Go language is an open source programming language that is efficient and concise. One of its data structures is map. In the Go language, map is a "key-value pair" data structure that can be used to store a series of unordered key-value pairs. This article will introduce in detail the basic use of map in Go language and provide specific code examples.
1. Create a map
In the Go language, you can use the built-in make function to create an empty map. The syntax is as follows:
m := make(map[string]int) // 创建一个键为字符串,值为整数的map
2. Add key-value pairs
To add key-value pairs to the map, you can use map[key] = value. The example is as follows:
m := make(map[string]int) m["apple"] = 5 m["banana"] = 3
3. Get the value
Get it by key For the corresponding value, you can use map[key]. The example is as follows:
fmt.Println(m["apple"]) // 输出:5
4. Determine whether the key exists
When getting the value, sometimes you need to determine whether the key exists. You can Use the "comma ok" syntax, the example is as follows:
value, ok := m["orange"] if ok { fmt.Println("orange的值为:", value) } else { fmt.Println("orange不存在") }
5. Delete the key-value pair
To delete the key-value pair in the map, you can use the delete function, the example is as follows:
delete(m, "banana")
6. Map traversal
Use the for loop and range keyword to traverse the key-value pairs in the map. The example is as follows:
for key, value := range m { fmt.Println(key, ":", value) }
7. The length of the map
You can use the len function to get the number of key-value pairs in the map. The example is as follows:
fmt.Println("map的长度为:", len(m))
Through the above code examples, we can clearly understand how to use the map data structure in the Go language. The flexibility and efficiency of map make it one of the commonly used data structures in the Go language. Hope this article is helpful to beginners.
The above is the detailed content of Basic map usage in Go language. For more information, please follow other related articles on the PHP Chinese website!
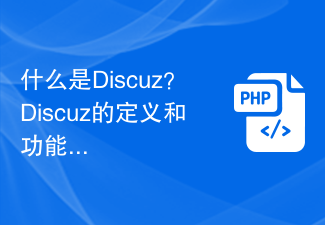
《探索Discuz:定义、功能及代码示例》随着互联网的迅猛发展,社区论坛已经成为人们获取信息、交流观点的重要平台。在众多的社区论坛系统中,Discuz作为国内较为知名的一种开源论坛软件,备受广大网站开发者和管理员的青睐。那么,什么是Discuz?它又有哪些功能,能为我们的网站提供怎样的帮助呢?本文将对Discuz进行详细介绍,并附上具体的代码示例,帮助读者更
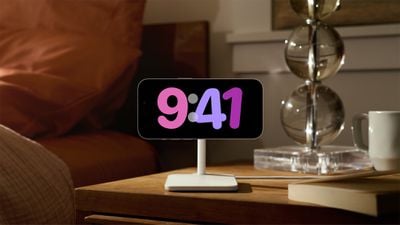
待机是一种锁定屏幕模式,当iPhone插入充电器并以水平(或横向)方向定位时激活。它由三个不同的屏幕组成,其中一个是全屏时间显示。继续阅读以了解如何更改时钟的样式。StandBy的第三个屏幕显示各种主题的时间和日期,您可以垂直滑动。某些主题还会显示其他信息,例如温度或下一个闹钟。如果您按住任何时钟,则可以在不同的主题之间切换,包括数字、模拟、世界、太阳能和浮动。Float以可自定义的颜色以大气泡数字显示时间,Solar具有更多标准字体,具有不同颜色的太阳耀斑设计,而World则通过突出显示世界地
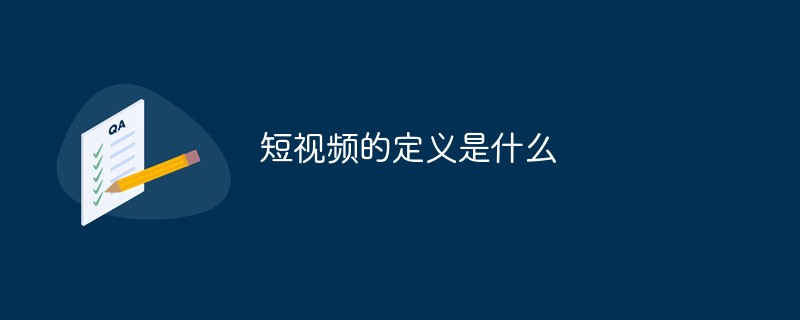
短视频的定义是指在各种新媒体平台上播放的、适合在移动状态和短时休闲状态下观看的、高频推送的视频内容,一般是在互联网新媒体上传播的时长在5分钟以内的视频;内容融合了技能分享、幽默搞怪、时尚潮流、社会热点、街头采访、公益教育、广告创意、商业定制等主题。短视频有着生产流程简单、制作门槛低、参与性强等特点。
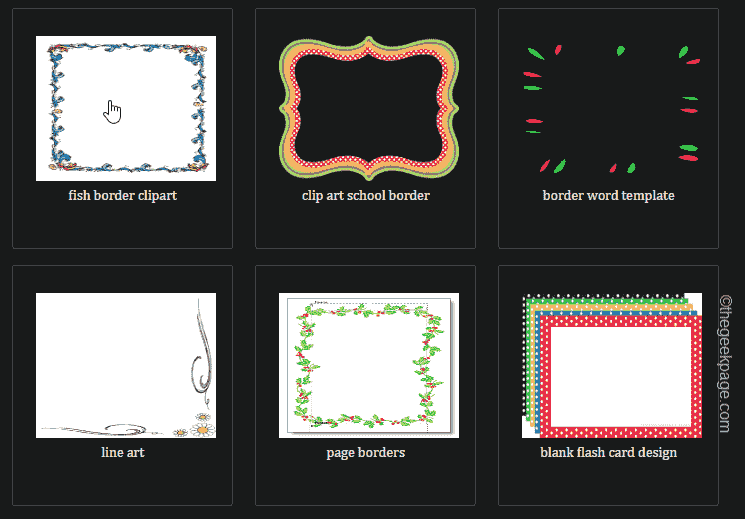
想让你的学校项目的头版看起来令人兴奋吗?没有什么比工作簿首页上的漂亮、优雅的边框更能使其从其他提交中脱颖而出了。但是,MicrosoftWord中的标准单行边框已经变得非常明显和无聊。因此,我们展示了在MicrosoftWord文档中创建和使用自定义边框的步骤。如何在MicrosoftWord中制作自定义边框创建自定义边框非常容易。但是,您将需要一个边界。第1步–下载自定义边框互联网上有大量的免费边界。我们已经下载了一个这样的边框。第1步–在Internet上搜索自定义边框。或者,您可以转到剪贴
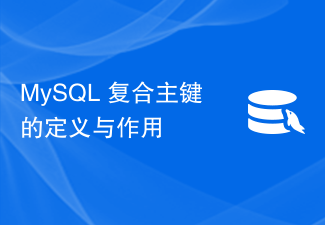
MySQL中的复合主键是指表中由多个字段组合而成的主键,用来唯一标识每条记录。与单一主键不同的是,复合主键由多个字段的值组合在一起形成。在创建表的时候,可以通过指定多个字段为主键来定义复合主键。为了演示复合主键的定义与作用,我们先创建一个名为users的表,其中包含了id、username和email这三个字段,其中id是自增主键,user
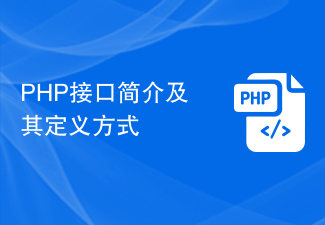
PHP接口简介及其定义方式PHP是一种广泛应用于Web开发的开源脚本语言,具有灵活、简单、强大等特点。在PHP中,接口(interface)是一种定义多个类之间公共方法的工具,实现了多态性,让代码更加灵活和可重用。本文将介绍PHP接口的概念及其定义方式,同时提供具体的代码示例展示其用法。1.PHP接口概念接口在面向对象编程中扮演着重要的角色,定义了类应
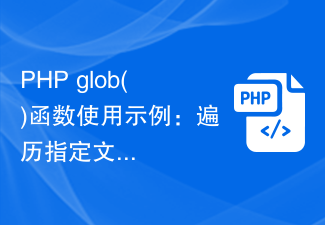
PHPglob()函数使用示例:遍历指定文件夹中的所有文件在PHP开发中,经常需要遍历指定文件夹中的所有文件,以实现文件批量操作或读取。PHP的glob()函数正是用来实现这种需求的。glob()函数可以通过指定一个通配符匹配模式,来获取指定文件夹中符合条件的所有文件的路径信息。在这篇文章中,我们将会演示如何使用glob()函数来遍历指定文件夹中的所有文件
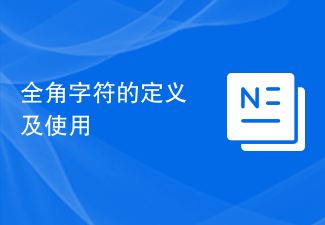
什么是全角字符?在计算机编码系统中,全角字符是一种占用两个标准字符位置的字符编码方式。相对应的,占用一个标准字符位置的字符编码方式被称为半角字符。全角字符通常用于中文、日文、韩文等亚洲文字的输入、显示和打印。在中文输入法和文字编辑中,全角字符与半角字符的使用场景是有所区别的。全角字符的使用中文输入法:在中文输入法中,通常全角字符用于输入中文字符,例如汉字、标


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
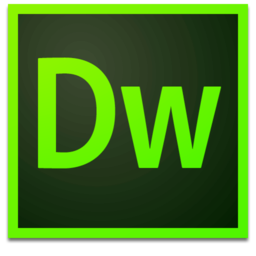
Dreamweaver Mac version
Visual web development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
