Explore the disadvantages and solutions of Go language
Disadvantages of Go language and solutions
As a fast, reliable and efficient programming language, Go language has been popular in recent years. The Internet field has been widely used and recognized. However, just like any other programming language, Go has some disadvantages that may present some challenges for some developers. This article will explore the disadvantages of the Go language and propose ways to solve these disadvantages, along with some specific code examples to help readers better understand.
1. Lack of generic support
As a statically typed language, Go language has weak support for generics, which makes it a bit clumsy when dealing with some common data structures. Frequently perform type assertions or use interface{} to achieve generic effects.
Solution: Generic functions can be simulated through interfaces, reflection or code generation. Next, a simple example will be used to illustrate how to use the interface to implement a general slice filter function.
package main import ( "fmt" ) type FilterFunc func(int) bool func Filter(slice []int, filter FilterFunc) []int { var result []int for _, v := range slice { if filter(v) { result = append(result, v) } } return result } func main() { slice := []int{1, 2, 3, 4, 5, 6} evenFilter := func(num int) bool { return num%2 == 0 } evenNumbers := Filter(slice, evenFilter) fmt.Println(evenNumbers) // Output: [2 4 6] }
2. Exception handling is more cumbersome
The error handling mechanism in Go language adopts an explicit method and needs to transfer error information through return values, which makes writing a large amount of error handling The code seems redundant and cumbersome.
Solution: You can simplify the exception handling process by using keywords such as defer, panic, and recover. The following is a simple example to demonstrate how to use panic and recover to handle exceptions.
package main import ( "fmt" ) func Divide(a, b int) int { defer func() { if err := recover(); err != nil { fmt.Println("发生了除零错误:", err) } }() if b == 0 { panic("除数不能为零") } return a / b } func main() { result := Divide(6, 3) fmt.Println("结果:", result) result = Divide(8, 0) fmt.Println("结果:", result) }
3. Performance optimization needs to be handled with caution
Although the Go language is famous for its efficient concurrency model and garbage collector, in some specific scenarios, performance optimization still requires developers to Be careful as over-optimization may lead to reduced code readability and maintainability.
Solution: When optimizing performance, it is necessary to analyze and test according to specific scenarios to avoid premature optimization and excessive optimization. In the following example, we show how to use Go's pprof tool for performance analysis.
package main import ( "fmt" "log" "net/http" _ "net/http/pprof" ) func fib(n int) int { if n <= 1 { return n } return fib(n-1) + fib(n-2) } func main() { go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }() fmt.Println("服务器已启动,请访问http://localhost:6060/debug/pprof/进行性能分析") result := fib(30) fmt.Println("斐波那契数列第30项:", result) }
Through the above examples, we have demonstrated some disadvantages and solutions of the Go language, and provided specific code examples to help readers better understand. As with any programming language, mastering its strengths and weaknesses and finding appropriate solutions are essential skills to become a good developer. I hope this article is helpful to you, thank you for reading!
The above is the detailed content of Explore the disadvantages and solutions of Go language. For more information, please follow other related articles on the PHP Chinese website!
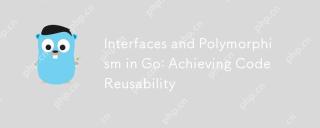
InterfacesandpolymorphisminGoenhancecodereusabilityandmaintainability.1)Defineinterfacesattherightabstractionlevel.2)Useinterfacesfordependencyinjection.3)Profilecodetomanageperformanceimpacts.
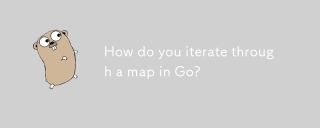
Article discusses iterating through maps in Go, focusing on safe practices, modifying entries, and performance considerations for large maps.Main issue: Ensuring safe and efficient map iteration in Go, especially in concurrent environments and with l
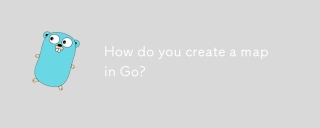
The article discusses creating and manipulating maps in Go, including initialization methods and adding/updating elements.
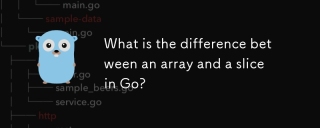
The article discusses differences between arrays and slices in Go, focusing on size, memory allocation, function passing, and usage scenarios. Arrays are fixed-size, stack-allocated, while slices are dynamic, often heap-allocated, and more flexible.
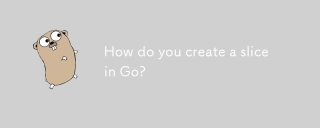
The article discusses creating and initializing slices in Go, including using literals, the make function, and slicing existing arrays or slices. It also covers slice syntax and determining slice length and capacity.
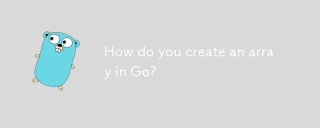
The article explains how to create and initialize arrays in Go, discusses the differences between arrays and slices, and addresses the maximum size limit for arrays. Arrays vs. slices: fixed vs. dynamic, value vs. reference types.
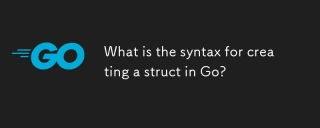
Article discusses syntax and initialization of structs in Go, including field naming rules and struct embedding. Main issue: how to effectively use structs in Go programming.(Characters: 159)
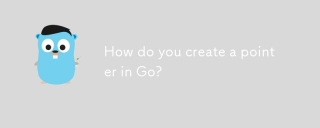
The article explains creating and using pointers in Go, discussing benefits like efficient memory use and safe management practices. Main issue: safe pointer use.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
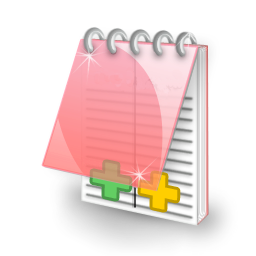
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use
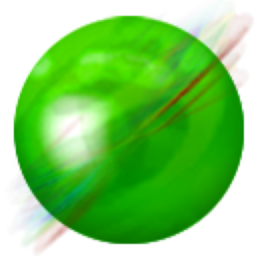
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
