Go language is an open source programming language developed by Google. It has the advantages of concurrent programming, in which blocking is a common programming pattern. This article will explore the principles and applications of blocking in the Go language, and give specific code examples to help readers better understand and apply blocking-related concepts.
Principle
In the Go language, blocking means that a goroutine (coroutine) is suspended while waiting for the completion of an operation, and then continues execution until the operation is completed. This mechanism can effectively manage concurrently executing goroutines and avoid resource competition and data inconsistency problems.
The most common blocking operation in the Go language is the sending and receiving of channels. When a goroutine tries to send data to a full channel, the goroutine will be blocked; conversely, when a goroutine tries to receive data from an empty channel, it will also be blocked. Through the blocking feature of the channel, synchronization and collaboration between multiple goroutines can be achieved.
Application
1. Use channels to achieve blocking synchronization
package main import "fmt" func worker(ch chan int) { data := <-ch fmt.Println("Received data:", data) } func main() { ch := make(chan int) go worker(ch) ch <- 42 // 向通道发送数据 fmt.Println("Sent data: 42") }
In the above example, the main goroutine will send data 42 to the channel, and the worker goroutine will receive data from the channel and print. Since the channel is blocked, the main goroutine will not continue execution until the worker receives the data.
2. Use select statements to handle multiple blocking operations
package main import ( "fmt" "time" ) func foo(ch1, ch2 chan int) { time.Sleep(2 * time.Second) ch1 <- 1 } func bar(ch1, ch2 chan int) { time.Sleep(1 * time.Second) ch2 <- 2 } func main() { ch1 := make(chan int) ch2 := make(chan int) go foo(ch1, ch2) go bar(ch1, ch2) select { case data := <-ch1: fmt.Println("Received data from foo:", data) case data := <-ch2: fmt.Println("Received data from bar:", data) } }
In the above example, blocking operations on multiple channels can be processed through the select statement, as long as one of the channels has readable data. The select statement will select and execute the corresponding case statement.
Conclusion
Through the above examples, we can see how blocking is used in the Go language to implement synchronous and asynchronous operations in concurrent programming. Blocking is an important feature of the Go language concurrency model. Proper use of blocking can simplify the complexity of concurrent programming and improve the maintainability and stability of the code. I hope this article can help readers gain a deeper understanding of the principles and applications of blocking in the Go language.
The above is the detailed content of Learn the principles and applications of blocking in Go language. For more information, please follow other related articles on the PHP Chinese website!
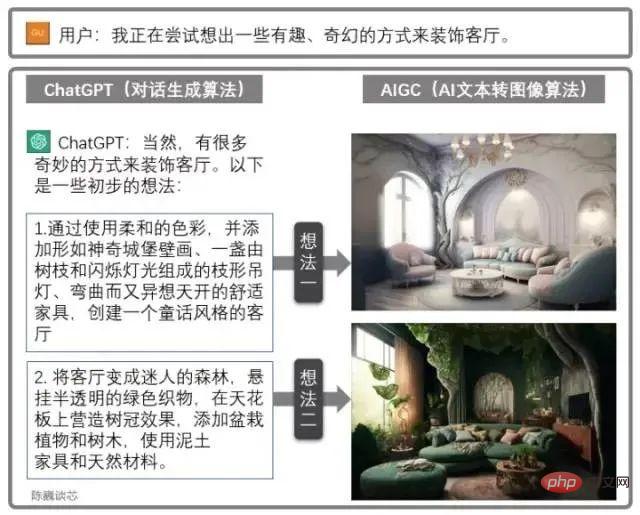
去年12月1日,OpenAI推出人工智能聊天原型ChatGPT,再次赚足眼球,为AI界引发了类似AIGC让艺术家失业的大讨论。ChatGPT是一种专注于对话生成的语言模型。它能够根据用户的文本输入,产生相应的智能回答。这个回答可以是简短的词语,也可以是长篇大论。其中GPT是GenerativePre-trainedTransformer(生成型预训练变换模型)的缩写。通过学习大量现成文本和对话集合(例如Wiki),ChatGPT能够像人类那样即时对话,流畅的回答各种问题。(当然回答速度比人还是
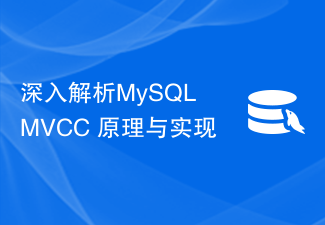
深入解析MySQLMVCC原理与实现MySQL是目前最流行的关系型数据库管理系统之一,它提供了多版本并发控制(MultiversionConcurrencyControl,MVCC)机制来支持高效并发处理。MVCC是一种在数据库中处理并发事务的方法,可以提供高并发和隔离性。本文将深入解析MySQLMVCC的原理与实现,并结合代码示例进行说明。一、M
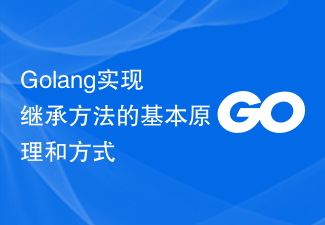
Golang继承方法的基本原理与实现方式在Golang中,继承是面向对象编程的重要特性之一。通过继承,我们可以使用父类的属性和方法,从而实现代码的复用和扩展性。本文将介绍Golang继承方法的基本原理和实现方式,并提供具体的代码示例。继承方法的基本原理在Golang中,继承是通过嵌入结构体的方式实现的。当一个结构体嵌入另一个结构体时,被嵌入的结构体就拥有了嵌
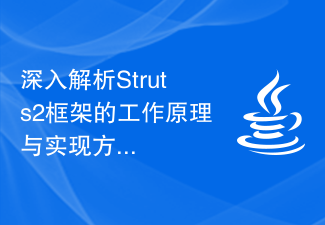
解读Struts2框架的原理及实现方式引言:Struts2作为一种流行的MVC(Model-View-Controller)框架,被广泛应用于JavaWeb开发中。它提供了一种将Web层与业务逻辑层分离的方式,并且具有灵活性和可扩展性。本文将介绍Struts2框架的基本原理和实现方式,同时提供一些具体的代码示例来帮助读者更好地理解该框架。一、框架原理:St
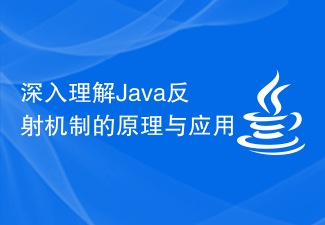
深入理解Java反射机制的原理与应用一、反射机制的概念与原理反射机制是指在程序运行时动态地获取类的信息、访问和操作类的成员(属性、方法、构造方法等)的能力。通过反射机制,我们可以在程序运行时动态地创建对象、调用方法和访问属性,而不需要在编译时知道类的具体信息。反射机制的核心是java.lang.reflect包中的类和接口。其中,Class类代表一个类的字节
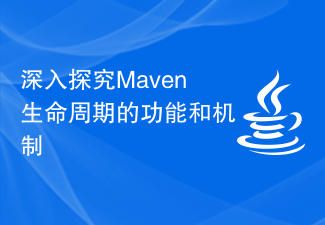
深入理解Maven生命周期的作用与原理Maven是一款非常流行的项目管理工具,它使用一种灵活的构建模型来管理项目的构建、测试和部署等任务。Maven的核心概念之一就是生命周期(Lifecycle),它定义了一系列阶段(Phase)和每个阶段的目标(Goal),帮助开发人员和构建工具按照预定的顺序执行相关操作。Maven的生命周期主要分为三套:Clean生命周
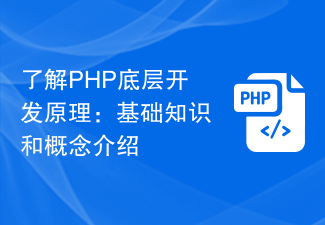
了解PHP底层开发原理:基础知识和概念介绍作为一名PHP开发者,了解PHP底层开发原理是非常重要的。正因为如此,本文将介绍PHP底层开发的基础知识和概念,帮助读者更好地理解和应用PHP。一、什么是PHP?PHP(全称:HypertextPreprocessor)是一门开源的脚本语言,主要用于Web开发。它可以嵌入到HTML文档中,通过服务器解释执行,并生成
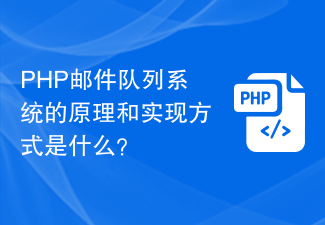
PHP邮件队列系统的原理和实现方式是什么?随着互联网的发展,电子邮件已经成为人们日常生活和工作中必不可少的通信方式之一。然而,随着业务的增长和用户数量的增加,直接发送电子邮件可能会导致服务器性能下降、邮件发送失败等问题。为了解决这个问题,可以使用邮件队列系统来通过串行队列的方式发送和管理电子邮件。邮件队列系统的实现原理如下:邮件入队列当需要发送邮件时,不再直


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
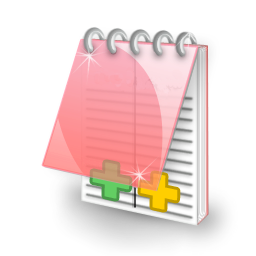
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
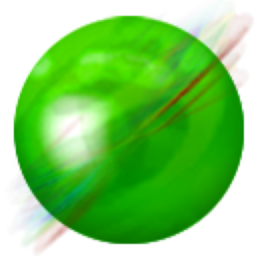
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
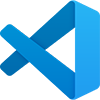
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
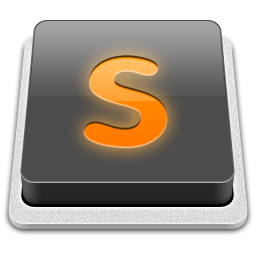
SublimeText3 Mac version
God-level code editing software (SublimeText3)
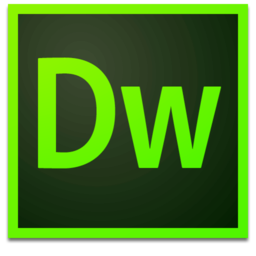
Dreamweaver Mac version
Visual web development tools