Exploration on the memory management mechanism of Go language
As a modern programming language, Go language has attracted much attention for its efficient concurrency performance and convenient programming model. Among them, memory management is one of the important factors for its performance advantages. This article will delve into the memory management mechanism of the Go language and demonstrate its working principle through specific code examples.
Memory allocation and recycling
The memory management of the Go language uses a mechanism called "automatic garbage collection (GC)", that is, programmers do not need to manually manage the allocation and recycling of memory. This is automatically taken care of by the runtime system. In Go language, use make
and new
to allocate memory.
Use make
for memory allocation
make
The function is used to create objects of slice, map and channel types, and returns an initialized Example. The usage of the make
function is as follows:
slice := make([]int, 0, 10) m := make(map[string]int) ch := make(chan int)
In the above example, an empty slice, an empty map and an int type are created through the make
function. channel.
Use new
for memory allocation
new
The function is used to allocate memory space for a type and return a pointer of the type. new
The usage example of the function is as follows:
var i *int i = new(int)
In the above example, the memory space is allocated for the int type through the new
function and assigned to the pointeri
.
Garbage Collection (GC) Algorithm
The garbage collection algorithm of Go language adopts an algorithm based on mark and sweep. When an object is no longer referenced, the GC reclaims memory by marking all reachable objects and clearing unmarked objects.
The following is a simple example that demonstrates the garbage collection process:
package main import "fmt" type Node struct { data int next *Node } func main() { var a, b, c Node a.data = 1 b.data = 2 c.data = 3 a.next = &b b.next = &c c.next = nil // 断开a对b的引用 a.next = nil // 垃圾回收 fmt.Println("垃圾回收前:", a, b, c) // 手动触发垃圾回收 // runtime.GC() fmt.Println("垃圾回收后:", a, b, c) }
In the above example, when a.next
is assigned the value nil
, the reference of a to b is disconnected. At this time, the b object becomes an unreachable object and will be recycled by the garbage collector.
Handling of memory leaks
Although the Go language has an automatic garbage collection mechanism, memory leaks may still occur. A memory leak refers to a situation where the memory allocated in a program is not released correctly, resulting in excessive memory usage.
The following is a sample code that may cause a memory leak:
package main import ( "fmt" "time" ) func leakyFunc() { data := make([]int, 10000) // do something with data } func main() { for { leakyFunc() time.Sleep(time.Second) } }
In the above example, an int array with a length of 10000 is created in the leakyFunc
function, but The memory space occupied by the array is not released. If the leakyFunc
function is called frequently in a loop, memory leaks will occur.
In order to avoid memory leaks, developers should pay attention to promptly releasing memory resources that are no longer needed. They can reduce memory usage through defer
statements, reasonable use of cache pools, etc.
Summary
This article explores the memory management mechanism of Go language from the aspects of memory allocation, garbage collection algorithm and memory leak processing. By having an in-depth understanding of the memory management of the Go language, developers can better understand the memory usage of the program during runtime, avoid problems such as memory leaks, and improve the performance and stability of the program. Hope this article can be helpful to readers.
The above is the detailed content of Research on the memory management mechanism of Go language. For more information, please follow other related articles on the PHP Chinese website!
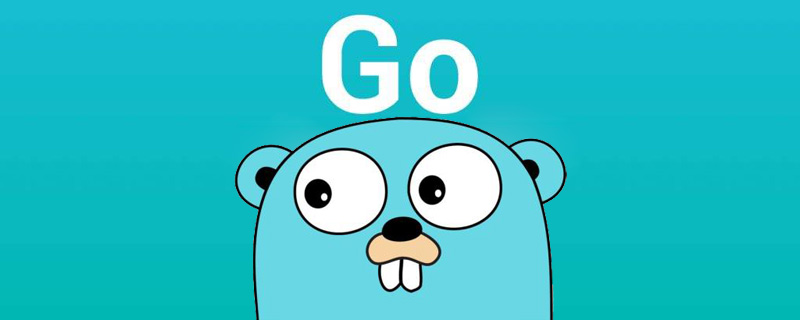
golang是一种静态强类型、编译型、并发型,并具有垃圾回收功能的编程语言;它可以在不损失应用程序性能的情况下极大的降低代码的复杂性,还可以发挥多核处理器同步多工的优点,并可解决面向对象程序设计的麻烦,并帮助程序设计师处理琐碎但重要的内存管理问题。
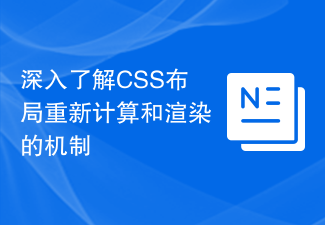
CSS回流(reflow)和重绘(repaint)是网页性能优化中非常重要的概念。在开发网页时,了解这两个概念的工作原理,可以帮助我们提高网页的响应速度和用户体验。本文将深入探讨CSS回流和重绘的机制,并提供具体的代码示例。一、CSS回流(reflow)是什么?当DOM结构中的元素发生可视性、尺寸或位置改变时,浏览器需要重新计算并应用CSS样式,然后重新布局
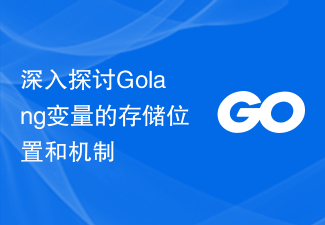
标题:深入探讨Golang变量的存储位置和机制随着Go语言(Golang)在云计算、大数据和人工智能领域的应用逐渐增多,深入了解Golang变量的存储位置和机制变得尤为重要。在本文中,我们将详细探讨Golang中变量的内存分配、存储位置以及相关的机制。通过具体代码示例,帮助读者更好地理解Golang变量在内存中是如何存储和管理的。1.Golang变量的内存
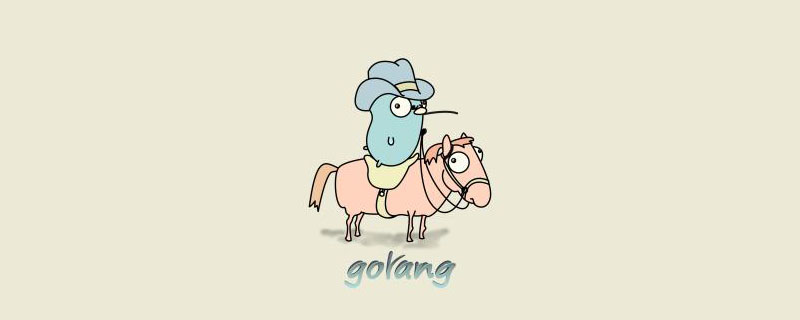
go语言有gc。GC是指垃圾回收,是一种自动内存管理的机制;go语言支持GC,Go语言中对象内存空间的回收是通过GC机制来完成的。对于Go语言而言,Go语言的GC使用的是无分代(对象没有代际之分)、不整理(回收过程中不对对象进行移动与整理)、并发(与用户代码并发执行)的三色标记清扫算法。
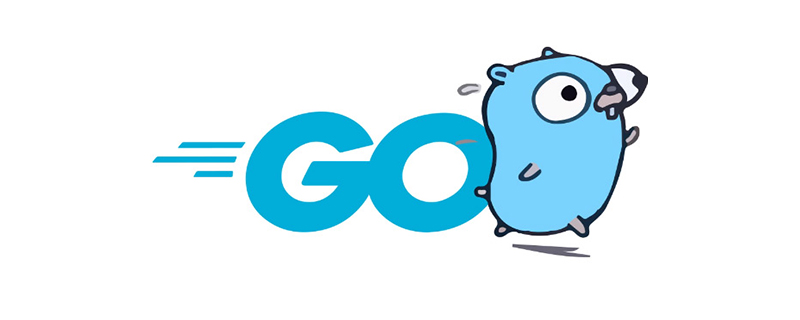
在go语言中,goto语句用于无条件跳转,可以无条件地转移到程序中指定的行;它通过标签进行代码间的无条件跳转。goto后接一个标签,这个标签的意义是告诉Go程序下一步要执行哪行的代码,语法“goto 标签;... ...标签: 表达式;”。goto打破原有代码执行顺序,直接跳转到指定行执行代码;goto语句通常与条件语句配合使用,可用来实现条件转移、构成循环、跳出循环体等功能。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
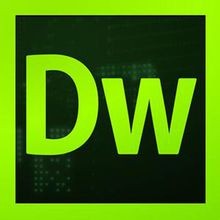
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
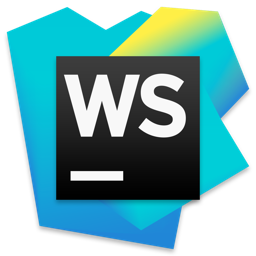
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
