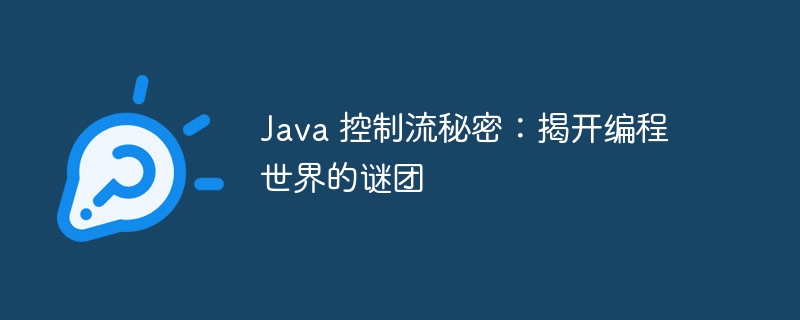
Conditional statements
Conditional statements are used to execute a specific block of code based on given conditions. There are two main types of conditional statements in Java:
-
if statement: If the given condition is true, the code block in the if statement is executed.
-
switch statement: Execute different code blocks based on the value of a variable.
loop statement
Loop statements allow developers to repeatedly execute a block of code until a certain condition is met. There are three main types of loop statements in Java:
-
for loop: Execute the code block a certain number of times.
-
while loop: Execute the code block as long as the given condition is true.
-
do-while loop: Execute the code block at least once, and then continue execution as long as the given condition is true.
Branch Control
Branch control allows developers to change program flow based on conditions. There are the following branch control statements in Java:
-
break: Exit the current loop or switch statement immediately.
-
continue: Skip the remainder of the current loop and go to the next iteration.
-
return: Return from the method, optionally returning a value.
Exception handling
Exception handling is used to handle errors that occur during program execution. There are the following exception handling structures in Java:
-
try-catch-finally: Try to execute a block of code (try), and catch and handle an exception when it occurs (catch). Regardless of whether an exception occurs or no exception occurs, the code in the finally block is eventually executed.
-
throws: Indicates that the method can throw the specified exception type.
Other control flow elements
Java also provides other control flow elements, such as:
-
Tags: Allows the use of tags to jump to specific locations in the code.
-
Assertion: Used to check whether the hypothesis is true. If the assertion fails, an exception is thrown.
-
Concurrency Control: Allows developers to control the execution of concurrent code, such as synchronization and locks .
Best Practices
When using control flow, following the following best practices will help ensure the readability and maintainability of your code:
- Use clear and understandable conditional statements.
- Use appropriate loop statements to maximize efficiency.
- Use branch control correctly to avoid unexpected behavior.
- Handle exceptions correctly to ensure application robustness.
-
Test all control flow paths to verify correct behavior.
With a deep understanding of Java control flow, developers can write more efficient and flexible programs. These concepts are essential tools for any developer in the programming world.
The above is the detailed content of Java Control Flow Secrets: Uncovering the Mysteries of the Programming World. For more information, please follow other related articles on the PHP Chinese website!