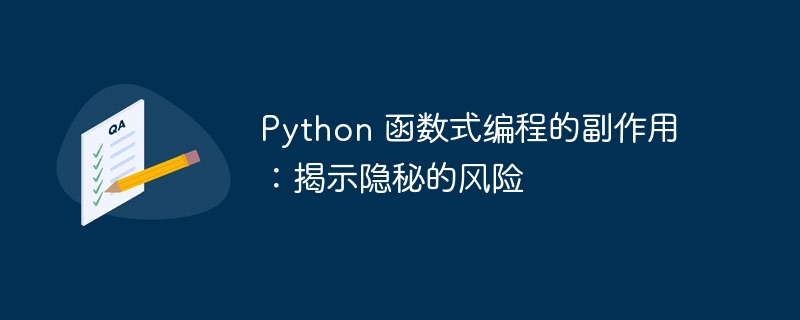
Functional Programming is becoming increasingly popular in python because it provides a way to improve code clarity and maintainability. However, when embracing functional programming, it is crucial to understand its inherent risks, especially side effects. This article will delve into Python side effects in functional programming, reveal their hidden dangers and provide mitigation strategies.
What are side effects?
Side effects refer to the behavior of a function that changes the external state of the function during execution. This might include modifying global variables, adding elements to a list or dictionary, or performing other external operations.
Risk of side effects
-
Difficult to debug: Debugging code becomes difficult when side effects are present because state changes can lead to unexpected behavior that is difficult to track.
-
Concurrency issues: If multiple threads call functions with side effects at the same time, it may lead to race conditions and data inconsistencies.
-
Testing Difficulty: Functions with side effects are difficult to test because they rely on external state, which can make it difficult to create repeatable test cases.
-
Limited Reusability: Functions with side effects have poor reusability because they rely on specific state, which may cause conflicts with other pieces of code.
Common impacts in Python
-
Modify global variables: Functions may modify global variables through assignment or reference, resulting in unexpected consequences.
-
Modify a list or dictionary: A function can modify the list or dictionary passed to it, causing unexpected interactions with the calling code.
-
File I/O operations: Functions may perform file I/O operations such as opening, writing, or closing files, which may introduce side effects such as file handle loss.
Mitigation Strategies
-
Avoid side effects: Avoid using side effects in functional code whenever possible. Write pure functions that do not depend on external state and do not change external state.
-
Handle side effects explicitly: If side effects are required, handle them explicitly and separate them from the business logic. Use explicit function parameters or return values to pass and return external state changes.
-
Use immutable objects: Use immutable objects such as tuples or frozen dictionaries whenever possible to prevent accidental modification.
-
Use local variables: Declare local variables to store any state required by the function instead of modifying global variables or external objects.
-
Test for side effects: Carefully test functions with side effects, considering all possible external states and interactions. Use mocking or isolation techniques to isolate side effects and test their expected behavior.
in conclusion
Python Functional programming is a powerful tool, but understanding its inherent risks, especially side effects, is critical. By avoiding side effects, handling them explicitly, and using appropriate mitigation strategies, developers can minimize risks and create reliable, maintainable functional code.
The above is the detailed content of Side Effects of Python Functional Programming: Revealing Hidden Risks. For more information, please follow other related articles on the PHP Chinese website!