


In-depth understanding of the similarities and differences between C++ and C languages
C and C are two popular programming languages that are similar in many ways, but also have many significant differences. This article will take an in-depth look at the similarities and differences between C and the C language, and illustrate their differences through specific code examples.
1. Basic syntax and structural differences
1.1 Data type definition
In C language, when defining a variable, you need to declare the data type first, for example:
int num;
In C, variables can be initialized while defining them, for example:
int num = 10;
1.2 Function definition and call
In C language, function definition and call are done separately, for example :
void sayHello() { printf("Hello"); } int main() { sayHello(); return 0; }
In C, function definitions and calls can be put together, for example:
void sayHello() { cout << "Hello"; } int main() { sayHello(); return 0; }
1.3 Namespace
There is no concept of namespace in the C language, and Namespaces can be used in C to avoid naming conflicts, for example:
namespace myNamespace { int num = 10; }
2. Similarities and Differences in Object-Oriented Programming
2.1 Classes and Objects
C is an object-oriented The programming language supports the concepts of classes and objects, and the properties and methods of objects can be defined through classes, for example:
class Car { public: string brand; int price; void display() { cout << "Brand: " << brand << ", Price: " << price; } }; int main() { Car myCar; myCar.brand = "Toyota"; myCar.price = 20000; myCar.display(); return 0; }
In C language, the concepts of classes and objects are not directly supported, and structures are needed. bodies and functions to simulate, for example:
typedef struct { char brand[20]; int price; } Car; void display(Car *car) { printf("Brand: %s, Price: %d", car->brand, car->price); } int main() { Car myCar = {"Toyota", 20000}; display(&myCar); return 0; }
2.2 Inheritance and polymorphism
C supports the characteristics of inheritance and polymorphism, and can inherit the properties and methods of the base class through derived classes, and can be implemented Runtime polymorphism, for example:
class Animal { public: virtual void sound() { cout << "Some sound"; } }; class Dog : public Animal { public: void sound() override { cout << "Woof"; } };
In C language, there is no direct support, and polymorphism needs to be simulated through function pointers and other methods.
3. Code example display
The following is a simple C program example that implements a simple calculator function:
#include <iostream> using namespace std; int add(int a, int b) { return a + b; } int subtract(int a, int b) { return a - b; } int multiply(int a, int b) { return a * b; } int divide(int a, int b) { if (b == 0) { cout << "Error: Cannot divide by zero"; return 0; } return a / b; } int main() { int a = 10, b = 5; cout << "Addition: " << add(a, b) << endl; cout << "Subtraction: " << subtract(a, b) << endl; cout << "Multiplication: " << multiply(a, b) << endl; cout << "Division: " << divide(a, b) << endl; return 0; }
Through the above understanding of C and C language With an in-depth understanding of the similarities and differences, you can better choose the appropriate programming language to complete your programming tasks. Each has its own advantages and applicable scenarios. Choosing the right language will help improve programming efficiency and code quality.
The above is the detailed content of In-depth understanding of the similarities and differences between C++ and C languages. For more information, please follow other related articles on the PHP Chinese website!
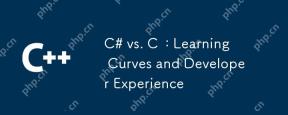
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
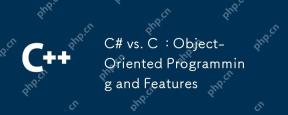
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
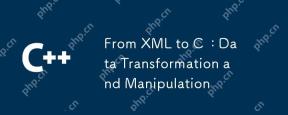
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
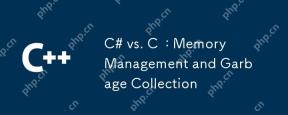
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
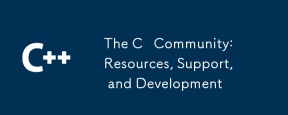
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
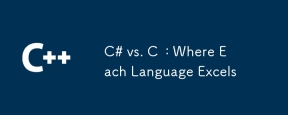
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
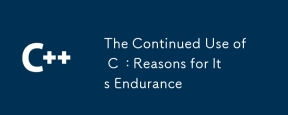
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor
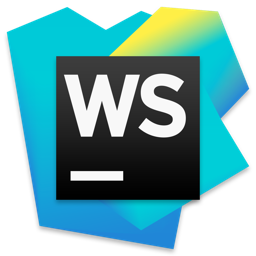
WebStorm Mac version
Useful JavaScript development tools
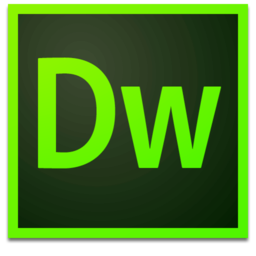
Dreamweaver Mac version
Visual web development tools
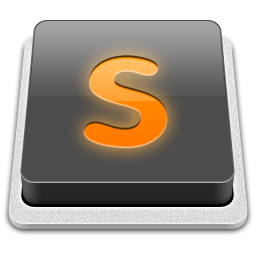
SublimeText3 Mac version
God-level code editing software (SublimeText3)