Concurrent programming in Go is implemented using goroutine (lightweight thread) and channel (communication pipe). Goroutine is created through the go keyword, which is very lightweight and can create a large number of goroutines without affecting performance. Channel is used for communication between goroutines and is a typed pipe. This example shows the application of concurrent crawlers, using goroutine to crawl URLs in parallel to improve efficiency.
Go core technology analysis: concurrent programming
The concurrency model in Go is based on goroutine (lightweight thread) and channel ( communication pipeline) concept. By taking full advantage of these features, developers can build highly concurrent, high-performance applications.
Goroutine
Goroutine is a lightweight thread in Go, created by the keyword go
. They run on a coroutine scheduler, and unlike traditional threads, goroutines are very lightweight and thousands can be created without significant performance impact. The following code shows how to create and use a goroutine:
package main import ( "fmt" "runtime" ) func main() { fmt.Printf("Number of goroutines: %d\n", runtime.NumGoroutine()) // 当前正在运行的 goroutine 数量 // 创建一个 goroutine go func() { fmt.Println("Hello from a goroutine!") }() fmt.Printf("Number of goroutines: %d\n", runtime.NumGoroutine()) // 当前正在运行的 goroutine 数量 }
Channel
Channel is a pipe used for communication between goroutines. They are typed, which means they can only pass values of specific types. The following code shows how to create and use channels:
package main import ( "fmt" ) func main() { // 创建一个 int 类型的 channel c := make(chan int) // 向 channel 发送值 go func() { c <- 42 }() // 从 channel 接收值 v := <-c fmt.Println(v) // 输出:42 }
Practical case: concurrent crawler
The following is a simplified example of using goroutine and channel to build a concurrent crawler:
package main import ( "fmt" "net/http" "sync" ) var wg sync.WaitGroup func main() { // 要爬取的 URL 列表 urls := []string{ "https://example.com", "https://example2.com", "https://example3.com", } // 创建 channel 来接收每个 URL 的响应 results := make(chan string) // 为每个 URL 创建一个 goroutine for _, url := range urls { wg.Add(1) go func(url string) { resp, err := http.Get(url) if err != nil { fmt.Printf("Error fetching %s: %v\n", url, err) } else if resp.StatusCode == 200 { results <- url } wg.Done() }(url) } // 从 channel 中获取响应 go func() { for url := range results { fmt.Println(url) } }() // 等待所有 goroutine 完成 wg.Wait() }
By using goroutine and channel, this crawler can crawl multiple URLs concurrently, thus improving efficiency.
The above is the detailed content of Go language core technology analysis. For more information, please follow other related articles on the PHP Chinese website!
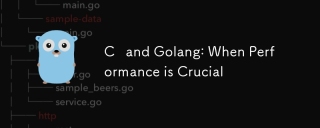
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
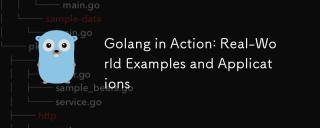
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
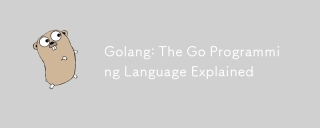
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
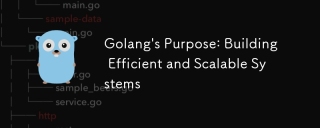
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
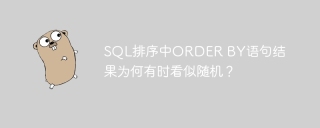
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
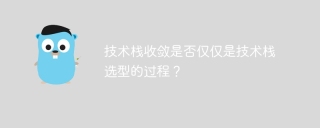
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
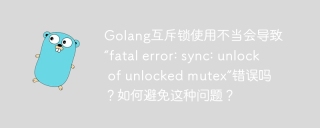
Golang ...
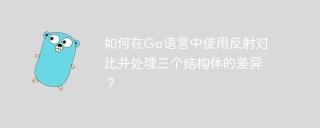
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!
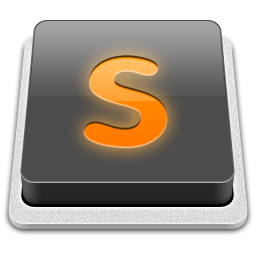
SublimeText3 Mac version
God-level code editing software (SublimeText3)