In Go, object-oriented programming is implemented using structures and methods: define a structure to represent a custom data type and contain data fields. Define methods to manipulate and modify structure instances. Methods are associated by the receiver type (that is, the structure). Use structures and methods to manage data, such as creating instances, accessing information, and modifying data.
Object-oriented programming practice in Go language
In Go language, object-oriented programming (OOP) uses structures and methods to fulfill. This article will guide you in using Go language for OOP programming and deepen your understanding through practical cases.
Structure
Structure is a mechanism for defining custom data types in the Go language. It contains a set of data fields of the same type. Create a structure as shown below:
type Person struct { name string age int }
This structure defines a type named Person
, which contains two fields: name
(String ) and age
(integer type).
Methods
Methods are functions associated by the receiver type (i.e. structure). They are used to manipulate and modify structure instances. Define a method for the structure as follows:
func (p *Person) Greet() { fmt.Printf("你好,我的名字是 %s\n", p.name) }
This method receives a Person
structure pointer p
as a parameter and uses p
Access structure fields. It prints a welcome message with the value of the name
field of the structure.
Practical Case: Employee Management
Now, let’s take a look at a practical case to show how to use OOP to manage employee information in Go.
// 定义员工结构体 type Employee struct { name string salary int manager *Employee // 指向经理的指针 } // 为员工结构体定义方法 func (e *Employee) GetSalary() int { return e.salary } func (e *Employee) Promote(salary int) { e.salary = salary } func main() { // 创建员工实例 ceo := Employee{name: "Tim", salary: 100000} manager := Employee{name: "Bob", salary: 50000, manager: &ceo} employee := Employee{name: "John", salary: 20000, manager: &manager} // 访问员工信息 fmt.Printf("CEO 的薪水:%d\n", ceo.GetSalary()) fmt.Printf("经理的薪水:%d\n", manager.GetSalary()) fmt.Printf("员工的薪水:%d\n", employee.GetSalary()) // 提升经理的薪水 manager.Promote(60000) fmt.Printf("经理的新薪水:%d\n", manager.GetSalary()) }
In this example, we create three employee instances: CEO, manager, and employee. Each employee has a name
and salary
field, and the manager also has a pointer to their manager
. We also defined methods for the structure to obtain and modify the employee's salary.
In the main
function, we create these employee instances and access their information. We also show how to increase a manager's salary and print out his new salary.
The above is the detailed content of Object-oriented programming practice in Go language. For more information, please follow other related articles on the PHP Chinese website!
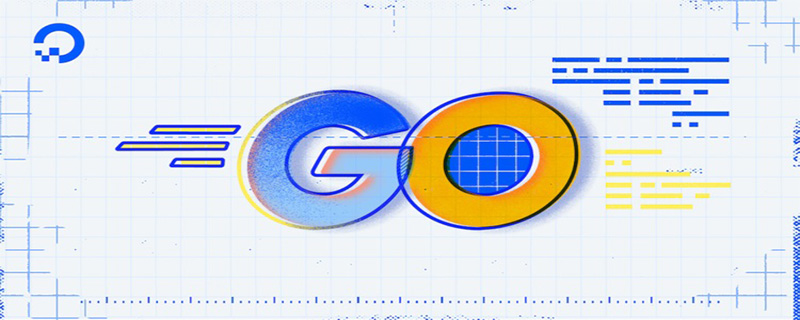
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
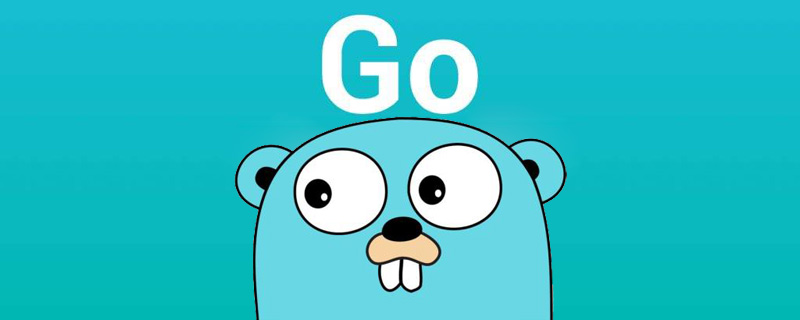
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
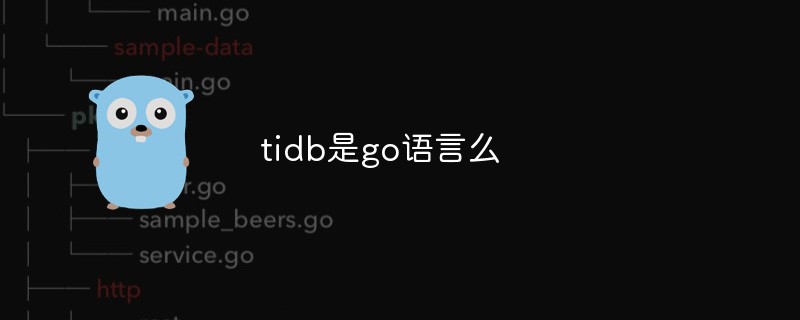
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
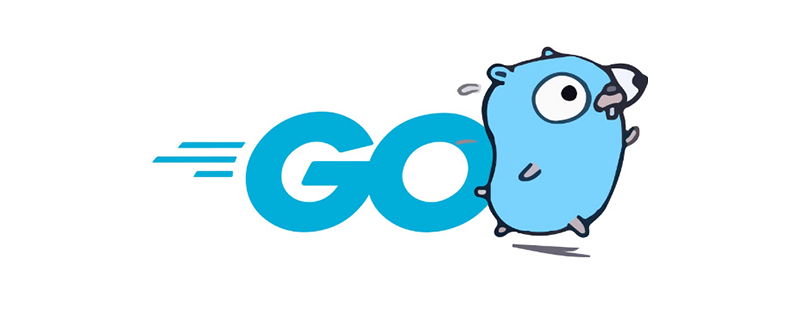
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
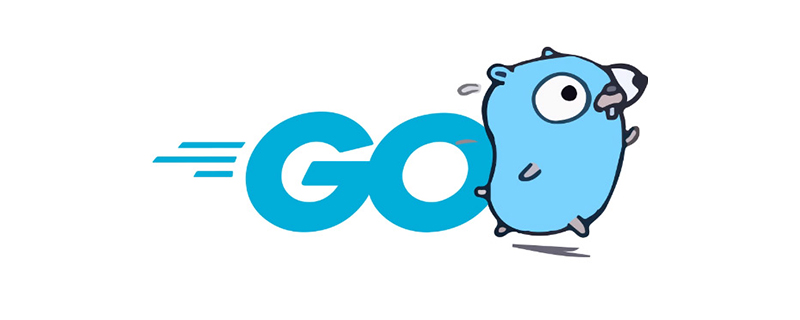
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
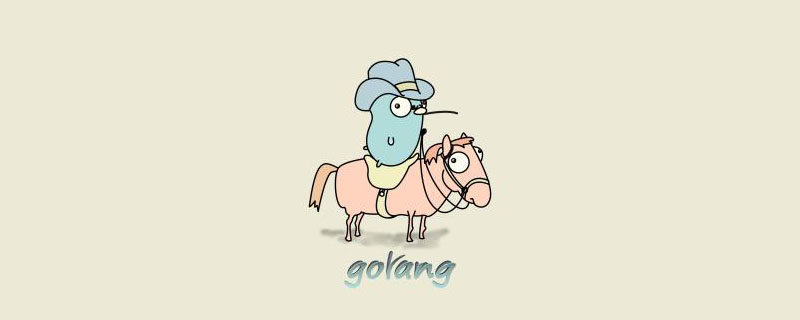
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
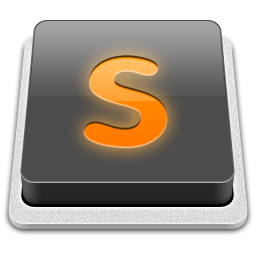
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.