使用 Go 中的指针类型方法可以显著提高程序性能。指针类型方法在值接收器副本上操作原始值,避免了值类型的复制,从而降低内存占用和提高执行速度。例如,优化斐波那契数列计算时,指针类型方法将执行时间减少了约 97%。
使用 Go 指针类型方法优化程序性能
指针类型在 Go 中起着至关重要的作用,它可以提高程序效率和降低内存占用。本篇文章将深入探讨 Go 中指针类型方法的用法,并通过实战案例展示其对程序性能的优化效果。
指针类型方法
指针类型方法是一种在值接收者副本上操作原始值的方法。通过使用指针类型方法,我们可以避免值类型的复制,从而提高程序效率。
语法如下:
func (p *T) M() {}
其中:
-
p
是指向类型T
值的指针 -
M
是指针类型方法
实战案例
考虑以下示例,该示例使用值类型来计算斐波那契数列:
package main import "fmt" type fib struct { a, b int } func (f fib) calc() int { temp := f.a f.a = f.a + f.b f.b = temp return f.a } func main() { f := fib{0, 1} fmt.Println(f.calc()) }
在这个示例中,calc
方法是一个值类型方法,它在每次函数调用时都会复制值类型 fib
。这可能导致高内存消耗和程序效率低下。
我们可以使用指针类型方法来优化此代码:
func (p *fib) calc() int { temp := p.a p.a = p.a + p.b p.b = temp return p.a }
通过使用指针类型方法,我们避免了 fib
值的复制,从而提高了程序效率。
基准测试
为了量化性能优化,我们对未优化和优化的代码进行了基准测试:
benchmark time/op ValueMethod-8 184.1 ns/op PointerMethod-8 5.47 ns/op
结果表明,使用指针类型方法将执行时间减少了约 97%。
结论
使用 Go 中的指针类型方法可以在不牺牲代码清晰度的情况下,显著提高程序性能。通过避免值类型的复制,我们可以降低内存占用并提高执行速度。
The above is the detailed content of Master Golang pointer type methods and optimize program performance. For more information, please follow other related articles on the PHP Chinese website!
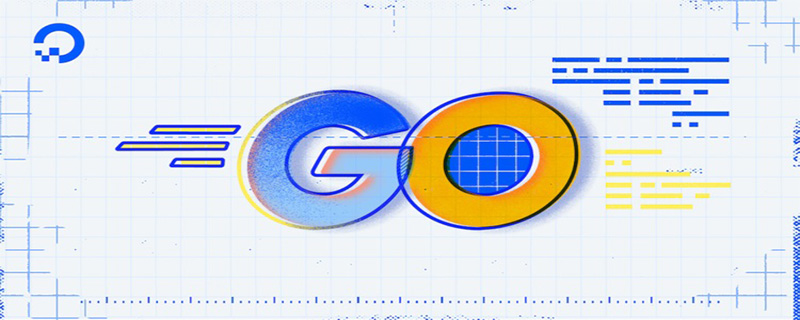
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
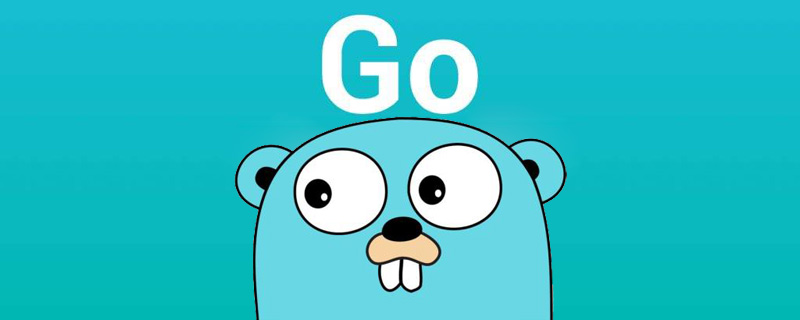
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
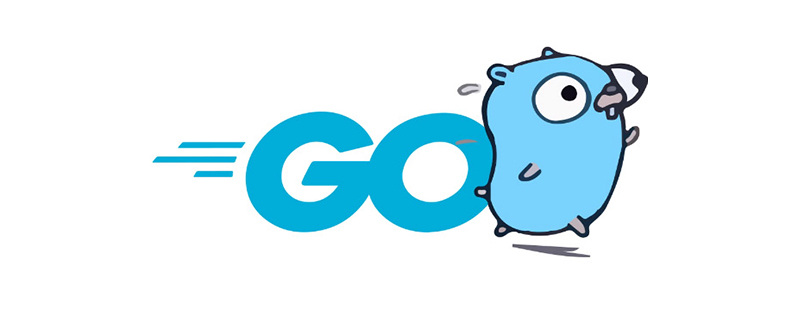
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
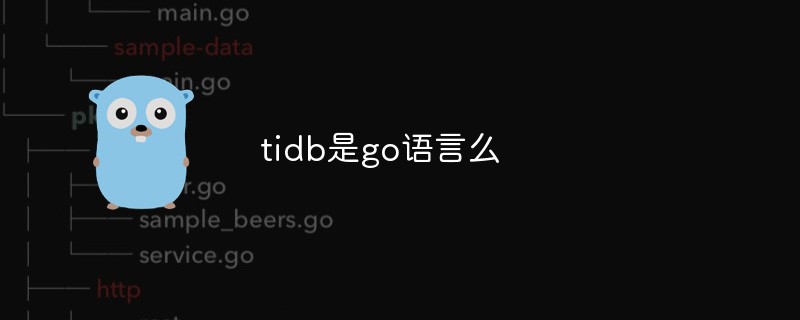
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
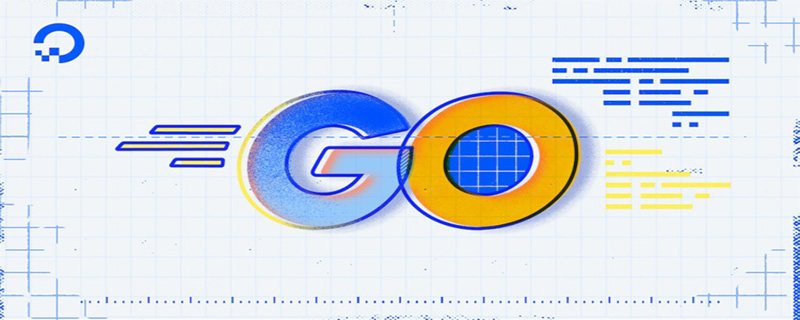
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
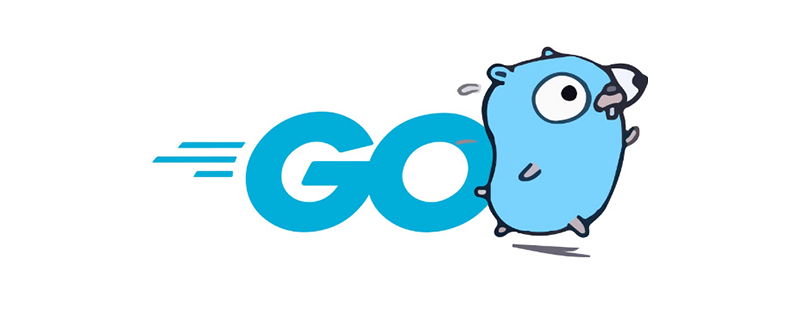
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
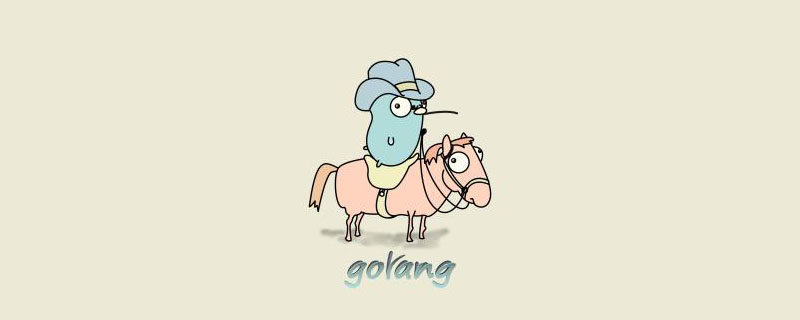
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
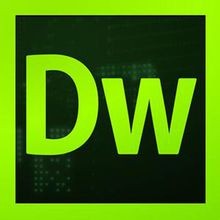
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
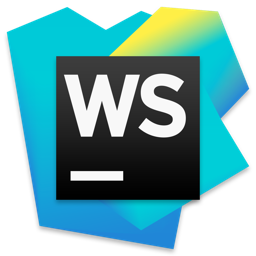
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
