Research on the application of Go language in network programming
Go 语言非常适合网络编程,本文概述了其应用,包括:建立 HTTP 服务器:使用 net/http 包创建简单的 HTTP 服务器。处理 HTTP 请求:使用 http.Handler 接口根据请求路径处理请求。创建 Websocket 服务器:利用 gorilla/websocket 库建立全双工通信的 Websocket 服务器。
Go 语言在网络编程中的应用探索
Go 语言以其高并发、易于使用和编译速度快等特点,使其非常适合网络编程。本文将探讨 Go 语言在网络编程中的应用,并通过实战案例进行演示。
建立 HTTP 服务器
Go 语言提供 net/http
包,可帮助你轻松地建立 HTTP 服务器。以下代码创建一个简单的 HTTP 服务器,监听端口 8080 并处理 GET 请求:
package main import ( "fmt" "log" "net/http" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, world!") }) log.Fatal(http.ListenAndServe(":8080", nil)) }
处理 HTTP 请求
可以使用 http.Handler
接口来处理 HTTP 请求。http.Handler
只是一个具有 ServeHTTP
方法的接口,该方法接受 http.ResponseWriter
和 *http.Request
作为参数,用于处理请求并写入响应。
以下代码使用 http.Handler
来处理 HTTP 请求,并根据请求的路径返回不同的响应:
package main import ( "fmt" "net/http" ) type MyHandler struct{} func (h *MyHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) { switch r.URL.Path { case "/": fmt.Fprint(w, "Hello, world!") case "/about": fmt.Fprint(w, "About page") default: fmt.Fprint(w, "404 Not Found") } } func main() { handler := &MyHandler{} http.Handle("/", handler) http.ListenAndServe(":8080", nil) }
创建 Websocket 服务器
Go 语言还可以使用 gorilla/websocket
库建立 Websocket 服务器。Websocket 是一种基于 TCP 的协议,允许客户端与服务器进行全双工通信。
以下代码创建一个简单的 Websocket 服务器,监听端口 8080 并处理 Websocket 连接:
package main import ( "log" "net/http" "github.com/gorilla/websocket" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { conn, err := websocket.Upgrade(w, r, nil, 1024, 1024) if err != nil { log.Fatal(err) } go echo(conn) }) log.Fatal(http.ListenAndServe(":8080", nil)) } func echo(conn *websocket.Conn) { for { messageType, message, err := conn.ReadMessage() if err != nil { log.Fatal(err) return } if err := conn.WriteMessage(messageType, message); err != nil { log.Fatal(err) return } } }
以上只是一些 Go 语言在网络编程中的应用示例。Go 语言还提供其他网络编程特性,如 TCP 套接字、UDP 套接字和 HTTP 客户端等。这些功能使 Go 语言能够构建各种网络应用程序,从简单的 HTTP 服务器到复杂的分布式系统。
The above is the detailed content of Research on the application of Go language in network programming. For more information, please follow other related articles on the PHP Chinese website!
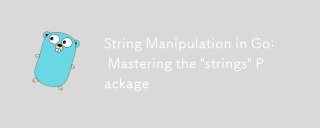
Mastering the strings package in Go language can improve text processing capabilities and development efficiency. 1) Use the Contains function to check substrings, 2) Use the Index function to find the substring position, 3) Join function efficiently splice string slices, 4) Replace function to replace substrings. Be careful to avoid common errors, such as not checking for empty strings and large string operation performance issues.
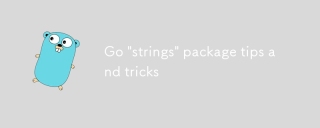
You should care about the strings package in Go because it simplifies string manipulation and makes the code clearer and more efficient. 1) Use strings.Join to efficiently splice strings; 2) Use strings.Fields to divide strings by blank characters; 3) Find substring positions through strings.Index and strings.LastIndex; 4) Use strings.ReplaceAll to replace strings; 5) Use strings.Builder to efficiently splice strings; 6) Always verify input to avoid unexpected results.
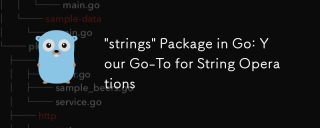
ThestringspackageinGoisessentialforefficientstringmanipulation.1)Itofferssimpleyetpowerfulfunctionsfortaskslikecheckingsubstringsandjoiningstrings.2)IthandlesUnicodewell,withfunctionslikestrings.Fieldsforwhitespace-separatedvalues.3)Forperformance,st
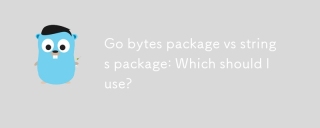
WhendecidingbetweenGo'sbytespackageandstringspackage,usebytes.Bufferforbinarydataandstrings.Builderforstringoperations.1)Usebytes.Bufferforworkingwithbyteslices,binarydata,appendingdifferentdatatypes,andwritingtoio.Writer.2)Usestrings.Builderforstrin
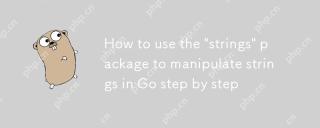
Go's strings package provides a variety of string manipulation functions. 1) Use strings.Contains to check substrings. 2) Use strings.Split to split the string into substring slices. 3) Merge strings through strings.Join. 4) Use strings.TrimSpace or strings.Trim to remove blanks or specified characters at the beginning and end of a string. 5) Replace all specified substrings with strings.ReplaceAll. 6) Use strings.HasPrefix or strings.HasSuffix to check the prefix or suffix of the string.
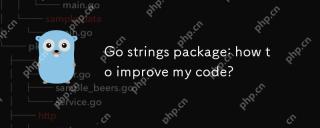
Using the Go language strings package can improve code quality. 1) Use strings.Join() to elegantly connect string arrays to avoid performance overhead. 2) Combine strings.Split() and strings.Contains() to process text and pay attention to case sensitivity issues. 3) Avoid abuse of strings.Replace() and consider using regular expressions for a large number of substitutions. 4) Use strings.Builder to improve the performance of frequently splicing strings.
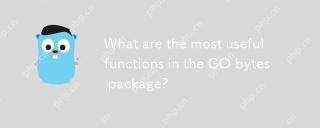
Go's bytes package provides a variety of practical functions to handle byte slicing. 1.bytes.Contains is used to check whether the byte slice contains a specific sequence. 2.bytes.Split is used to split byte slices into smallerpieces. 3.bytes.Join is used to concatenate multiple byte slices into one. 4.bytes.TrimSpace is used to remove the front and back blanks of byte slices. 5.bytes.Equal is used to compare whether two byte slices are equal. 6.bytes.Index is used to find the starting index of sub-slices in largerslices.
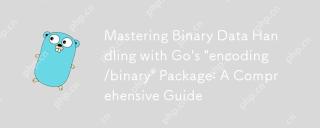
Theencoding/binarypackageinGoisessentialbecauseitprovidesastandardizedwaytoreadandwritebinarydata,ensuringcross-platformcompatibilityandhandlingdifferentendianness.ItoffersfunctionslikeRead,Write,ReadUvarint,andWriteUvarintforprecisecontroloverbinary


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
