice strings to avoid performance problems. 2) It contains advanced functions, such as the ContainsAny function, to check whether a string contains a specific character set. 3) The Replace function is used to replace substrings in a string, and attention should be paid to the replacement order and case sensitivity. 4) The Split function can split strings according to the separator and is often used for regular expression processing. 5) Performance needs to be considered when using, such as
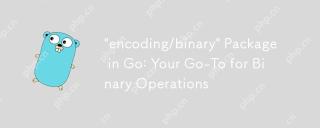
The"encoding/binary"packageinGoisessentialforhandlingbinarydata,offeringtoolsforreadingandwritingbinarydataefficiently.1)Itsupportsbothlittle-endianandbig-endianbyteorders,crucialforcross-systemcompatibility.2)Thepackageallowsworkingwithcus
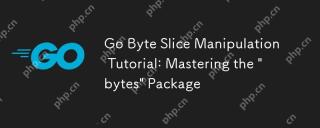
Mastering the bytes package in Go can help improve the efficiency and elegance of your code. 1) The bytes package is crucial for parsing binary data, processing network protocols, and memory management. 2) Use bytes.Buffer to gradually build byte slices. 3) The bytes package provides the functions of searching, replacing and segmenting byte slices. 4) The bytes.Reader type is suitable for reading data from byte slices, especially in I/O operations. 5) The bytes package works in collaboration with Go's garbage collector, improving the efficiency of big data processing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
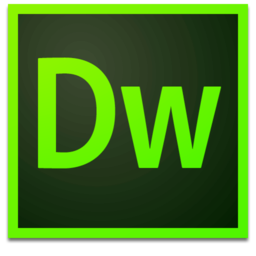
Dreamweaver Mac version
Visual web development tools
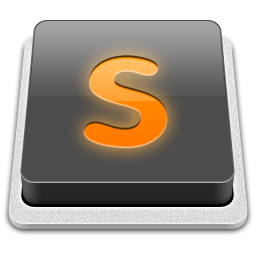
SublimeText3 Mac version
God-level code editing software (SublimeText3)
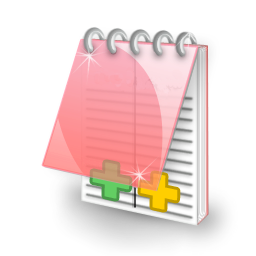
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
